Non-primitive Data Types in Java
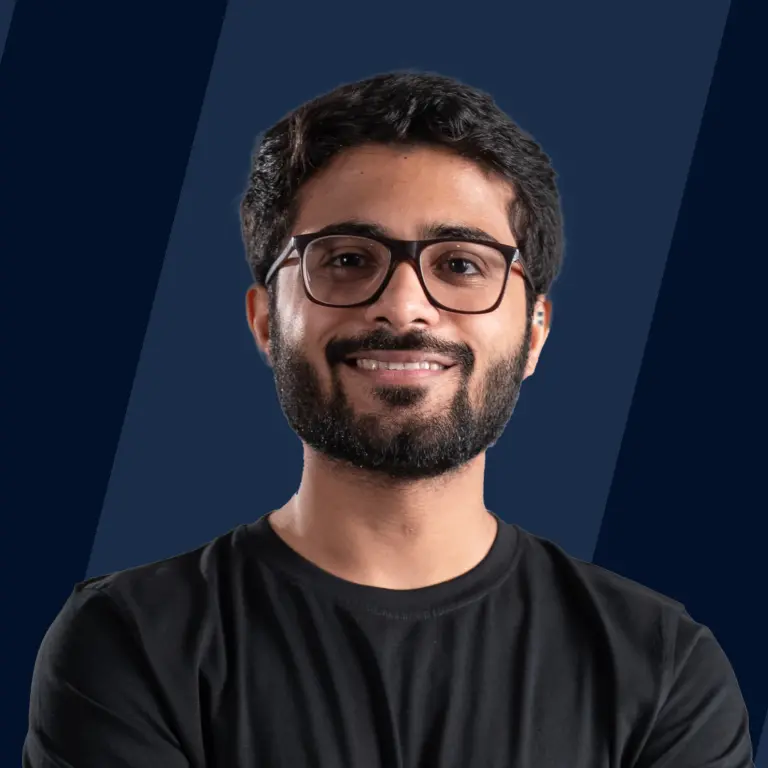
In Java, data types tell us what kind of information we can store in a variable. Besides primitive data types, there are more complex types called non primitive data types in Java. These help us organize data in more advanced ways. For example, we have classes that act like blueprints for making objects, arrays for storing lists of things, interfaces that set rules for classes to follow, enums for naming specific options, and arrays of objects for storing many objects together.
Non-primitive Data Types
Non Primitive data types in Java are user-defined data types (except for String class) and they can be easily created by the users. They can be used to store multiple values and invoke methods to perform certain operations.
Non primitive data types in Java, like classes and arrays, reference memory locations in the heap where objects are stored. Hence, they're called referenced data types or object reference variables, pointing to where data resides rather than storing it directly.
Types of Non-Primitive Data Types in Java
1. Classes and Objects
Class is a user-defined data type that is used to create objects. A class contains a set of properties and methods that are common and exhibited by all the objects of the class.
Example
In the example below, we have created a class with the class name Demo outside the public Main class.
Output:
Explanation:
Upon creating an object of class Demo, we have invoked two functions with some parameters to perform addition and subtraction and the result will be then displayed.
2. String
In Java, strings are capable of containing sequences of characters within a single variable, unlike character arrays where each character is stored separately. Unlike in C/C++, there's no requirement to terminate strings with a null character. This design difference simplifies string handling and removes the need for explicit termination markers, enhancing the efficiency and ease of use in Java string operations.
Syntax for declaration of String:
Example 1: Standard String Declaration
In this Java example code, we have declared two strings: str1 and str2 using the non-primitive data type String and initialized them with some values.
Output:
Example 2: String Declaration Using new Operator
In this example, we have used an alternative method of declaring the string str1 using the new operator where the sequence of characters is passed as a single parameter to the new String() constructor.
Output:
3. Array
Arrays are non primitive data types in Java that are used to store elements of the same data type in a contiguous manner. Arrays have a unique reference name by which all their elements are accessed. Elements are stored in an indexed manner where the index starts from 0.
Key points to remember about Arrays in Java:
- In Java, all arrays are dynamically allocated and their size can be declared by the programmer dynamically at run time. This means memory space is allocated to Java arrays at run time rather than compile time.
- The size of an array must be specified by an integer value and not long or short.
Syntax of Array Declaration:
Usage:
Example:
In the example below, we have declared and initialized arr1 with elements 1,2,3,4,5 and for arr2 of size 5, the user will input the elements. After that, we print the values of elements of both arrays as output.
Output:
4. Interface
Interace in Java is a tool to achieve abstraction. Interface can contain non-implemented methods (without the method body) also known as abstract methods. This is the main difference between a class and an interface. Interfaces in Java may contain:
- Abstract methods
- Default and static methods (since Java 8)
- Private methods (since Java 9)
If a class implements an interface, then it must also implement all the abstract methods of that interface otherwise we need to declare that class as an abstract class.
Example:
In this Java example code, we have implemented an interface consisting of two abstract methods declarations: void mult() and void div() without their bodies. Now, they need to be implemented by the class Solve, where we will implement both methods.
Output:
Difference between Primitive And Non-Primitive Data Types in Java
- Definition: Primitive data types in Java are predefined by the language, whereas non-primitive data types in Java are user-defined.
- Storage: Primitive types store data directly, while non-primitive types store references to data locations.
- Size: Primitive types have fixed sizes, while non-primitive types have variable sizes.
- Default Values: Primitive types have default values, and non-primitive types default to null.
- Memory Allocation: Memory for primitive types is allocated on the stack, while memory for non-primitive types (objects) is allocated on the heap.
- Operations: Primitive types support basic operations directly; non-primitive types often require method calls.
- Usage: Primitive types are for basic data (e.g., numbers, characters), and non-primitive types handle complex structures (e.g., objects, arrays).
Conclusion
- Non-primitive data types in Java, defined by users, offer versatility beyond simple values.
- They include classes, arrays, strings, and interfaces, facilitating complex data structures.
- Non-primitive data types in Java reference memory locations, enhancing efficiency in handling data.
- Understanding these differences is crucial for effective Java programming.
- Mastery of both primitive and non-primitive data types in Java is essential for building robust software.
- Their usage empowers developers to implement object-oriented programming paradigms effectively.