What are Higher Order Functions in JavaScript?
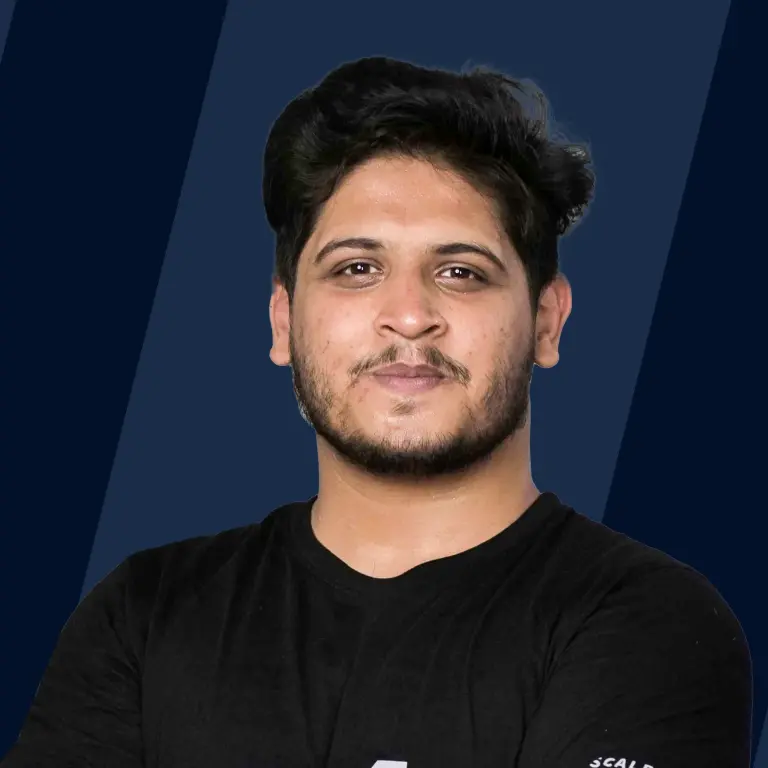
Consider an example of a simple function,
In the above code block, We are using the getNum function to get the number. Here, getNum is a first-order function. Let's write another function,
In the above code block, We wrote a function that returns a function. Hence, the twiceNum is a Higher order function. Therefore, The function that returns a function, operates on another function, or takes another function as an argument is called a higher-order function in javascript.
The Higher-order Functions in Practice
- In the above code block, we wrote two simple methods sum and mul that perform the addition and multiplication of two numbers respectively.
- Then, We wrote another function that can take two arguments : operation as a function and numsArray as an array. Hence calculate function is a higher-order function.
- calculate(sum, [5, 6]) uses the sum() method and performs addition on the numbers in the array.
- calculate(mul, [10, 3]) uses the mul() method and performs addition on the numbers in the array.
Filtering an Array
Reducing an Array
We use the Array.reduce() method, which is a higher-order function in javascript, to reduce an array to a single value by applying a function to each element in the array.
For example :
Sorting an Array
We use the Array.sort() method, which is a higher-order function in javascript, to sort the elements of an array. By default, the sort() method sorts the elements in ascending order, but you can pass a comparator function as an argument to specify a different sort order.
For example :
Example
The Array.map(mapperFunc) Method
- In the above code block, We use the map() method to double each number of array numsArray.
- map() applies the callback function callbackFn to each element of the array. Then it returns a new array.
- Because the map() method accepts a function as an argument. Therefore map() is a higher-order function in javascript.
Element.addEventListener(type, handler) DOM Method
-
addEventListener() method attach an event handler to an element in the DOM. It takes two arguments :
- type :
A string that specifies the type of event to listen for (e.g. "click", "mouseover", "submit") - handler :
A function that will be executed when the event occurs
- type :
-
Here is an example of attaching an event listener to a button element,
Note :
addEventListener() is not a higher-order function. Because It responds to the event if any event occurs in the DOM. However, it accepts the callback function as an argument. but the callback function executes only if an event occurs in the DOM.
The Benefits of Higher-order Functions
- Reusability :
Higher order function helps to make our code more reusable. We can use the same higher-order function with different inputs including functions. The higher-order function makes it easy to write less and do more. - Abstraction :
Generally, We abstract properties or values with the help of a general function. We can also abstract the function or action with a Higher order function. Therefore, a higher-order function makes our code more flexible. - Readability :
Higher-order functions make our code more readable. Because We write specific logic into separate functions. Also, We can avoid repeating a logic in multiple places. Therefore, the Higher order function makes it easy to understand our code. - Modularity :
When we write higher-order functions, we break our code down into smaller, more modular pieces. These small pieces of code make it easy to maintain our code. - Efficiency :
Higher-order functions can make your code more efficient by allowing you to write more general, reusable code that can be applied to a variety of inputs. This can reduce the amount of duplicate code you need to register and maintain.
Beyond JavaScript Basics: Our Full Stack Web Developer Course Guides You Through Advanced Techniques. Enroll Now & Transform Your Coding Expertise.
Transforming with map()
map() takes a callback function as an argument and applies that function to each element in the array. And create a new array with the transformed elements.
For example :
Filter
The filter() method is a higher-order function in javascript that allows you to select elements from an array based on a certain condition. It takes a callback function as an argument and applies the callback function to each element in the array. And return a new array with only the elements that fulfill the criteria.
For example :
Summarizing with reduce()
The reduce() method is also a higher-order function in javascript that helps to iterate over an array and perform a calculation on each element, resulting in a single value. It takes a callback function as an argument and applies that function to each element in the array, starting from the leftmost element and progressing to the right. The callback function takes two arguments: an accumulator and the current element being processed. For example,
In the above code block, the callback function passed to reduce() takes two arguments accumulator total and the current element element. The initial value of total is set to 0. The reduce() method applies the callback function to each element of the array numbers.
Composability
In the above code block, We calculated the average year of origin for living and dead scripts separately. Here, we use two for loops that look the same except variable. So, we will use the higher-order function to calculate the average year of origin as shown below,
In the above code block, averageYearOfOrigin is a higher-order function. Because averageYearOfOrigin returns a function Math.round() that applies some math to give arguments.
Script Data Set
We can use the higher-order function in javascript to process any dataset as we did in the previous section. We can use filter(), reduce(), map() and any other custom higher-order function. In the previous section, we used the script data set to process the information. The script is a kind of format like an alphabet to form words or sentences. Here is an example of the script data set,
Higher-Order Functions vs Callback Functions
-
Higher-order functions take other functions as arguments or return a function as output.
-
On the other hand, callback functions are passed as arguments to other functions and called later when needed.
-
We can use Callback functions in conjunction with higher-order functions.
-
Here is the comparison table of higher-order function vs callback function,
Terms Higher-Order Functions Callback functions Definition A function accepts a function as an argument or returns a function in the output. A function that is passed to another function. But the function is called later when Any event occurs. Objective A function that helps to make our code more readable and reusable. A function that waits for a particular event and is called later if that particular event occurs. Example There is a built-in higher-order function in javascript. For example : map(), filter(), reduce() Generally timer functions are a good example of a callback function. For example: addEventListner(), setTimeout()
Browser Compatibility
-
Most modern browsers support the use of higher-order functions in JavaScript.
-
Here is a list of the most common browsers that support higher-order functions like forEach(), map(), filter(), reduce(), some(), and every().
Supported Browser Version Chrome 108 Edge 108 Firefox 107 Safaari 16.2 -
Other browsers that support higher-order functions are DuckDuckGo, Brave, Samsung Internet, Vivaldi, etc.
Your journey to becoming a certified Node.js developer starts here. Enroll now in our Free Node JS certification course and set yourself on the path to a rewarding career.
Conclusion
- Higher order function can accept a function as an argument and return a function in the output.
- Practically, We can use in-built higher-order functions like map(), reduce(), filter(), sort(), etc. Also, We can create a custom higher-order function.
- addEventListner() is a callback function but We can use it with the higher-order function.
- Higher order function helps to increase the code reusability, improve the readability and make the code more efficient.
- Combining higher-order functions with other functions can help simplify complex functionality and make the code more reusable and suitable for different scenarios.
- We can process and modify a data set using a higher-order function.