What is Currying in JavaScript?
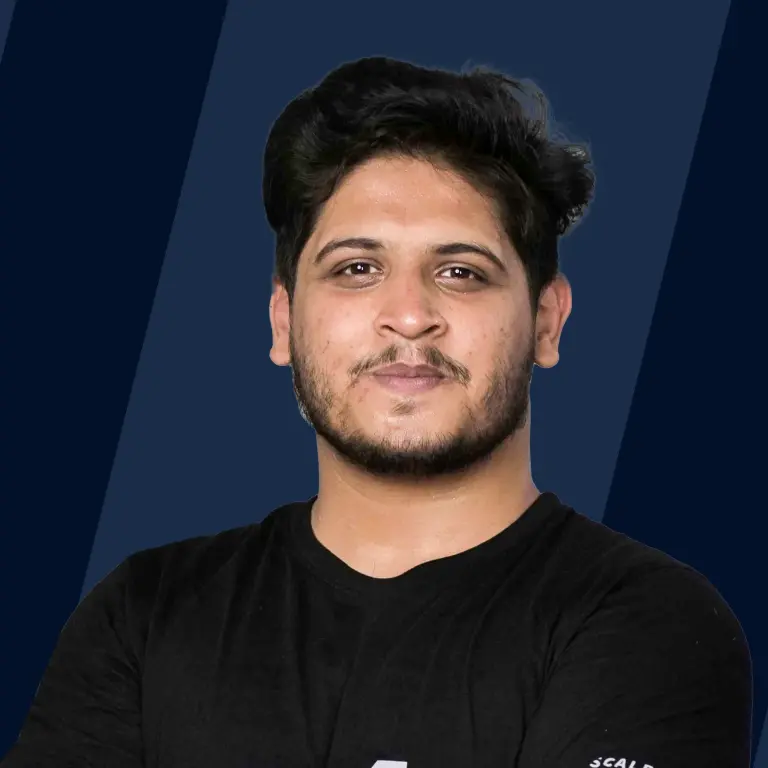
Currying is an advanced function in JavaScript which is used for the manipulation of functions' arguments and parameters. It was named after "Haskell B. Curry". The concept of currying in Javascript comes from the Lambda calculus.
Currying takes a function that receives more than one parameter and breaks it into a series of unray (one parameter) functions. Hence, the currying function takes only one parameter at a time.
Uses of Currying Function
Currying in JavaScript can be for the following reasons:
- Currying is helpful in Event handling.
- By using the currying function, we can avoid passing the same variable many times.
- Currying in JavaScript can be used to make a higher-order function.
How does Currying Work?
As we know, currying in JavaScript is a function that takes multiple arguments. In it, the function is transformed into a number of functions, and each of these series of functions will accept one argument.
Example One: A Simple, Three-Parameter Function
In this example we will see a simple function that will accept three parameters:
Output:
Explanation: In the above example, we have given three parameters of which addition is performed and the result is printed as the output.
Example two: Converting an Existing Function into a Curried Function
In this example, we will use see the implementation of the currying function where one argument will be accepted by the function and it will return a series of function.
Explanation: In the above example, we '1' is passed to the function "sum". It will return a function like:
Now, the above function definition will be treated as "sum1" and takes "b" as an argument. Then we will call the function "sum1" and pass this in 2. Then the sum1 will return the third function as-
Now, the returned function will be stored in the variable "sum2". Then the function "sum2" will be called and it will take '3' as the parameter.
Now, the calculation will be performed with the previous parameters x,y, and z as 1,2, and 3 respectively and it will return 6.
Example Three: Creating a Friend Request Curry Function
In this example, we will create a curry function where a friend user can send friend requests to his/her friend.
Output:
Explanation: In the above example, a function "sendReq" is created, and "greet" is its only argument. This function will return the name of the person and the message for the person as specified by the user. The result will print as output when the function is invoked.
Basic vs. Advanced Currying Techniques
Basically, there are two types of Currying in JavaScript. Let us have a look at them.
- Basic Currying
- Advanced Currying
In Basic currying, a single argument is taken by the function and returns a series of functions under which all the other parameters are contained.
In this currying, the function is incomplete until all the parameters are received by the function. Otherwise, the function will not give any fruitful results.
While in Advanced currying, there is a fixed number of parameters in the function. In it, the function is received as an outer function which is a wrapper function.
Let us see examples of both the currying in JavaScript for better understanding.
- Basic currying:
Explanation: In the above code, a function "getCakeIngredients" is created whose argument is "ingred_1". This "ingred_1" will return a series of functions as other ingredients that we required for making the cake. Thi function will be incomplete until it receives all the ingredients as a parameter.
- Advanced currying:
Explanation: In the above example, the "Adv_curry" function is received as the outer function which is a 'wrapper function'. Here one more function is 'curried' returned which receives arguments with an operator named 'spread operator'.
This operator is for comparison of the function length 'fn.length'. Spread operator in JavaScript is used to fast copying of array or object into another array or object. This copying can copy all the parts or some part of the array or object as specified by the user.
The number of arguments passed here will show the length property of the function. The arguments will keep increasing every time the calling of function.
Modern Currying With ES6
ES6 is the new modifications and improvisations in the syntax of JavaScript. The arrow function is used for currying in JavaScript. Modern currying can be done using this modern syntax as given in the below example.
Output:
Currying Can be Used to Manipulate the DOM in Javascript
Currying in JavaScript is also used to modify the Document Object Model in JavaScript. As we know, in currying a function is rewritten with multiple arguments into a series of functions. This affects the appearance of the whole structure of a web page.
Currying vs. Partial Application
- Currying: A function in which multiple arguments are accepted is known as the Currying function. By applying the currying function, a function is divided into a series of many functions and each of these functions will accept a single argument unless all the parameters are accepted.
- Partial Functions: The idea of the partial application comes from the fact that you can take a system and then split them into multiple systems. In partial application, we create a new function by prefilling some of the arguments to the original function.
Let us see an example and understand the partial application.
Explanation: In the above example, a function is created that will add the numbers and return the result as output. A partial function is one that converts a function into another function but a number of arguments is less there.
Take the first step towards becoming a JavaScript wizard. Enroll in our JavaScript full course and get certified!
Conclusion
- Currying in JavaScript is a method that takes one argument at a time and returns a new function that expects the next argument.
- Using currying, higher-order functions can be created in functional programming.
- Using Currying, we can also modify the DOM version in JavaScript.
- Event listeners can be triggered using currying.