Map Interface in Java
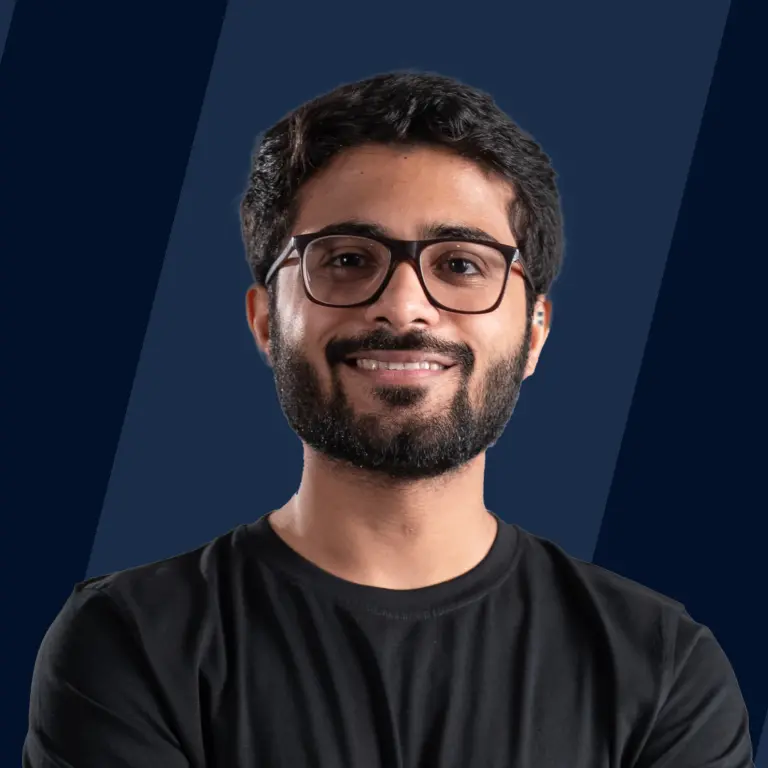
Overview
The map interface in java of the Java collections framework maps unique keys to the values. It is present in java.util package and store the data in key, value pairs where a key is an object that you use to later access the value.
Introduction to Map Interface in Java
Java's map interface has often been misunderstood as a subtype of the Collections interface. It behaves a little bit differently from the other collection types. The maps interface in java is a collection that links a key with value pairs.
- Each entry in the map store the data in a key and its corresponding value.
- Map interface contains only unique keys and does not allow any duplicate keys.
- The map interface in Java is a part of the java.util.map interface.
- A key is an object that you use to access the value later, it is associated with a single value.
- A map is used when you need to search, edit, or remove elements based on a key.
- A Map cannot be traversed, therefore you must use the keySet() or entrySet() method to convert it into a Set.
Hierarchy of the Map Interface in Java
Java supports the following two interfaces and three classes for Map implementation: Interface: Map and SortedMap. Classes: HashMap, LinkedHashMap, and TreeMap.
Below is a diagram showing the standard map interface hierarchy.
The HashMap class, which implements the Map interface, is extended by LinkedHashMap. The SortedMap interface, which extends the Map interface, is implemented by the TreeMap class.
Classes that implement Map
Class | Description |
---|---|
HashMap | Although HashMap implements Map, it doesn't maintain any kind of order. |
LinkedHashMap | The implementation of Map is LinkedHashMap. It inherits the class HashMap. The insertion order is maintained. |
TreeMap | both the map and the sortedMap interfaces are implemented. In TreeMap, the order is always maintained in ascending order. |
Interfaces that extend Map
Interface | Description |
---|---|
Map | Duplicate keys are not allowed in a map, although duplicate values are. |
SortedMap | In SortedMap, elements can be traversed in the sorted order of their keys. |
Creating Map Objects
Since Map is an interface, objects of the type map cannot be created. To create an object, we always need a class that extends this map. Also, because Generics were added in Java 1.5, it is now possible to restrict the types of objects that can be stored in the Map.
Syntax:
Characteristics of a Map Interface
- Each key can map to a maximum of one value, and a map cannot contain multiple keys. While some implementations, like the HashMap and LinkedHashMap, allow null keys and null values, others, like the TreeMap, do not.
- In the map interface in java, the order depends on the specific implementations. For eg, TreeMap and LinkedHashMap have a predictable order, whereas HashMap does not.
- Java provides two interfaces for implementing Map. They consist of three classes: HashMap, LinkedHashMap, and TreeMap as well as Map and a SortedMap.
- Set of keys, Set of Key-Value Mappings, and Collection of Values are the three collection views a map interface provides.
- Since the Map interface is not a subtype of the Collection interface. Thus, it differs from the other collection types in terms of features and behaviors.
When to Use the Map Interface in Java?
When someone has to retrieve and update elements based on keys or execute lookups by keys, the maps are used. Additionally, For key-value association mapping like dictionaries, maps are useful. The following are a few common scenarios:
- A map of cities and their zip codes.
- A map of error codes with their descriptions.
- A map of school classes and the students names. Each key (class) is associated with a list of values (student).
- A map of managers and employees in a company.
Methods of Map Interface
Method | Description | Time Complexity |
---|---|---|
put(Key, Value) | In this map, the given value and key are associated together using this method. | O(1) |
get(Key) | The object containing the value associated with the key is returned by this method. | O(1) |
containsKey(Key) | If a key matching the key exists in the map, this method returns true; otherwise, it returns false. | O(1) |
containsValue(Value) | If a value matching the value exists in the map, this method returns true; otherwise, it returns false. | O(n) |
isEmpty() | When the map has no keys, this method returns true; when there are keys, it returns false. | O(1) |
clear() | This method is used to clear all the elements in a map | O(n) |
remove(Key) | You can use it to remove an entry for the given key. | O(1) |
remove(key, value) | The given values and their corresponding specified keys are removed from the map. | O(1) |
size() | The number of key/value pairs in the map is returned by this method. | O(1) |
equals(Object) | The given Object and the Map are compared using this method. | O(n) |
entrySet() | It returns the Set view, which includes each key and value. | O(n) |
hashCode() | It returns the Map's hash code value. | O(1) |
keySet() | It returns the Set view with all the keys contained in it. | O(n) |
putIfAbsent(key, value) | it only inserts the specified value and key into the map when a value and key are not already present. | O(1) |
putAll(Map) | It is used to put the specified map into the map. | O(n) |
replace(key, value) | For a given key, it swaps out the given value. | O(1) |
replace( key, oldValue, newValue) | For a given key, it swaps out the old value with the new one. | O(1) |
values() | The values contained in the map are returned as a collection view. | O(n) |
Operations Using Map Interface and HashMap Class
Let's now explore a few commonly performed operations on a Map utilizing the popular HashMap class. Additionally, since Generics were added to Java 1.5, it is now possible to restrict the types of objects that can be stored in the map interface in java.
1. Adding Elements
The put() method is used to add new elements to the map. However, the hashmap does not keep track of the insertion order. Internally, a unique hash is created for each element, and the elements are indexed based on this hash to increase efficiency. The time complexity for adding the element is O(1).
Output:
Explanation:
At first, we initialized two maps that take a key as an integer and a string as a value. After that, we inserted some language names associated with an integer value in both maps, and At last, we printed the map as shown in the output above.
2. Changing Element
If we want to update an element after adding it, we can do so by adding it once more using the put() method. The values of the keys can be modified by simply inputting the updated value for the key that needs to be changed because the elements in the map are indexed using the keys. The time complexity for changing the element is O(1).
Output:
Explanation:
At first, we initialized a map that takes a key as an integer and a string as a value. Since the elements in the map are indexed using the keys, we modified it by simply putting the updated value for the key that needs to be changed.
3. Removing Elements
The remove() method can be used to remove an element from the Map. If there is a mapping for a key in the map, it is removed using this method, which accepts the key value. The time complexity for removing the element is O(1).
Output:
Explanation:
At first, we initialized a map that takes a key as an integer and a string as a value. Since the elements in the map are indexed using the keys, we deleted the element by simply passing the key to the remove() method.
4. Iterating through the Map
The Map can be iterated through in a number of ways. The most well-known method is to retrieve the keys using a for-each loop. The getValue() method is used to determine the key's value. Since it is iterating through all the elements, Hence the time complexity becomes O(n).
Output:
Explanation:
At first, we initialized a map that takes a key as an integer and a string as a value. After that we simply used a for-each loop and entrySet() to retrieve all the elements, then we stored each key and value in variables 'key' and 'value'. At the last, In each iteration, we printed the variables.
Implementing Map Interface in Java
The following images show classes that implement the Map interface, and the following description follows:
1. Implementing Map in Java Using the HashMap Class
The HashMap class provides the basic implementation of the map. It uses a hashing method to access the values from the keys it stores the elements as a key: value pair.
Output:
Explanation:
At first, we initialized a map using the HashMap Class that takes a key as a string and an integer as a value. After that we simply used a for-each loop and entrySet() method to retrieve all the elements, then In each iteration, we printed the key and values using the getKey() and getValue() methods.
2. Implementing Map in Java Using the LinkedHashMap Class
The LinkedHashMap class is almost similar to the HashMap. The only difference is it maintains order. The order in which the elements were added can be maintained, which the HashMap class was unable to do.
Output:
Explanation:
At first, we initialized a map using the LinkedHashMap Class that takes a key as a string and an integer as a value. After that we simply used a for-each loop and entrySet() method to retrieve all the elements, then In each iteration, we printed the key and values using the getKey() and getValue() methods.
3. Implementing Map Interface in Java Using the TreeMap Class
Since it provides a fast way to sort the map, it differs from HashMap and LinkedHashMap. We can sort the map using either the keys or the constructor used. The map is sorted with respect to the natural ordering of its keys.
Output:
Explanation:
At first, we initialized a map using the TreeMap Class that takes a key as a string and an integer as a value. After that we simply used a for-each loop and entrySet() method to retrieve all the elements, then In each iteration, we printed the key and values using the getKey() and getValue() methods.
Conclusion
- Map interface in java of the Java collections framework maps unique keys to the values.
- Map is present in java.util package and store the data in key, value pairs where a key is an object that you use to later access the value.
- A Map cannot be traversed, therefore you must use the keySet() or entrySet() method to convert it into a Set.
- Java supports the following two interfaces and three classes for Map implementation Map, SortedMap, HashMap, LinkedHashMap, and TreeMap.
- Since the Map interface is not a subtype of the Collection interface. Thus, it differs from the other collection types in terms of features and behaviors.