Basic Syntax and First Program in NumPy
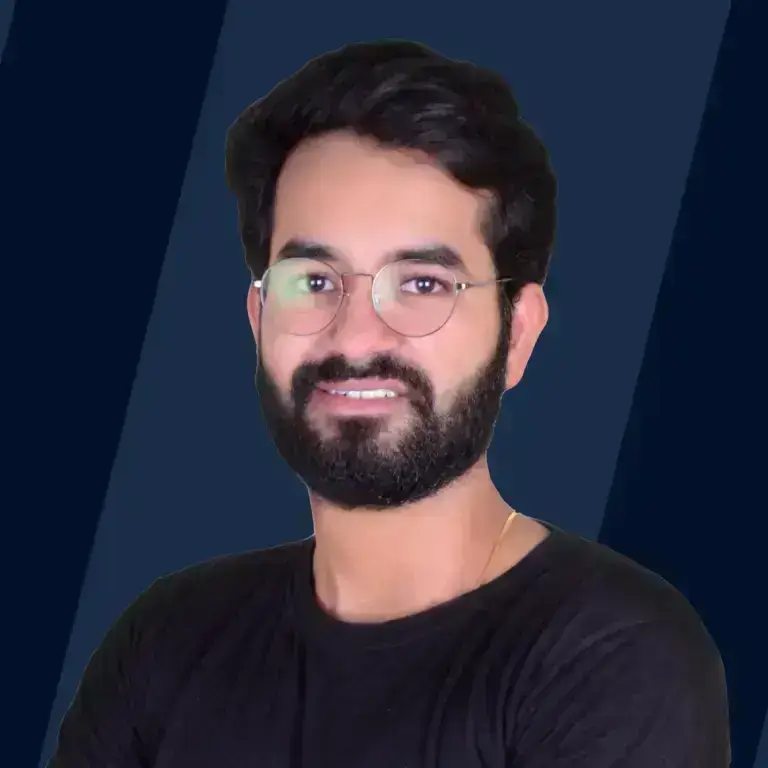
Overview
This article is a brief introduction to the syntax of the NumPy program in python, an open-source python library. Correct syntax helps to run the program or code in an error-free and efficient manner.
Introduction
To run the Numpy program error-free and correctly, the code should be written in a proper format. The methods for writing and creating NumPy arrays in Python using basic syntax are discussed in the below article.
Numpy Basic Syntax
The syntax is a set of pre-defined instructions that should be followed to run an error-free code.
Numpy should be installed before running any Numpy program.
The steps to install Numpy are as follows :
- Download python from www.python.org according to the operating system.
- After installing python go to the command prompt and type python to create a python environment.
- Install Numpy by using a python library called pip by using the command :
Basic Syntax of NumPy as follows :
- Need to import NumPy library from python by using the following command :
- np is the attribute that can be used in place of NumPy whenever creating a NumPy program in python.
Let's see some examples below to understand the Syntax for creating the Numpy Program in python :
-
Creating a numpy program in python using python list :
Syntax :
Code :
Output :
Explanation :
While creating an array arr through the list, elements should be in [] braces, and array [ 2 4 6 8] will be printed as output. -
Creating a numpy program using a tuple :
Syntax :
Code :
Output :
Explanation :
Creating an array arr by using the tuple elements should be in () braces, and array [ 2 4 6 8] will be printed as output. -
Numpy program for creating arrays of zeros :
Syntax :
Code :
Output :
Explanation :
zeros function is used to create the numpy array in python arr of zero values. The print statement will execute the array of zero values of shape(2,3) of datatype float.- Shape :
is for defining the shape of the array - dtype :
is the datatype of the array - order :
is whether multi-dimensional data should be stored in memory in row-major or column-major order.
- Shape :
-
Numpy program for creating arrays of ones :
Syntax :
Code :
Output :
Explanation :
The ones function is used to create the array arr of ones. The print statement will execute the array of one value of shape (2,3) of the data type integer.- Shape :
is for defining the shape of the array. - dtype :
is the datatype of the array. - order :
is whether multi-dimensional data should be stored in memory in row-major or column-major order.
- Shape :
-
Creating an array of random values :
Syntax :
Code :
Output :
Explanation :
The random function generates arrays of random elements of shape(2,3) i.e 2 rows and 3 columns. -
Creating array in range 0 to 3 of sequence 5 :
Syntax :
Code :
Output :
Explanation :
The linspace is a built-in function of the python numpy library which is used to create a linearly spaced array by giving the start value, end value, and the total number of elements inside the linspace function.In the above example, an array of 5 elements between 0 to 3 is generated.
-
Creating a sequence of integers with interval :
Syntax :
Code :
Output :
Explanation :
Inside the arange function, specify the start, end, and the difference between elements. An integer array of elements between 0 to 20 with a given difference of 4 between the elements is executed as output.
NumPy First Program
Simple Numpy programs in python :
Code :
Output :
Explanation :
Creating two arrays, arr1 using arange function of elements between 0 to 20 with difference 4 and arr2 of 4 elements using simple numpy.
2-D Numpy program
Note : Elements should be in [] brackets.
Code :
Output :
Explanation :
A 2-D array of 2 rows and 3 columns is executed as output.
3-D Numpy program :
Code :
Output :
Explanation :
A 3-D array of shape(2, 2, 3) is generated.
Where is the NumPy Codebase ?
The collection of source code of an application or software program is called a codebase.
Numpy Codebase is a community-driven open-source project where the source code of NumPy is developed by a diverse group of developers.
NumPy's source code can be found in this GitHub repository.
Conclusion
- NumPy is an open-source library of python programming language.
- The Basic syntax of the Numpy program in python is very easy to understand.
- Firstly Numpy needs to be imported from the python library and then the Numpy program in python can be created in many ways by list, tuple, and inbuilt functions like arange, random, etc.
- Numpy Codebase is an open source project where the source code of Numpy can be found at this GitHub repository.