Merge Two Lists in Python
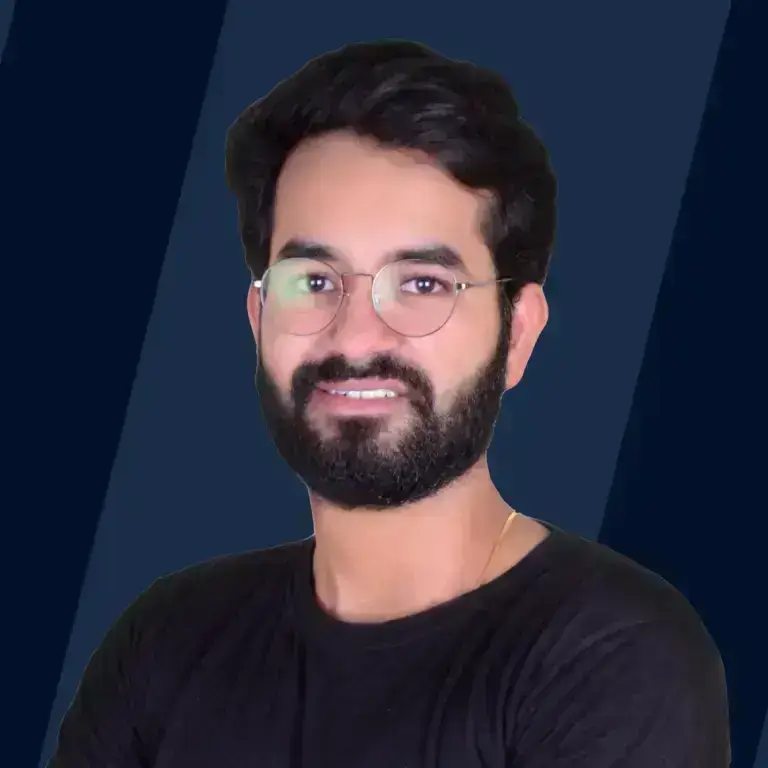
Overview
A list is a data structure in Python that contains a sequence of elements. We can have many lists in our code, which sometimes we may need to merge, join, or concatenate. Merging a list is very useful in many ways, and through this article, we will see the various ways we can merge two lists in Python.
Merging Two Lists in Python
Merging lists means adding or concatenating one list with another. In simple words, it means joining two lists. We can use various ways to join two lists in Python. Let us discuss them below:
1. Using append() function
One simple and popular way to merge(join) two lists in Python is using the in-built append() method of python. The append() method in python adds a single item to the existing list. It doesn't return a new list of items. Instead, it modifies the original list by adding the item to the end of the list.
Code:
Output:
Explanation:
- In the above code, we declare two lists, ls1 and ls2.
- We iterate over ls2 and append its elements to ls1 using our python's append method.
2. Using the '+' Operator
This is the simplest way to merge two lists in Python. '+' operator is a multipurpose operator, which we can use for arithmetic calculations and for merging purposes, strings, lists, etc.
Code:
Output:
Explanation:
- We defined our lists ls1 and ls2
- Then, we merged them using the '+' operator in Python.
- The use of + operator adds the whole of one list behind the other list.
3. Using List Comprehension
We can merge two lists in Python using list comprehension as well. List comprehension offers a shorter and crisper syntax when we want to create a new list based on the values of an existing list. It may also be called an alternative to the loop method.
Code:
Output:
Explanation:
- In the above example, first, this line is executed for n in (num1,num2), which will return [[1, 2, 3], [4, 5, 6]].
- After that, we pick one element at a time from the above lists. Hence we do for x in n.
- Finally, we get our desired result by storing the value of x in the list: [x for n in (num1,num2) for x in n]
4. Using the extend() method
The extend() method adds all the elements of an iterable (list, tuple, string, etc.) to the end of the list. It updates the original list itself. Hence its return type is None. It can be used to merge two lists in Python.
Code:
Output:
Explanation:
- We defined our lists and passed them to our extend method in python
- extend basically, extended the list2 to the end of list1. The size of the list increased by the length of both lists.
- Also, extend updated our ls1 directly.
5. Using iterable unpacking operator *
An asterisk * denotes iterable unpacking. The unpacking operator takes any iterable(like list, tuple, set, etc.) as a parameter. Then the iterable is expanded into a sequence of items included in the new tuple, list, or set at the site of the unpacking.
Any no. of lists can be concatenated and returned in a new list using this operator. Hence it can be used to merge two lists in Python.
Note: works only in Python 3.6+.
Code:
Output:
Explanation:
- The * first unpacks the contents of ls1, ls2, and ls3 and then creates a list from its contents.
- Then, all the items of our iterables will be added to our new list ls.
Python's simplicity and flexibility make it an ideal language to learn. Join our free Python certification course & witness your coding proficiency soar to new heights.
Conclusion
- Merging two lists in Python is a common task when working with data.
- There are several ways to merge two lists in Python, depending on the specific requirements of the task.
- One of the simplest ways to merge two lists is to use the "+" operator to concatenate them.
- Another approach is to use the "extend()" method to add the elements of one list to another.
- You can also use the "zip()" function to combine the elements of two lists into a list of tuples.
- If the lists contain duplicates, you can use the "set()" function to remove them before merging.
- In some cases, you may need to merge lists while preserving the order of elements. This can be done using the "sorted()" function or a custom sorting function.
- When merging large lists or working with memory-intensive data, it's important to consider the efficiency of the merging method.