What is Memory Leak in C?
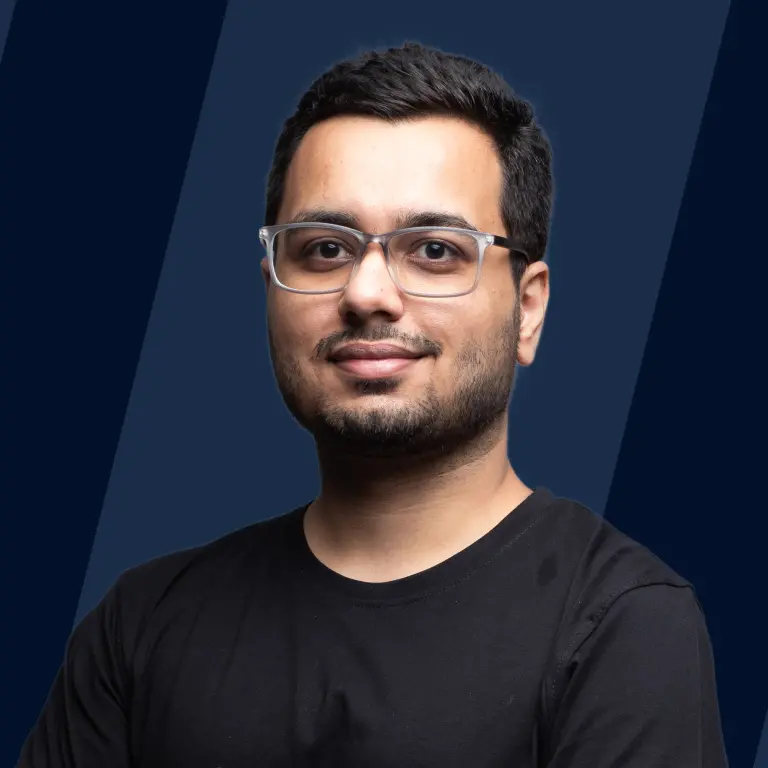
A memory leak in C is a specific kind of resource leak that happens when a computer software or program poorly handles memory allocations and fails to free up the memory that is no longer required. A memory leak can also occur when a variable/object is kept in memory but is not accessible to the program that is running.
As there is limited memory available in each system, memory leaks can have an effect on lowering the system's performance. In the worst-case scenario, too much of the available memory may be allocated, causing the system or device to malfunction entirely or partially, the programs may crash, or the system may significantly slow down.
What can Cause a Memory Leak in C?
A memory leak in C typically happens when the pointer loses its initial allocated address due to which the pointed memory block cannot be accessed or cannot de-allocated, and this becomes the reason for the memory leaks. Let's look at a few other examples where memory leaks can occur:
- Assigning a different address value to the pointer variable before de-allocating the memory block already pointed by the pointer.
- If a pointer variable goes out of scope, it may lead to a memory leak.
- Not deallocating the memory before the program execution finishes.
- If an error occurred during the de-allocation of the memory block.
Visit the following link to know more about dynamic allocation and de-allocation of memory blocks using malloc() and calloc() in C Language, Dynamic Memory Allocation in C.
How to Avoid Memory Leaks in C?
Various tools are available to find memory leaks in a C program, but by following the basic programming best practices, we can prevent memory leaks. Let's look at some tips to avoid memory leaks in C:
1. There should be a free() function statement associated with every malloc() or calloc() function statement. See the illustration below:
Writing the free() statement immediately after the malloc() or calloc() function is a good practice. It helps to avoid the scenario when a programmer forgets to add a free() statement.
2. Avoid modifying the original pointer, use a temporary pointer.
Working with a temporary pointer is a good practice since the original pointer keeps the address of the allocated memory, and it can get accidentally changed. So, if we work with a temp pointer, we can preserve the original pointer value and re-collect the address value to de-allocate the memory for avoiding any memory leaks.
Examples of Memory Leaks in C
1. Let's see an example where we assign a NULL value to a pointer variable to demonstrate memory leaks in a C Program:
C Program:
Output:
Explanation:
In the above C Program, we have allocated the space for an integer memory block using the malloc() function in the ptr pointer. When we assign NULL to the ptr pointer, it changes the pointer value but doesn't de-allocate the memory block that it was previously pointing to. So, it will lead to a memory leak.
To avoid this situation, we have to uncomment the free(ptr); statement mentioned just above the ptr = NULL; statement.
2. Let's look at an example, where a pointer variable goes out of scope, it leads to a memory leak in C Program
C Program:
Output:
Explanation: In the above C Program, we have allocated an integer memory block to the sum pointer variable in a local scope (inner block of code) inside the main() function. We have assigned the addition of a and b to the allocated memory block using the sum pointer, but when the block scope finishes the sum pointer gets destroyed and the memory block remains allocated. So, it will lead to a memory leak.
To avoid this situation, we have to uncomment the free(sum); statement mentioned just above the sum = NULL; statement in the inner block of code.
Learn about Memory Layout in C
Visit the following link to know about components of Memory Layout in C Language like instructions, global variable space, stack, heap, and more: Memory Layout in C.
Conclusion
- A memory leak in C happens when a computer program poorly handles memory allocations and fails to free up the memory that is no longer required.
- Memory leaks can have an effect on lowering the system's performance.
- Writing the free() statement immediately after the malloc() or calloc() function is a good practice to avoid memory leaks.
- We should assign NULL to the pointer variable after using the free() statement to avoid dangling pointers.