What is Memory Management in Java?
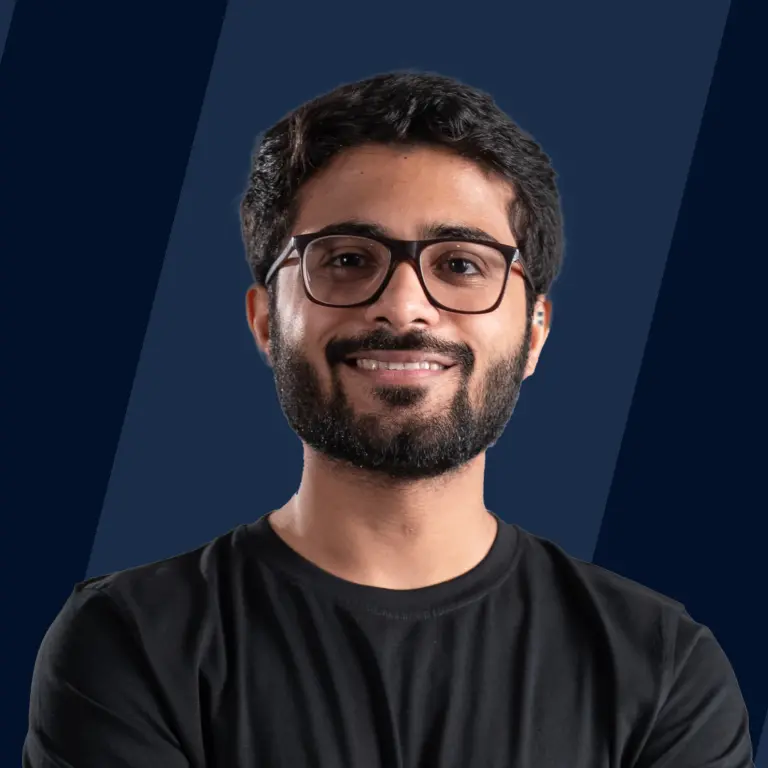
Memory management in Java refers to the process of allocating and freeing up space for objects. Java automatically manages memory. The "garbage collector" is an autonomous memory management technique used by Java. Consequently, implementing memory management logic in our program is not necessary.
Why Learning about Memory Management is Important in Java?
Everyone knows that Java maintains memory on its own and doesn't require a programmer's explicit assistance. Garbage collection guarantees that memory may be released when not needed and that unused space is cleaned up. So what is a programmer's job and why is it important for them to understand Java Memory Management? Programmers don't have to worry about issues like object destruction thanks to the garbage collector. However, not everything is ensured by automatic waste pickup. If we don't understand how memory management functions, we frequently find ourselves dealing with JVM-managed objects (Java Virtual Machine). Some items cannot be collected automatically by garbage disposal.
Thus, understanding memory management is crucial because it will help programmers create high-performance systems that do not crash or, if they do, know how to troubleshoot or fix them.
Java Memory Management is divided into two major part :
- JVM Memory Structure
- Working of the Garbage Collector
JVM Memory Structure
Method Area :
-
The heap memory, which is shared by all threads, includes a section called Method Area. When the JVM starts, it creates.
-
The name of the superclass, the name of the interface, and the constructors are all stored there.
-
It includes elements specific to each class, including fields, constant pools, method local data, method codes, Constructor codes, etc., that are used to initialize objects and interfaces.
-
The Java Virtual Machine throws an OutOfMemoryError if the memory in the method area cannot be made accessible to satisfy an allocation request.
-
It could have a set size or fluctuate. It might not have a continuous memory.
Heap Area :
The objects that are produced while a Java program is running are kept in heap memory.
The reference to newly formed objects is kept in stack memory. Dynamic memory allocation is followed by heap (memory is allocated during execution or runtime).
When compared to stack, heap memory is larger in size.
The garbage collector automatically removes any unnecessary objects from the heap memory.
The heap's memory does not have to be contiguous.
JVM gives the user the option to initialize or change the heap size as needed.
The Java Virtual Machine throws an OutOfMemoryError whenever a calculation needs more heap than the automatic storage management mechanism can provide.
An object is given a place in the heap when a new keyword is used, but a reference to the same object already existing on the stack.
Example :
Code Explanation :
The above code creates an object of the Person class. The object allocates to the heap, and the reference p allocates to the stack.
Heap is divided into the following parts :
- Young generation :
All new objects are assigned and aged in this location. When this is full, there is a small garbage collection. - Old generation :
Long-lasting items are kept in this location. A threshold for the object's age is specified when it is placed in the Young Generation, and once it is met, the object is transferred to the Old Generation. - Permanent generation :
This comprises JVM metadata for the application methods and runtime classes. - Survivor space
- Code Cache
Stack Area :
- When a thread starts, the stack area is generated. It might have a fixed size or a variable size.
- Each thread has its allocation of stack memory. Data and unfinished results are stored there.
- There are heap object references in it.
- Furthermore, it stores the value directly rather than a reference to an item from the heap.
- The term "scope" refers to the visibility of the variables that are kept in the stack.
- It is not necessary for stack memory to be continuous.
Native Method Stack :
Native method stacks, often known as C stacks, are not created in the Java programming language. Each thread receives a memory allotment when it is formed. Furthermore, it may have a fixed or dynamic nature.
Program Counter (PC) Registers :
A Program Counter (PC) register is linked to every thread. A native pointer or the return address is kept in the PC register. It also includes the location of the JVM instructions that are now being carried out.
Working of Garbage Collector
- The garbage collector is managed by JVM. When to do garbage collection is determined by JVM. Additionally, we can ask the JVM to activate the garbage collector. However, there is never an assurance that the JVM will adhere to the rules. If JVM detects that memory is running low, it starts the garbage collector. The JVM often responds quickly to requests from Java programs for the garbage collector. It doesn't guarantee that the recommendations are granted.
- Garbage collection is an expensive process since it pauses all other processes or threads. Although the customer finds this situation intolerable, it can be fixed by using numerous garbage collector-based methods. This algorithm application process, also known as garbage collector tuning, is crucial for enhancing program performance.
- The generational garbage collectors, which add an age field to the objects that are allotted a memory, offer a different solution. The time it takes to gather garbage grows as more and more things are generated and added to the garbage list. The objects are divided into groups and given an age based on how many clock cycles they have endured. This way the garbage collection work gets distributed.
Java Has Five Types of Garbage Collector Implementations :
-
Serial GC :
Given that it mostly relies on a single thread, this is the simplest GC implementation. Because of this, whenever this GC implementation executes, all application threads are frozen. Therefore, using it in multi-threaded applications like server environments is not a good idea. -
Parallel GC :
It is referred to as throughput collectors and serves as the JVM's default GC. It uses several threads, as opposed to Serial Garbage Collector, to manage memory space, but it also pauses other application threads while doing GC. -
Concurrent Mark Sweep (CMS) Collector :
Multiple garbage collector threads are used by the Concurrent Mark Sweep (CMS) garbage collector to collect garbage. It analyses the heap memory to identify instances that should be swept, then it marks such instances for eviction. It is made for programs that can afford to share processor resources with the garbage collector while they are operating and that want shorter trash collection pauses. The "CMS collector" employs more CPU than the parallel garbage collector to ensure faster application throughput. The "CMS garbage collector" is recommended over the parallel collection if we can assign additional CPU for better performance. -
G1 Garbage Collector :
The G1 (Garbage First) Garbage Collector is made for programs that use multi-processor computers with a lot of memory.It divides the heap memory into sections and performs parallel collection within each one. Just after reclaiming the memory, G1 simultaneously compacts the free heap space. However, in stop-the-world (STW) conditions, the CMS trash collector compacts the memory. The G1 collector will take the place of the CMS collector because it performs better.
-
Z garbage collector :
ZGC is appropriate for applications that demand low latency and/or employ a very large heap since it executes all expensive work concurrently without pausing the execution of application threads for longer than 10ms. "Oracle documentation" states that multi-terabyte heaps are supported. Oracle made ZGC available in Java 11. Cycles are completed by the Z garbage collector in its threads. It typically pauses the application for 1 milliseconds. The Parallel and G1 collectors have an average of about 200ms.
Conclusion
-
"Memory management" in Java refers to the process of allocating and freeing up space for objects.
-
Java Memory Management is divided into two major part :
- JVM Memory Structure
- Working of the Garbage Collector
-
The Java memory model explains how threads communicate with one another through memory in the Java programming language. The objects of the programs are stored in various locations in the JVM memory structure.
-
Process of freeing space in the heap for the allocation of new objects is known as "Garbage collection".
To learn more about Java Programming Click here