How to Create Object in Java?
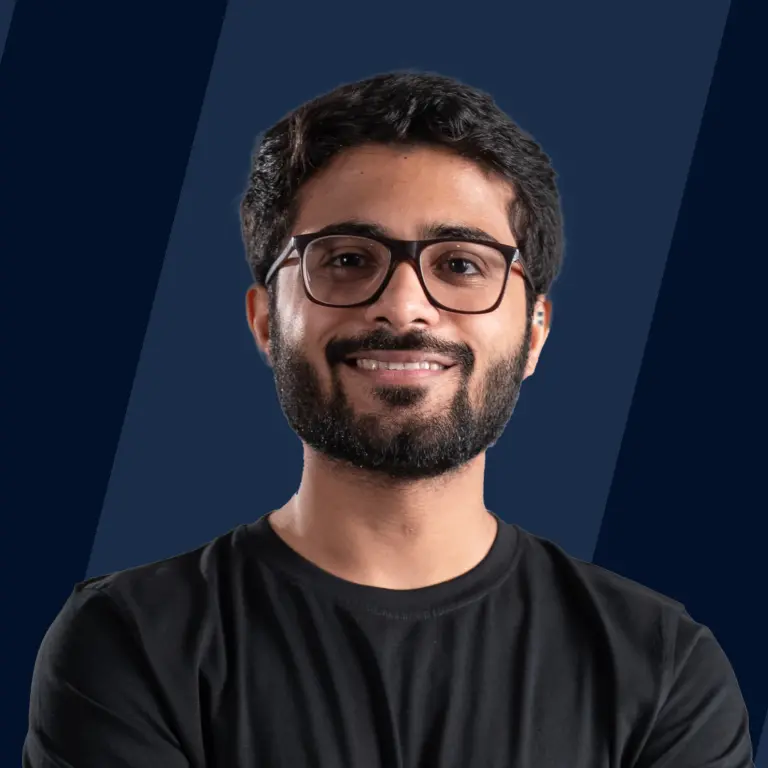
Class provides a blueprint for an object while object is the implementation of this class.
An object has three characteristics:
- State: Represents the value stored in the object. Eg. height, weight, etc.
- Behavior: Represents functionality performed by an object. Eg. running, sitting, etc.
- Identity: Each object has an unique ID and JVM uses this ID to identify an object.
This article focuses on how to create object in java.
Different ways to Create Objects in Java
There are six ways to create objects in Java:
- new keyword
- newInstance() method of Class class
- newInstance() method of Constructor class
- clone() method
- Deserialization in Java
- Factory Method Pattern
Create Object in Java Using new Keyword
This is the most common way regarding how to create object in java. The new keyword calls constructor of the class implicitly. The constructor can be both parameterized or non-parameterized. The new keyword allocates memory for the object and returns a reference to that object.
Output :
Explanation
- The object obj is created using the default no-arg constructor, setting the name attribute to "Ayush" by default.
- The object obj1 is created using the parameterized constructor, passing the argument "Priya", which assigns the value "Priya" to the name attribute.
Create Object in Java Using newInstance() method
To use this object creation method, you need to know the class name and ensure that the class has a public default constructor.
- Use Class.forName(<class_name>) to load the Java class.
- To create an object of the loaded class, utilize the newInstance() method, which returns an object of the class.
Output :
Explanation:
- Class.forName("ObjectCreate") loads the class "ObjectCreate" and assigns the reference to c. Then, (ObjectCreate) c.newInstance() creates a new object of the loaded class.
- We use a try-catch block to handle errors, such as when the class does not exist.
Create Object in Java Using newInstance() method of Constructor Class
The newInstance() method of Constructor class is similar to newInstance() method of class Class except that we can pass parameters for different parameterized constructor.
Code:
Output:
Explanation:
- In getConstructor() method, we specify the data types of the required arguments to find the desired constructor.
- In newInstance() method, we provide the necessary arguments.
- The newInstance() method returns a newly created object by invoking the appropriate constructor identified through the getConstructor() method.
Create Object in Java Using clone() Method
- The clone() method generates a new object with a distinct hash code and stores it in a separate memory location.
- clone() produces a replica of an existing object with identical attribute values.
- clone() generates an object of a class without invoking any class constructors.
- Modifying the values of one object does not affect the other.
Prerequisite: For utilizing this method, the class must implement the Cloneable interface and define its own clone() method.
Hence, the concept of cloning can also help in how to create object in java.
Output
Create Object in Java Using Deserialization Method
- An object is created by JVM when we serialize and then deserialize objects.
- No constructor is used to create an object in deserialization.
Prerequisite: The class whose object is to be created must implement the Serializable interface.
Output
Explanation:
- Initially, we serialize the serializeObject object, converting it into a byte sequence.
- The serialized object is then saved in a file named "file.ser" using the writeObject() method of the ObjectOutputStream class.
- The readObject() function is employed to read an object from the ObjectInputStream class, storing it in deserializeObj.
- Lastly, we print the data from the deserializeObj object.
Create Object in Java Using Factory Method Pattern
- To create an object using the Factory method pattern, we must define an abstract class or interface. Then define different classes which implement this interface.
- Factory Method Pattern is also known as Virtual Constructor.
- This is used when a class either does not know which subclasses will be required to create or the class wants its sub-classes specify the objects to be created.
Output
Explanation:
- Using the interface "Calculator" and the function Calculate(), we constructed a basic calculator.
- The calculator is capable of performing operations such as addition, subtraction, multiplication, etc.
- The Calculate() method is defined by distinct concrete classes that implement the "Calculator" interface.
- To generate objects of the classes that implement the interface, relying on user input, we define the "CalculatorFactory" class.
- Ultimately, by providing arguments like "ADD," "SUBS," and "MULTIPLY," we acquire instances of the concrete classes. The appropriate class instance is generated based on the argument provided.
Conclusion
- The new keyword is the most common way to create an object. new keyword can call both parameterized or non-parameterized constructors.
- The newInstance() method of class Class is used when the class name is known. Using this method, only no-arg constructor can be called to create objects.
- The newInstance() method of Constructor class is similar to newInstance() method of Class class execept it works with parameterized constructor too.
- The clone() method creates a new object by cloning the properties of an existing instance.
- JVM creates an object when we serialize and then deserialize an object.
- clone() method and deserialization do not use any constructor to create an object.
- Using factory methods, we can create objects of different classes based on certain parameters. In factory method pattern, a number of subclasses implement an interface or a abstract class.