NumPy Data Types
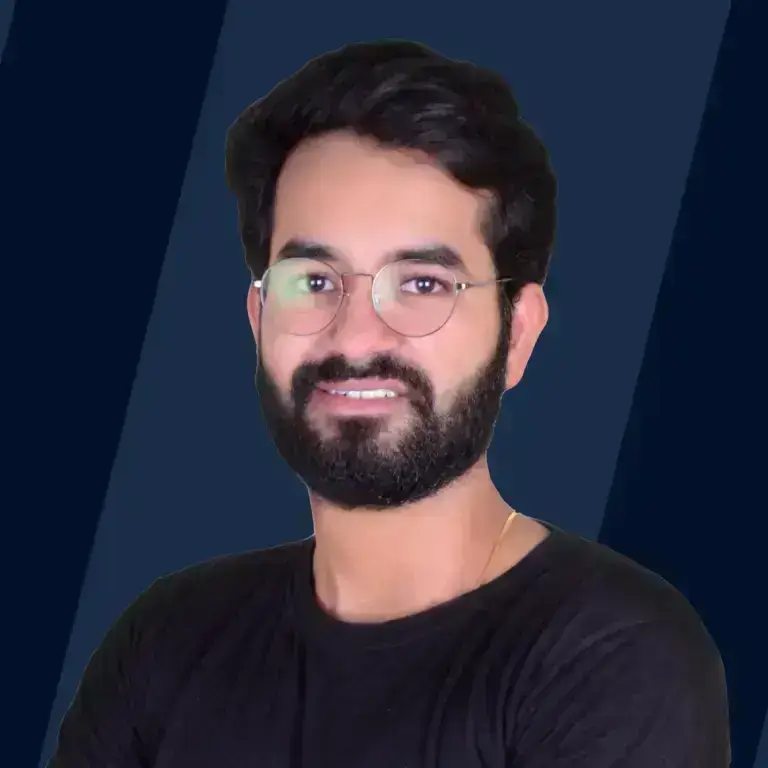
Overview
We will discuss data types in the Python NumPy module in this tutorial. All of an array's components in Numpy are datatype objects or NumPy dtypes. The fixed size of memory for an array is implemented using the data type object. Python supports a fundamental set of data types. Compared to Python, NumPy provides a greater variety of data types, often called NumPy datatypes. The capabilities of NumPy are expanded by the wider variety of data types. For the elements in an array, there are more possibilities for specifying the data type.
Introduction
Python supports a fundamental set of data types. Compared to Python, NumPy provides a greater variety of data types. The capabilities of NumPy are expanded by the wider variety of data types. For the elements in an array, there are more possibilities for specifying the data type. All of an array's components in Numpy are datatype objects or NumPy dtypes. The fixed size of memory for an array is implemented using the data type object. Specifically, it informs us about the following:
- It provides details on the different types of data (that is, integer, float, or Python object)
- It informs us of the size of the data.
- It explains the Byte order (little-endian or big-endian)
When it comes to structured types, it provides information on the names of the fields, their respective data types, and the amount of RAM each field occupies. If the data type is a subarray in this instance, it provides information on the shape and data type. In this, the data type's prefix " or '>' determines the byte order, where the symbol "<" indicates little-endian encoding (in which the least important data is put in the smallest address) and the symbol ">" indicates big-endian encoding (a most significant byte is stored in the smallest address).
NumPy Datatypes
Various additional data types in NumPy only have one character, such as i for integers and u for unsigned integers.
The characters used to represent each NumPy datatype are listed below.
Character | Meaning |
---|---|
i | integer |
b | boolean |
u | unsigned integer |
f | float |
c | complex float |
m | time delta |
M | DateTime |
O | object |
S | string |
U | Unicode string |
V | a fixed chunk of memory for other types ( void ) |
Numpy Scalars and Array Scalars
Python only defines one type of a specific data class called scalars (there is only one integer type, one floating-point type, etc.). This may be useful for applications that don't have to worry about all the different ways that data can be represented on a computer. But for scientific computers, additional control is frequently required.
There are 24 new fundamental Python types in NumPy that are used to express various scalar kinds. With a few additional kinds that are compatible with Python's types, these type descriptors are primarily based on the types accessible in the C language, in which CPython is built in.
The attributes and methods of ndarrays and array scalars are identical. Removing the sharp edges created when combining scalar and array operations enables one to handle some items of an array like how arrays are treated.
-
Some Built-in Array scalar types in NumPy Some of the scalar types inherit from both the generic array scalar type and some of the basic Python types since they are fundamentally equal to those kinds:
Array Scalar Python Equivalent Inheritable int_ int Only in Python 2 float_ float Yes str_ str Yes bool_ bool Yes complex_ complex Yes bytes_ bytes Yes timedelta64 datetime.timedelta Yes datetime64 datetime.datetime Yes -
NumPy Scalars
NumPy datatypes (Scalar) Description bool_ Boolean true or false values int_ Default integer type (int64 or int32) intc Identical to the integer in C (int32 or int64) intp Integer value used for indexing int8 8-bit integer value (-128 to 127) int16 16-bit integer value (-32768 to 32767) int32 32-bit integer value (-2147483648 to 2147483647) int64 64-bit integer value (-9223372036854775808 to 9223372036854775807) uint8 Unsigned 8-bit integer value (0 to 255) uint16 Unsigned 16-bit integer value (0 to 65535) uint32 Unsigned 32-bit integer value (0 to 4294967295) uint64 Unsigned 64-bit integer value (0 to 18446744073709551615) float_ Float values float16 Half precision float values float32 Single-precision float values float64 Double-precision float values complex_ Complex values complex64 Represent two 32-bit float complex values (real and imaginary) complex128 Represent two 64-bit float complex values (real and imaginary)
Overflow Errors
Let's look at how NumPy Python handles overflowing NumPy datatypes. When a value exceeds a data type's storage capacity, it becomes an overflow condition; the solution in Python is to switch to a different data type.
Let's take a look at this using the numpy.exp() function.
Code:
Output:
This warning appears because the largest data type that NumPy will accept is float64, which has a maximum range of 1.7976931348623157e+308. The warning is generated because the value is greater than this.
Let's see how to fix this.
To fix this, we need to convert float64 data types to float128 data types.
Code
Output:
Extended Precision
The extended precision floats with real and imaginary parts serve as the representative for the NumPy datatype for complex numbers in the NumPy package.
The highest NumPy datatype represented in NumPy by default is the float64 representation, and for complex float numbers, it is the complex128 representation. But we often happen to deal with numbers and values which exceed the limits of these representations. In those times, we mention the extended precision representation explicitly.
Let's make this clear by using some examples.
- float128
Code:
Output:
Here by default, the NumPy datatype of the float64 representation was to be used, but the numeric value generated by the np.exp() function exceeded the limit of the float64 representation. Hence we resorted to extended precision by explicitly mentioning the float128 NumPy datatype representation.
- complex256
Code:
Output:
Similar to the above example of float64, here the complex128 numpy datatype representation could not hold the generated value, and by using the np.clongdouble() function, we extended the NumPy datatype to complex256 representation.
How to Check the Data Type of an Array
The dtype attribute of the NumPy array object returns the array's data type:
Syntax:
object: (mandatory) The object that is to be converted to a data type is represented by this parameter.
Code:
Output:
Here the dtype function returned int64 as the output. It means that every element of the array is of a 64-bit integer data type.
Code:
Output:
Here the dtype function returned <U6 as the output. It means that every element of the array is of a 64-bit Unicode string, and the encoding used is the little-endian (that is the least significant and is stored in the smallest address).
How to Create Arrays With a Defined Data Type
The numpy.array () function is used to generate arrays; it accepts the argument dtype to assist in defining the NumPy datatype for the array's members.
Code:
Output:
Here we converted the NumPy array into the specified NumPy datatype by providing the argument dtype for the numpy.array() function.
What if We Cannot Convert a Value?
NumPy raises a ValueError if a type is specified in which elements cannot be cast.
Code:
Output:
At first look, you might be confused about how NumPy converts elements with different data types into an array. Well, look again. It is not of different data types, but rather the numerical values are represented as strings. Also, we can see that dtype returned <U1, which means it is encoded in 1 bit (Unicode string) representation of the little-endian encoding pattern.
Now let's see what happens when we try to change this array into a floating point array.
Code:
Output:
ValueError: In Python, ValueError is produced when an unexpected or wrong argument type is supplied to a function.
Therefore we established the fact some conversions are not possible with NumPy datatypes, much similar to Python data types.
When we try to convert a value that cannot be converted in NumPy, Python throws a ValueError.
Converting Data Type of Existing Arrays
By using the astype() function, we will convert the datatype in the example below from float to integer:
Code:
Output:
Conclusion
Let's see what we have learned here.
- NumPy datatypes inform us about details on the different types of data (that is integer, float, or Python object) and also about the size of the data.
- Different NumPy datatypes are used for various purposes.
- How to deal with overflow errors concerning NumPy datatypes.
- Extended precision is used when the default NumPy datatypes cannot represent the given value.
- How to check the data type of an array in NumPy using the dtype function.
- ValueError is thrown by Python when we cannot convert a value to the specified choice.
- We can convert the NumPy datatype of an already existing NumPy array to another type using the astype function.