Python Nested Loops
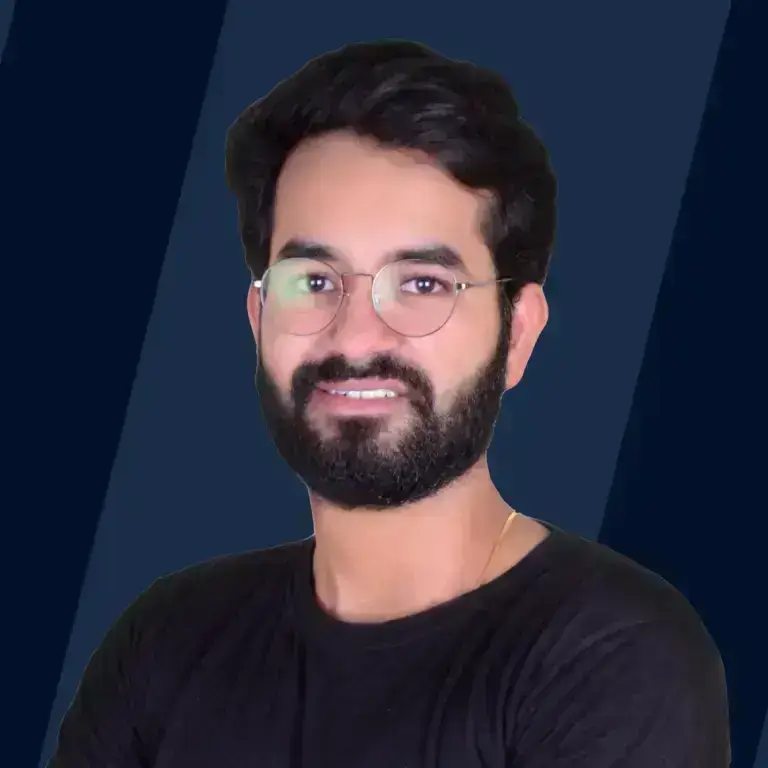
Nested loop in Python involves the inclusion of one loop within another. This construct allows for iterating over elements in multiple dimensions, such as rows and columns of a matrix. By nesting loops, complex data structures can be traversed efficiently, offering versatility in problem-solving and algorithm implementation.
Python Nested for Loop
Syntax of Using a Nested for loop in Python
The x loop or outer loop is executed several times. And for each execution of the x loop, the y loop is getting executed b number of times. Hence the statement of y loop is executing a*b number of times.
Example: Printing multiplication table using Python nested for loops
For a number n, we have to generate a multiplication table of size n*n. Let n=5; then we have to generate the multiplication table as,
Like the above problem, the outer loop is iterating over rows from row=1 to row=n. In each outer loop iteration, the inner loop runs n times from col=1 to col=n. In each execution of the inner loop, we print the product of the row number and column number. Once the row is printed, a new line should be printed.
But note that the row and column numbers also start from 1, instead of 0. Hence we will pass two arguments to the range function.
Output:
Nested while Loop in Python
The syntax for a nested while loop statement in Python programming language is as follows:
Syntax:
Example:
The following program uses a nested for loop to find the prime numbers from 2 to 100:
Code:
Output:
Nested Loop to Print Pattern
Consider the pattern for n=5 rows.
If we carefully observe, in each row, we are printing the * row number times, like if the row number is 1, we print * 1 time. If the row number is 2, we print * 2 times. If the row number is 3 we print * 3 times, and so on.
Now let's see how we can break this problem in the form of a nested loop in python. For n=5, there are 5 rows; therefore, we need a loop to iterate over the rows. Hence, the outer loop should iterate over the rows. In each row, * is printed row number times. Therefore the inner loop should iterate row number times and print * in each inner loop execution. Once the row is printed, a new line should be printed.
But note that the range(a) generates a sequence from 0 to a-1, but our row number starts from 1 to n; hence we will pass two arguments to the range function.
Output:
Using break statement in nested loops
The break statement is used inside the loop to exit out of the loop. If the break statement is used inside a nested loop in python, it will terminate the innermost loop. In the following example, we have two loops. The outer for loop iterates the first four numbers using the range() function, and the inner for loop also iterates the first four numbers. If the outer number and a current number of the inner loop are the same, then break the inner (nested) loop. Code:
Output:
As you can see in the output, no rows contain the same number.
Using continue statement in nested loops
The continue statement skip the current iteration and move to the next iteration. In Python, when the continue statement is encountered inside the loop, it skips all the statements below it and immediately jumps to the next iteration.
In the following example, we have two loops. The outer for loop iterates the first list, and the inner loop also iterates the second list of numbers.
If the outer number and the inner loop’s current number are the same, then move to the next iteration of an inner loop.
Code:
Output:
As you can see in the output, no same numbers multiplying to each other.
Single line Nested loops using list comprehension
For example, if you had two lists and want to get all combinations of them, To achieve this, you need to use two nested loop in python as mentioned below.
Code:
Output:
When To Use a Nested Loop in Python?
- Nested loop in python is handy when you have nested arrays or lists that need to be looped through the same function.
- When you want to print different star and number patterns using rows and columns.
- For matrix operations such as multiplication, addition, or transposition, nested loops are used to iterate through rows and columns.
- In some searching or sorting algorithms, nested loops may be needed to compare elements across multiple dimensions.
Conclusion
- A nested loop in python is a loop inside a loop. Therefore a nested for loop in python is a for loop inside another for loop.
- The inner for loop is executed for each execution of the outer for loop. If the outer for loop has range(a) and the inner for loop has range(b), then the inner for loop executes a*b times.
- Nested for loop in python can be used to solve problems such as prime numbers in a given range, pattern printing, multiplication table printing, etc.