What is Multilevel Inheritance in C++?
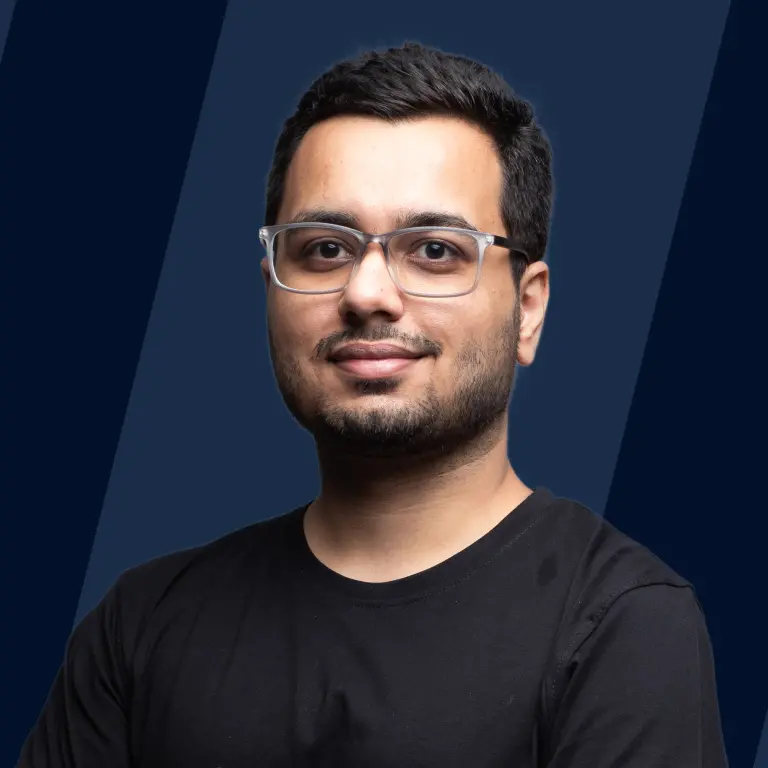
Overview
Inheritance is a mechanism in which one class acquires the property of another class. For example, a child inherits the traits of his/her parents. With inheritance, we can reuse the fields and methods of the existing class. Hence, inheritance facilitates Reusability and is an important concept of OOPs.
Introduction
Before going on to the main topic, let’s understand basic inheritance. The child class is derived from its parent class in the basic level inheritance. The parent class is called base class and the child class is called the derived class. All the properties of the parent class (public and protected properties) are inherited into the child class. We can say it is a father-son relationship.
In the case of multilevel inheritance, more than two classes are involved. I will try to explain multilevel inheritance in layman's terms. As everyone knows, the theory of evolution that Charles Darwin proposed. The theory says that organisms evolve over generations through the inheritance of physical or behavioral traits. It means humans' physical or behavioral traits are now inherited from their ancestors.
Similarly, in the case of multilevel inheritance, a derived class will inherit a base class, and the derived class will act as the base class for other classes. Consider n classes numbered from 1 to n, as shown in the following diagram.
We can see that class 1 is the parent of all the n-1 classes. Every class i (2 <= i <= n) is derived from the i-1th class. The ith class will not only inherit the properties of i-1th, but also it will inherit all the properties of classes from 1 to i-1. Hence, this is multilevel inheritance.
Syntax
The basic syntax of multilevel inheritance in C++ is as follows.
In the above pseudo-code, class Three inherits from class Two, whereas class Two inherits from Class One. Hence class One is the parent of the rest two classes.
Example of Multilevel Inheritance
Let us see an example of Multilevel Inheritance.
Code
Output
Explanation
In the above example, we have created three classes, i.e., Grandfather, Father, and Son. Now, we have created an object of the Son class, which inherits properties from the Father class. The Father class also inherits properties from the Grandfather class; therefore, the Son class will have properties of both the Father and Grandfather classes. With the created object of the Son class, we can call the methods of the Son, Father, and Grandfather classes.
How Does Multilevel Inheritance Work in C++?
Let us modify the above-taken example and will try to understand the working of multilevel inheritance in C++. What happens when we remove the Print method from Father and Son class?
Code
Output
Explanation
In the above code, we have a process in the Grandfather class, and the processes from the Father and Son classes are deleted. Now, when we create the object of the Son class and call the Print method, the Print method from the Grandfather class will be executed as there is no Print method in Father and Son class. The compiler first searches for the Print method in the Son class. There is no Print method in the Son class, so it continues its search in the Father class. Again compiler will fail to find the Print method. Finally, it will search for the Print method in the Grandfather class. As the compiler is successful in finding the Print method, it will execute the Print method. In this way, the execution of multilevel inheritance in C++ occurs.
Code
Output
Explanation
In the above code, we are creating an object of the Son class. Using the created object, we are calling the Print function. But this time, we have also included the Print method in the Father class. So, in this case, first, the compiler will find the Print method in the Son class. After the unsuccessful search, it will go to the Father class, and hence it executes the Print method of the Father class.
Examples to Implement Multilevel Inheritance in C++
Example 1
Code
Output
Explanation
In the above example, we have declared a parent class as Airline, an intermediate class as Indigo derived from the Airline class, and a child class as Boeing747, derived from the Indigo class. Indigo is an airline company and has inherited the feature of the fastest means of transport from the Airline class. The Boeing747 class is inherited from the Indigo class as Boeing747 is one of the planes of Indigo Airlines. The feature of the Boeing 747 is that it can have a maximum speed of 660 mph.
Example 2
Code
Output
Explanation
In the above example, we have declared three classes, i.e., Shape, Area, and Cost, where the Shape class inherits the Area class. The Area class inherits the Cost class. In the Shape class, we are taking input from the user about the shape he wants to paint (Setting the string shapeName to user input). Nextly, we will use the shapeName data of the Shape class in the Area class. In the Area class, we will take the dimensions of the selected shape accordingly and calculate the area. Finally, we will use the area data to calculate the cost of painting the shape in the Cost class.
Example of Constructor in Multilevel Inheritance
A constructor is a special method called as soon as the object of the class is created. Constructor has the same name as its class. We will be taking an example to understand the flow of multilevel inheritance in the case of a constructor.
Code
Output
Explanation
In the above example, we have three classes, i.e., Parent, D1, and D2. Where the D1 class is derived from the Parent class. The D2 class is derived from the D1 class. Whenever we create an object of class D2 class, the constructors of all the parent classes get evoked. The order in which they execute is from higher order to lower order (Base class to lowest derived class). Therefore, the constructor of the Parent class is called first, and then the constructor of the derived class D1 is evoked. Finally, the constructor of the derived class D2 is evoked.
Difference Between Multilevel and Multiple Inheritance in C++
Multilevel Inheritance | Multiple Inheritance |
---|---|
In Multilevel Inheritance, the parent shares inherits with the kid, and the child then becomes the parent of another class, sharing the parent's resources with its offspring. | In Multiple Inheritance, each child can be derived from two or more parents. |
Multilevel inheritance can have multiple levels of inheritance. The base class is the parent of all the classes. | Multiple Inheritance has two class levels, i.e., base class (parent class) and derived class (child class). |
Multilevel inheritance is easy to implement and is widely used in various applications. | Multiple Inheritance is complex in nature and is not preferred widely. |
Conclusion
-
In multilevel inheritance, a child class inherits all its parent classes' properties.
-
The base class is the parent class of all sub-classes in multilevel inheritance.
-
Inheritance goes from parent to child. Parent classes cannot access the properties of the child class.
-
In the case of constructors, the execution of constructors goes from top to bottom (from the parent class to the child class).
Learn More
Want to learn more about inheritance in cpp, here is an awesome blog by scaler topics.