Method Overriding in Python
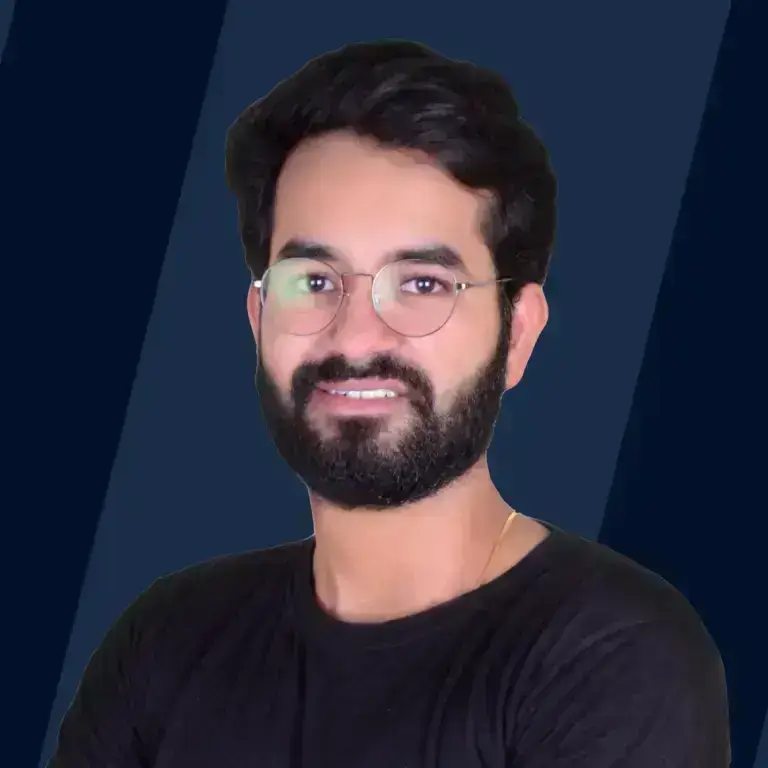
Abstract
In this tutorial, we will learn a very important aspect of object-oriented programming -- Method Overriding in Python. It is an essential part of the inheritance mechanism. Let us get a brief description of overriding first, so that we can know about it's super powers and how can it make our code super productive after that.
Introduction to Method Overriding in Python
Method Overriding in Python is an OOPs concept closely related to inheritance. When a child class method overrides(or, provides it's own implementation) the parent class method of the same name, parameters and return type, it is known as method overriding.
In this case, the child class's method is called the overriding method and the parent class's method is called the overriden method.
Method overriding is completely different from the concept of method overloading. Method overloading occurs when there are two functions with the same name but different parameters. And, method overloading is not directly supported in Python.
Parent class: The class being inherited is called the Parent or Superclass.
Child class : The class that inherits the properties and methods of the parent class is called the Child or Subclass.
Note:
Inheritance is the capability of one class to derive or inherit the properties from another class, hence promoting code reusability.
Key features of Method Overriding in Python
These are some of the key features and advantages of method overriding in Python --
- Method Overriding is derived from the concept of object oriented programming
- Method Overriding allows us to change the implementation of a function in the child class which is defined in the parent class.
- Method Overriding is a part of the inheritance mechanism
- Method Overriding avoids duplication of code
- Method Overriding also enhances the code adding some additional properties.
Prerequisites for method overriding
There are certain prerequisites for method overriding in Python. They're discussed below --
- Method overriding cannot be done within a class. So,we need to derive a child class from a parent class. Hence Inheritance is mandatory.
- The method must have the same name as in the parent class
- The method must have the same number of parameters as in the parent class.
Example of Method Overriding in Python:
So, in the above example we can see,
- no_of_sides is the overriden method in the Shape class, since the Square class method - no_of_sides() will be overriding it (adding it's own implementation).
- two_dimensional is inherited by the sub_class(hence inherited method)
- color is a new method added in the Square class.
- data1 & data2 are individual properties of the classes.
Let us code the above example and understand more deeply. Here we have our parent class Shape --
Let's create a child class - Square which will extend the class Shape:
In the child class - Square, we have overridden the method no_of_sides() adding our own implementation.
So now, when we create an object of the Square class and call the method no_of_sides() we will see, it will override the already exsisting version of the parent class.
OUTPUT:
Not only that, we can also override the data1 variable present in the parent class(Shape) using the Child Class(Square) --
OUTPUT:
Complete code:
OUTPUT:
Method Resolution Order in Python
Method resolution order in Python is the order in which a python program resolves or searches for a class in case of multiple inheritance.
Important points to be noted --
- It play's a crucial role for programs using multi-inheritance.
- In multi-inheritance a class is derived from more than one base class, hence inheriting all properties of the base class
- In this approach -> the method or attributes are first searched in the base class. If it is not present then the seraching moves a level up -- to the immediate parent class. And if again no luck, then the searching continues following the same search approach.
- It follows the depth-first search approach.
Now let us understand this through an example -- Diamond Inheritance:
Diamond Inheritance refers to an ambiguity that arises when two classes -- B and C inherit from a superclass A and class D inherits from both class B and C. Now, suppose there is a method “demo” which is an overridden method in one of Class B and Class C or both then the ambiguity arises which of the method “demo” Class D should inherit.
The problem is solved in a depth first search fashion.
Note:
Diamond Inheritance is a very good example of overriding in multiple inheritance in Python.
Let us take an example to understand this problem better --
Scenario 1:
Here we have a base class A, and B & C are the sub class, inheriting from A. Class D inherits from B & C, also getting the access to methods of class A. So, this is an example of a multi-level inheritance. Let us see the code:
In this code the order of execution will be : class D -> class B -> class C -> class A
So, the output will be:
Since, the class D will find the method demo() instantly in class B so will execute class B's method and will not search in other classes.
Scenario 2:
Suppose, we pass the parameters to D in some other order -- like class D(C,B)
Then MRO will resolve it in this order:
class D -> class C -> class B -> class A
So, since it finds the method in class C at the first chance, it executes the method in that class itself.
Output:
Scenario 3:
But, let us violate the structure as shown in the tree and pass in this order :
class D(A,B,C):
It is a clear violation, because D cannot start finding the method from A and eventually go to B then C; Then definitely, we will get the error:
Since, D cannot resolve the order, going straight to A and the coming to B & C. So here we get the TypeError. But we will not do the same if we pass the parameter in this fashion:
Then we will get the intended output:
So MRO is nothing, but the order in which a class searches for any method/attribute in it's parent classes in a DFS fashion. And, the order of class we pass as parameters to the class is very important, otherwise it will result in error if violated.
Overriding With Multiple and Multilevel Inheritances
Inheritance:
It is the capability of one class to derive or inherit the properties from another class. It promotes reusability of code.
Note:
Inheritance is transitive in nature, i.e. if any class B inherits from another class A, then all the subclasses of B would automatically inherit from class A.
Multiple Inheritance
When a class is derived from one or more parent(base) class, inheriting all it's properties, it is called the multiple inheritance. In the above image, D is heriting from multiple parents(B & C).
Overriding in Multiple Inheritance
If the child class provides it's own implementation to the methods/attributes while inheriting them from it's parent/parents then it is known as Overriding in Multiple Inheritance.
Example:
The Diamond Probelm we discussed above is a great example of Overriding in Multiple Inheritance.
Let us take one more example to further clarify our concept:
Here, when function call takes place, it goes in a depth first search fashion and provides the output.
Multi-level Inheritance:
When we have a child and grandchild relationship in our code where we have different levels relationship, it is known as Multi-level Inheritance. In the above image, class 3 is inheriting from class 2 which in turn is inheriting from class 1. So 3 is the Grandchildren, 2 is the Parent and 1 is the GrandParent.
Overriding in Multi-level Inheritance:
When we override the methods or attributes of the Parent class or GrandParent class through the child class(class 3 here), then it is known as Overriding in Multi-level Inheritance. In this example above, if class 3 provides it's own implementation for class 2 and class 1 then it is Overriding in Multi-level Inheritance.
Example:
Here the father inherits the method (home) from his father.
And the Son inherits "home" from his father's father(Grandfather).
Although, they provide separate implementation for their ages hence overriding this method.
OUTPUT:
Hence we see, the home() method is inherited but the age method is overriden by the class Son.
Conclusion
Here, we come to the end of the article. So, let us revise our understanding of method overriding in Python. We saw that Method Overriding in Python can be so much useful and how can it ease our burden of re-writing the same code. Also --
- Prerequisites for method overriding
- Example
- Python method resolution order
- Overriding with multiple and multilevel inheritances
- Some great examples of overriding in Python
Overall we can conclude that it is a very useful and beautiful concept as it allows us to extend the functionality of our parent classes and thus improves code reusability.