matches() in Java
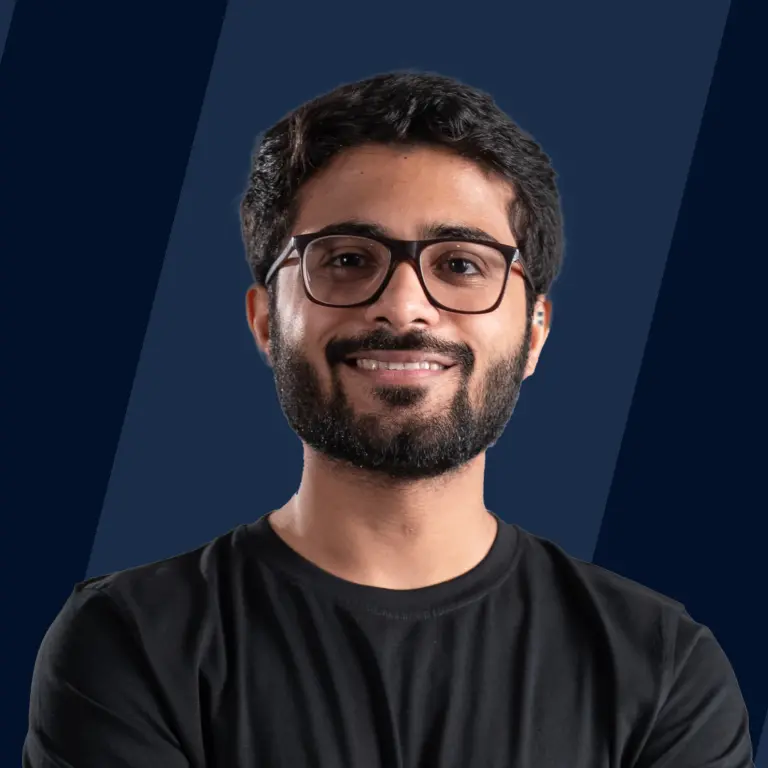
Overview
The matches() in java is the method of String class that checks whether a string matches a given regular expression or not. A regular expression or regex expression is a string pattern mainly used for searching or matching operations.
Syntax of matches() in Java
The signature of matches() is defined as:
The following defines how the method is written i.e. the syntax :
So as we can see, the method is called on the given string and matches with the regex statement.
Parameters of matches() in Java
matches() method takes a single parameter.
- String regex: The regular expression to which the string is to be matched.
Return Values of matches() in Java
Return Type: boolean
It returns:
- true: If the string matches the given regular expression.
- false: If the string does not match the given regular expression.
Exceptions for matches() in Java
The matches() throws the following exceptions:
-
NullPointerException: This exception is thrown in case null is passed as a parameter to the method.
-
PatternSyntaxException - This exception is thrown when the regular expression passed to the function is invalid.
Examples for both exceptions are discussed in the "More examples" section.
Example of matches() in Java
The following example will help you understand the implementation of the method.
Output:
Explanation: In the above program, we have defined a regex expression for a four-letter word that starts with J and ends with "a".Since the string matches the regex, true is returned.
More about matches() in Java
- An invocation of matches() method of the form str.matches(regex) shall yield the same result as of the expression Pattern.matches(regex, str). This method is widely used in URL matching and search operations in various text editors.
In order to implement this method, a little knowledge about regex expressions shall be helpful. Mostly these expressions are used for the "Find and Replace" kind of operations. The following table shall help you understand how a regular expression is written:
Syntax | Description |
---|---|
. | Matches any one character |
^ | Anchor; matches from the start of a string Anchor |
$ | Anchor; matches at the end of a string |
\ | Escape character |
* | Matches zero or more repetitions of the previous character |
+ | Matches one or more repetitions of the previous character |
? | Matches zero or one repetition of the previous character |
{n} | Quantifier; matches n repetitions of the previous character |
{n,x} | Quantifier; matches from n to x repetitions of the previous character |
[] | Character group; e.g. [AGCT] will match the characters AGCT |
[^ ] | Negated character group e.g. [^AGCT] will match any characters not in this group |
() | Matches the pattern specified in the parentheses exactly |
For example:
In the example section, we defined a regex expression for matching "Java" like: "^J..a$"
So in the pattern, "^" operator indicates that string to be matched should start with "J" and "$" indicates that string to be matched should end with "a". This way, we can define our own regex expressions.
Methods similar to matches() in Java
There are two other variants of this method. Let us learn more about the same.
String regionMatches():
This method is used to check if two string subregions are equal or not.
Syntax:
Following shows how the method is written:
- Parameters: regionMatches() takes four parameters.
- str_offset: It is the starting offset of the subregion in the case of this string
- other: It defines the string argument
- other_offset: This parameter defines the starting offset of the subregion in the case of the string argument
- len: It defines the number of characters that are to be compared
- Return Values: The return type of this method is Boolean. It returns :
- True: If the subregions(as specified using offset and length in params) of this string and string argument match.
- False: If the subregions(as specified using offset and length in params) of this string and string argument do not match.
Let us consider an example to understand better.
Output
Explanation
In the above program, We have two strings str 1 and str 2. Subregion of str 1 starting from index 11 is matched with the subregion of str 2 starting from 0, for a length of 5 characters(i.e., "learn" in both cases). Both the subregions match, hence true is returned in the output.
Note: The matching with regionMatches is case-sensitive.
String regionMatches() With ignoreCase
This variant can be considered as a modification of the regionMatches() method. It is useful while performing case-insensitive matching. ignoreCase parameter is present, in addition to the parameters of plain regionMatches(). This parameter basically decides whether case-insensitive matching is to be performed or not. A true value indicates case insensitive search. The return type of the method is, again boolean.
- Parameters: regionMatches() takes four parameters.
- str_offset: It is the starting offset of the subregion for this string
- other: It defines the string argument
- other_offset: This parameter defines the starting offset of the subregion for string argument
- len: It defines the number of characters that are to be compared
- ignoreCase: if true, the case is ignored while matching characters
- Return Values
return type of this method is Boolean. It returns : 1. True: If the subregions(as specified using offset and length in params) of this string and string argument match. 2. False : If the subregions(as specified using offset and length in params) of this string and string argument do not match.
Let us look at the implementation below:
Output:
Explanation: So as you see, the cases of subregions of str 1 and str2 do not match. When a true value is assigned to ignoreCase, case insensitive matching takes place, and true is returned i.e, subregions match. Contrary to this, on being assigned false, the method returns false as the case of the subregion is taken into consideration.
More Examples of matches() in Java
Example 1: Using Java matches()
Let us consider a situation where you have to find all the strings that end with a particular word. matches() method can be used in the case, we can define a regex expression/pattern for such strings.
Output:
Explanation: So as you can see, we have defined a regex pattern to check all the strings that end with "Java". String Str1 matches the regex, true is returned. String Str2 does not match the regex; hence false is returned.
Example 2: Check for Numbers
Output:
Explanation: In the above program, we have defined a regex expression for number matching. The case where the string to be matched has all numbers returns the true output. However, when alphabets are added along with numbers, it doesn't match the regex expression hence false is returned.
Example 3: NullPointerException
Output:
Explanation: So in the above example, matches() is called on string "Java", null is passed as the parameter; hence exception is encountered.
Example 4: PatternSyntaxException
Output:
Explanation: In the above program, the regex expression is not defined in a correct manner. The syntax is invalid; hence exception is thrown.
Conclusion
- matches() method checks whether a string matches a given regular expression or not.
- It has a boolean return type.
- The other variant of the method is regionMatches(), which is used to check if two string regions are equal or not.