What is the Difference Between Static and Dynamic Memory Allocation?
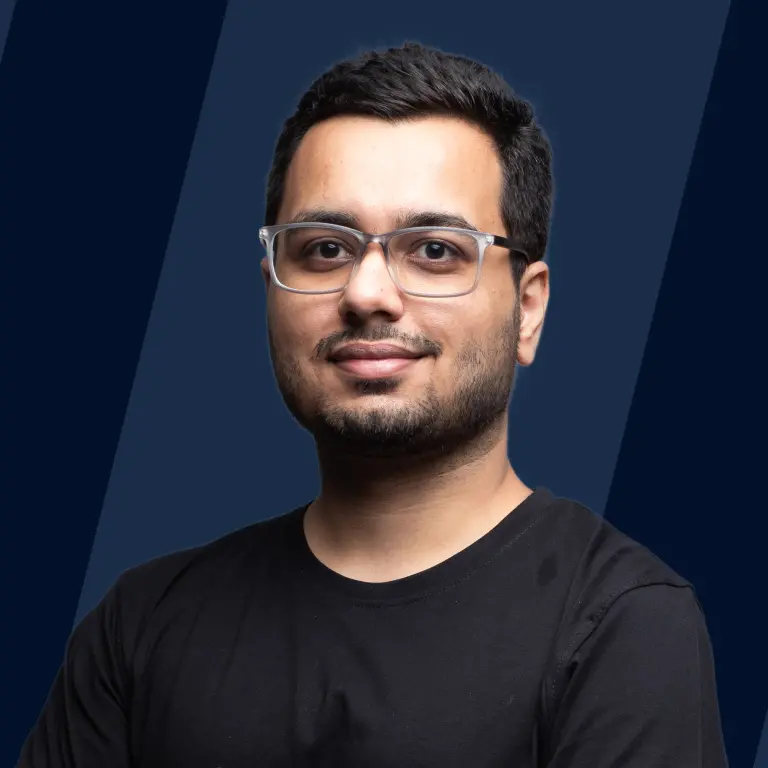
Introduction
Memory is the place where data fetched from the code is stored. When a variable is created in C++, a Memory address is assigned. The term allocation is used to indicate variable has been stored. Memory is divided into two parts known as stack Memory and heap Memory. Stack Memory is associated with static Memory allocation and is smaller than heap Memory. It is also known as compile time allocation. Heap Memory is associated with dynamic Memory allocation and is larger than stack Memory. It is also known as run-time allocation.
Static Memory Allocation in C++
Static Memory allocation in C++ allocates size and location to a fixed variable. Static Memory allocation is done before the program is executed. In other words, memory is allocated during compile time. Hence, it is known as compile time allocation. The size and the location of the variable are fixed throughout the program.
Example of Static Memory Allocation in C++
Let us look at an example of allocating static Memory in C++.
In the above code, we have declared three variables- integer p, character c, and an array of integers arr.
In the static Memory allocation in C++, variables will be allocated a fixed location and size. One important aspect has to be considered, that actual physical memory is allocated only at the runtime. During compile time, actual physical Memory is not allocated to the variables.
Some other points that need to be considered about static Memory allocation are-
- Static memory allocation in C++ is less flexible than dynamic Memory allocation in C++.
- Static memory allocation is marginally faster than dynamic Memory allocation in C++.
- The compiler's role is to allocate and deallocate Memory.
Dynamic Memory Allocation in C++
In dynamic Memory allocation in C++, the Memory is defined during the execution of the program. Heap Memory is associated with dynamic Memory allocation and is larger than stack Memory. It is also known as run-time allocation.
Pointers play a crucial role in dynamic Memory allocation in C++. As we have seen in static Memory allocation in C++, the Memory is allocated and deallocated automatically. Whereas in dynamic Memor allocation, the programmer must do the memory allocation and de-allocation manually. The 'new' keyword is used to allocate Memory, and the 'delete' keyword is used to deallocate Memory. While creating a new variable, if Memory is available, then Memory is initialized, and the address of the space is returned to the pointer variable.
Different factors need to be considered-
- Memory reuse is possible in dynamic Memory allocation.
- Memory leak is possible. It needs to be taken care of by the programmer.
Allocate memory in C++:
Syntax-
The pointer is of pointer data_type. The data_type is of the type float, int string, etc.
Example- 1)
De-allocate memory in C++:
For deallocating Memory in C++, we use the delete operator.
Syntax-
Example-
In C++, deallocating Memory plays a very vital role. When the variable is not in use, the programmer must deallocate the Memory. Otherwise, the problem of Memory leak occurs. Memory leak means when a programmer allocates memory but forgets to deallocate it. Since the memory is limited in a system, we need to free it after its utilization. So to deallocate memory, we use the delete keyword, and we use it when the variable is no longer needed for use.
Example of Dynamic Memory Allocation in C++
We will understand how to allocate memory dynamically in C++.
In the code, we have created a variable n. n is statically allocated memory, and during execution, the new keyword is used. It returns the address of the memory where it has been allocated memory. We have created an array dynamically using the new keyword. Here the work of memory allocation is done. After taking input from the user, we store it in the array. Since we don't need the array, we deallocate the associated memory. The delete keyword is used. This is how dynamic memory allocation works in C++.
Differences between Static and Dynamic Memory Allocation
Static memory allocation | Dynamic memory allocation |
---|---|
Static memory allocation in C++ allocates size and location to a fixed variable. | Dynamic memory allocation in C++ allocates size and location to a dynamic variable. |
Static memory allocation is done before the program is executed. | In dynamic memory allocation in C++, the memory is defined during the execution of the program. |
It is known as compile time allocation. | It is known as run-time allocation. |
It is less flexible. | It is more flexible. |
Stack memory is used. | Heap memory is used. |
Allocation and de-allocation are done by the compiler. | Allocation and de-allocation are done by the programmer using new and delete keywords. |
Conclusion
- Memory is divided into two parts, known as stack memory and heap memory. Stack memory is associated with static memory allocation and is smaller than heap memory. Heap memory is associated with dynamic memory allocation and is larger than stack memory.
- Static memory allocation in C++ allocates size and location to a fixed variable.
- Dynamic memory allocation in C++ allocates size and location to a dynamic variable.
- Static memory allocation in C++ is done before the program is executed, and dynamic memory allocation in C++ is done during run-time.
- The compiler does allocation and de-allocation in static memory allocation, and the programmer does it in dynamic memory allocation.