IIFEs in JavaScript
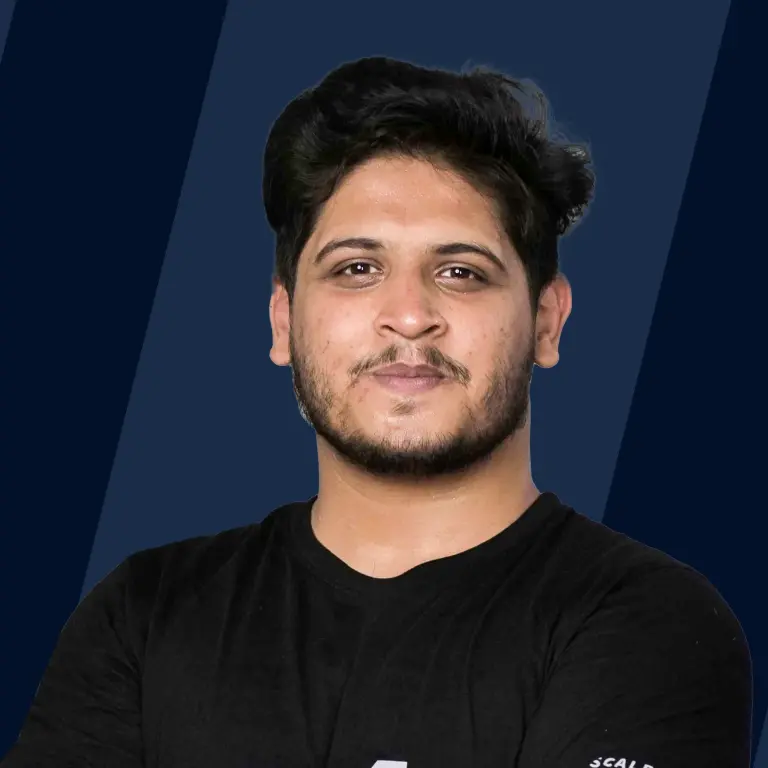
Overview
IIFE is a function expression that is called immediately as soon as it is defined. IIFE in javascript is one of the good ways to hide data from the global scope as it creates its own scope. IIFE in javascript is used often while creating your own javascript library to avoid unnecessary conflicts like duplicate variables and functions in the global scope.
What is an IIFE?
Considering we want to build our own javascript library like jQuery. Then, there is a high chance that the variables or functions with the same name came into existence on a global scope as a lot of people will use our library.
Hence, we use IIFE in JavaScript which helps us to avoid duplicate variables and functions in the global scope as IIFE keeps these variables and functions private in its own scope.
IIFE, Immediately Invoked Function Expression, is called immediately as soon as the function is defined. We do not need to call the function specifically with its name. We use IIFE to avoid overwriting variables and functions in the global scope and keep their scope to IIFE in Javascript.
Syntax
Explanation of The Syntax:
- Round brackets () are used to define IIFE in Javascript.
- The function within closed brackets is an anonymous function i.e. function didn't have any name.
- Another pair of round brackets () is used to call the function immediately just like we call the named function after declaration.
Why is It Called Immediately Invoked Function Expressions?
In the above code block, there are two parts:
1. Function Expression
We wrapped the function with square brackets that convert the function into a function expression. Hence, IIFE includes the phrase Function Expression.
2. Function Call
We called the function with another square brackets just after creating the function expression. Hence, we can say that the function is called immediately. Hence, IIFE includes the phrase Immediately Invoked.
IIFE Starting With a Semicolon (;)
The Problem:
Note: If you run the above code, it will cause an Uncaught TypeError: callMe(...) is not a function. And it concatenates the callMe function with IIFE next to it.
The Solution:
The above code will result in syntax errors during execution. Hence, we use semicolons to avoid unnecessary concatenation with the previous/next line of javascript code.
Converting Functions to IIFEs
Normal Function
IIFE in Javascript
As we are already aware of the IIFE syntax. Hence, we enclose the regular function within square brackets that converts the regular function to function expression and add another square bracket to invoke the function immediately as shown below.
Also, we can add the exclamation mark at the start to convert the normal function to function expression and then call it immediately.
Note: We can use named as well as anonymous functions to define IIFE in javascript.
jQuery & IIFE
Let's have an example
In the above example, we are using the $ symbol to use jquery in our javascript file and selecting the paragraph to apply the hide effect to the paragraph. Also, we have our customized function in the same javascript file.
Now, the Hide effect would not work for paragraphs because we override the jquery object $ to variable $. Hence, jQuery use IIFE to avoid these conflicts. Let's see, How?
Apply IIFE to JQuery Code
In the above code block, we wrapped the code inside IIFE. We use the parameter _ safely without worrying about the global scope as it is local to IIFE and pass the jQuery as an argument. We can also replace the parameter _ with anything you like as shown below.
In the above code block, we pass _ as a parameter and provide jQuery as an argument to IFEE.
Why Use Immediately Invoked Functions Expressions?
Consider there are three files named file1.js, file2.js, and file3.js
In the above code, if we include all these javascript files in the same code. The variable will be overwritten as they exists in same scope (global). Let's fix this problem.
In the above code block, we converted normal functions into IIFE and assigned a return value to a variable as it creates its local scope and prevents overriding the variable name.
Conclusion
- IIFE in Javascript is a function expression that is invoked immediately.
- Square brackets are used to define IIFE.
- Function within the square brackets could be named or anonymous.
- It doesn't work for function statements/declarations.
- We can access any objects/variables from the global scope by passing them as arguments.