Random Numbers in NumPy
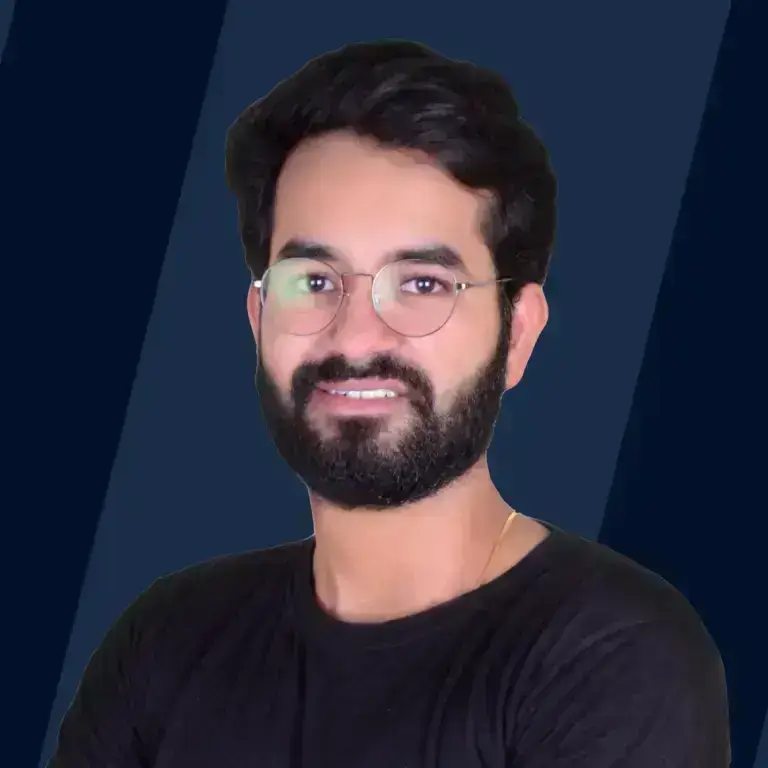
Overview
The core Python module for scientific computing is called NumPy. NumPy provides a programmer with a multidimensional array object, a collection of functions that allows them to efficiently use arrays, a lot of mathematical and logical functions, and much more. NumPy also helps us generate random numbers and arrays that are made up of random numbers.
In this article, we will deep-dive into various ways we can generate random numbers using NumPy in Python.
What is a Random Number in NumPy?
A random number in NumPy is produced using a huge sample of numbers and a mathematical process that gives each number in the given distribution an equal chance of occurring. These numbers are generally produced with the help of a special random number function.
Random numbers (or NumPy random randint) are used extensively in nearly all computer applications. The fields of statistical sampling and cryptography see the most use. Further in this article, we will have a look at the various algorithms that are used to create random numbers in NumPy.
Simple Random Data
The numpy.random() module consists of a lot of functions that allow us to generate arrays and collections of random numbers. To generate an array of random numbers, we use the numpy.random.rand() function.
Now that we have seen a simple example of random numbers using NumPy, let's understand the concept behind random number generation in Python and Pseudo-Random numbers.
Pseudo Random and True Random
Pseudorandom numbers are a collection of partially random numbers, as the name implies. Despite appearing random, they are not. In other terms, a pseudo-random number is a random number that was produced by a computer. If the beginning of the series is known, many numbers are created quickly and can also be repeated later. As a result, pseudo-random numbers are accurate and deterministic.
We need to obtain random data from some external source to generate truly random numbers on our computers. Typically, this external source consists of our keystrokes, mouse clicks, etc. But since gathering and collecting such data is difficult and tedious, we prefer pseudo-random number generators over truly random numbers.
Generate a Random Number
To generate a random integer, we use the random module from NumPy. Using the numpy random randint() function, we can generate a random integer from a range of 0 to a number of our choice. Let us generate a random number between 0 and 12 using the numpy random randint() function.
Output
Hence, we have successfully understood the working of the numpy random randint() function.
Generate Random Samples
-
Generate a Random Normal Distribution Sample
We can also generate random samples of a Normal Distribution using NumPy. The function to do that is called NumPy random normal().
Output
Hence, we have seen the implementation and working of the numpy random normal() function.
-
Generate a Random Uniform Distribution Data
Just as we created a random sample of normal distribution, we can do the same for uniform distribution as well. By using the numpy random uniform() function, we can generate random samples of uniform distribution.
Output
Hence, we have seen the implementation and working of the numpy random uniform() function.
Generate Random Float
To generate random float values, we use the rand() function provided by the numpy.random() module in NumPy. The rand() function returns a float value that lies between 0 and 1.
Output
Generate a Random Array
-
Generate a Random Array of Integers
We can use the numpy.random() module to generate an array consisting of random integers. To do this, we need to use the numpy random randint() function and add a parameter defining the size of the array we want.
Output
-
Generate a Random Array of Floats
To generate an array of random floats, we simply use the random() function with a parameter that specifies the size of the array that we want.
Output
Generate a Random Number from the Array
The applications of the numpy.random() modules in NumPy are endless. From generating random samples for statistical distributions to finding out a random number from an array, we can do it all using the random() module. In this section, we will once again use the random() module to choose one element at random from an array.
To perform this, we use the numpy random choice() function. NumPy random choice() is a function that enables us to generate a random value from an array of data, regardless of the types of those values. Since it's a part of the numpy.random() module, we can use it with the numpy.random() function.
Output
Hence, we have successfully implemented the numpy random choice() function.
Conclusion
- In this article, we covered random numbers; a number created using a large sample of integers, and a mathematical procedure that ensures that each number has an equal chance of occurring.
- Apart from this, we understood the major difference between pseudo-random numbers and truly random numbers; pseudo-random numbers are generated from an algorithm that has a constant seed value.
- Using some code examples, we understood various methods in Python to generate random integers, floats, and random arrays of various data types using the numpy.random() module in NumPy.