Multiple Inheritance in Java
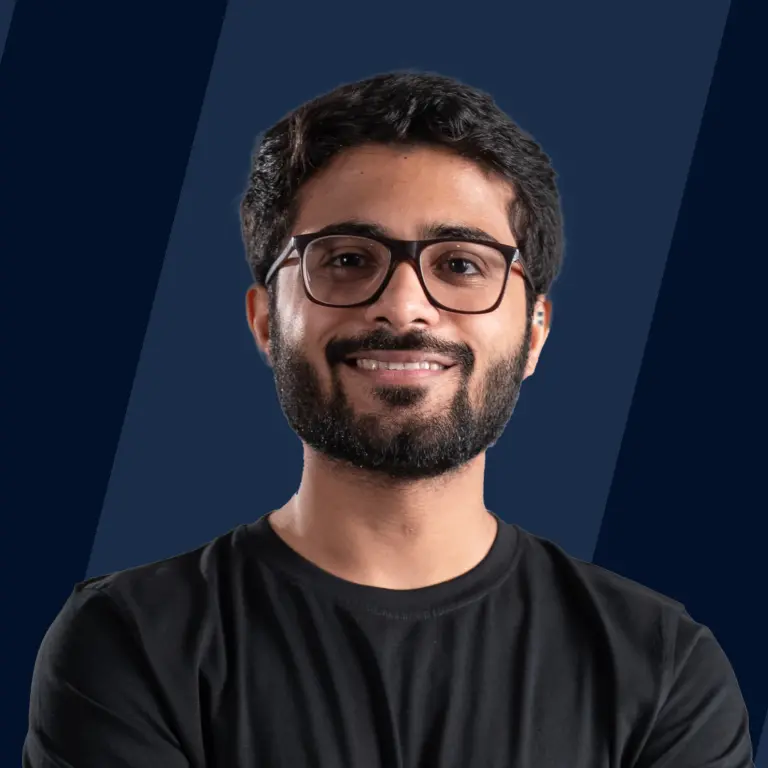
Overview
Inheritance is one of the fundamental concepts of Object-Oriented Programming (OOP).
By definition, Inheritance is the process in which a class inherits all the properties (including methods, functions, variables) of another class. However, Multiple Inheritance is the process in which a class inherits properties from more than one class.
Java doesn't support Multiple Inheritance, but we can use interfaces (instead of classes) to achieve the same purpose.
What is Multiple Inheritance?
Multiple Inheritance is the process in which a subclass inherits more than one superclass.
In the below image, we can observe that Class C(sub-class) inherits from more than one superclass i.e., Class A, Class B. This is the concept of Multiple Inheritance.
Many real-world examples of Multiple Inheritance also exist. For example, consider a newly born baby, inheriting eyes from mother, nose from father.
Kindly note that Java does not support Multiple Inheritance, but we can use Interfaces to achieve the same purpose.
Now we will be discussing an example to see what happens when we try to implement Multiple Inheritance in Java.
Example of Multiple Inheritance in Java
Definitely, there must be a reason why Java doesn't support Multiple Inheritance. Before we discuss the actual reason behind this, Let's see what happens if we try to implement Multiple Inheritance in Java.
Observe the below program for Multiple Inheritance Program in Java:
- The below Java program has three classes A, B, C
- Class A, class B contains a method with the same name i.e. execute()
- Class C inherits both class A, and class B
- In the Main class, we created an object of class C with the name obj itself
On executing the above code, we could find an error, i.e., an arrow mark pointing towards class C. This is because the compiler is confused about whether to call the execute() from class A or class B.
Why Multiple Inheritance is Not Supported in Java?
Implementation of Multiple Inheritance in Java is not supported by default to avoid several ambiguity issues.
One kind of ambiguity is the Diamond Problem. Let's understand this ambiguity with the help of the below image:
- From the below image, we can observe that class A is inherited by classes B and C.
- Also, observe that classes B and C are inherited by class D. This is where the actual ambiguity arises.
- Assume that classes B and C both contain the same method(say Perform()) with the same signature. (Perform() can be a method in A that B and C have overridden). If an object is instantiated for class D, and this object is used to call the method which is present in both class B and C, take a moment to understand the scenario.
- Compiler would be confused because the compiler does not know which class it should call to execute the method(Perform()) as it is present in both classes.
- This is the actual reason why Multiple Inheritance in Java is not supported.
An Alternative to Multiple Inheritance in Java
We have discussed that Multiple Inheritance in Java is not supported.
Now Let’s discuss an alternative approach for implementing Multiple Inheritance in Java. We can take the help of Interface for the implementation of Multiple Inheritance in Java. Java Interface is a blueprint of a class that contains abstract methods.
Let us look at the below program for a better understanding:
- In the below code, we have declared two abstract methods, i.e. execute1() and execute2().
- Both the methods execute1() and execute2() are written in interface A and interface B, respectively.
Output:
By seeing the above output, we can come to a conclusion that a Java class can extend two interfaces (or more), which clearly supports it as an alternative to Multiple Inheritance in Java.
Let’s look at another example where two Interfaces contain the same method with the same signature.
As we already know that when base classes contain a method of the same signature, then ambiguity occurs for the child class. Let's see how this could be managed by using the interface. Observe the below code
- In the below code, we have two interfaces, i.e., interface A, and interface B, along with an implementation of class C and Main class.
- Here, interface A and interface B contain the same method, execute().
- The above two interfaces are implemented by the implementation Class C.
Output:
From the above output, we could observe that though interface A and interface B contain the same method with the same signature, its implementation is provided in the implementation class C. Interface methods are abstract by default, so they don't contain a definition, due to which there is only one definition of execute(), and the object of the child class had only to call that.
By now, you would have a clear idea of how we could eliminate the Diamond problem by implementing Multiple Inheritance in Java through Interfaces.
Conclusion
- Inheritance is one of the core concepts of Object-Oriented Programming.
- Multiple Inheritance is the process in which a subclass inherits more than one superclass.
- Java does not support Multiple Inheritance; however, Java interfaces help us achieve Multiple Inheritance of type in Java.
- Implementation of Multiple Inheritance in Java is not supported by default to avoid several ambiguities such as Diamond problem. (This, too, can be solved by using interfaces).
- By default, Java cannot extend two classes but can implement two or more interfaces.