Operator Precedence in Java
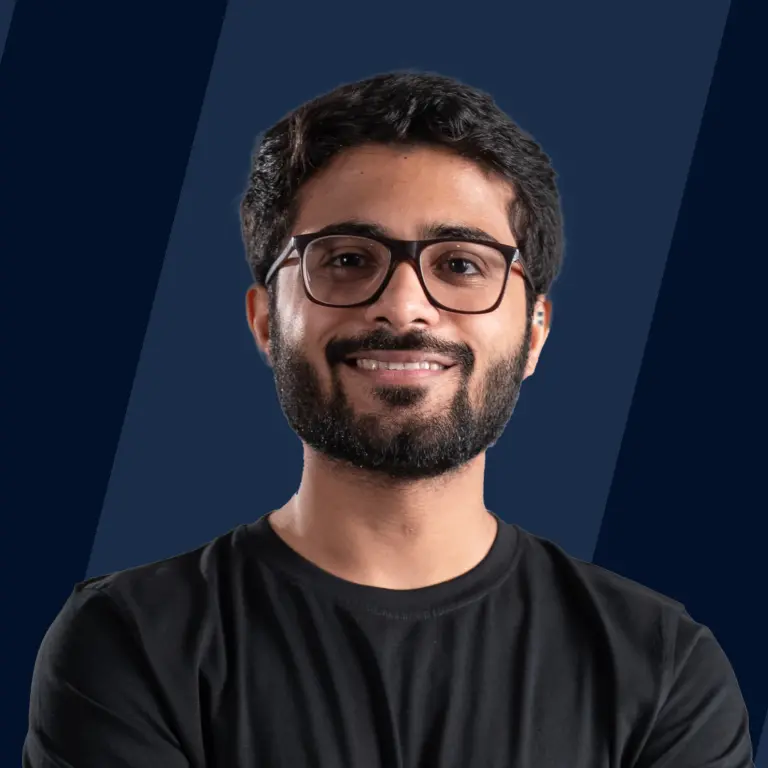
Overview
There exist many arithmetics and logical operators in Java like +, -, /, *, &, |, etc that can be applied in an expression. Operator precedence in java is a set of rules which tells the compiler the order in which the operators given in a particular expression are evaluated if there are multiple operators in a single expression.
Java Operator Precedence
During our primary schooling, we learned about the BODMAS rule which helps us to determine which operator to be solved first if many operators are there in a given equation.
Similarly, we have the operator precedence in java which is a set of rules that tells us the precedence of different operators, and as per the rule operator with higher precedence is evaluated first. For example in the expression x * y - z the * operator will be evaluated before the - operator, because the * operator has higher precedence than the - operator.
The precedence of each operator has been clearly defined which is also shown in the table given later in the article.
Note that it is always possible to modify the result of an expression by placing parentheses in the original expression. For example - 20 / 2 * 5 will evaluate to 50 but if we want to get 2 as result we can modify the expression to 20 / (2 * 5).
Operator Associativity - So we have seen that the operator with higher precedence is evaluated first. But if two operators in an expression are with the same precedence then the operator and operand are grouped according to their associativity.
The associativity of each operator has also been clearly defined in the table given in the next section. For example - 50 / 2 / 5 will be treated as (50 / 2) / 2 because the / operator is left-to-right associative.
Java Operator Precedence Table
The following table well defines the precedence of operators in java. Here the operators present at top of the table have higher precedence while those who are placed lower in the table have lower precedence.
Operator | Description | Precedence | Associativity |
---|---|---|---|
() [] . | Parentheses Array Subscript Member Function | 16 | Left to Right |
++ -- | Unary Post Increment Unary Post Decrement | 15 | Right to Left |
++ -- + - ! ~ | Unary Pre Increment Unary Pre Decrement Unary Addition Unary Subtraction Unary Logical Negation Unary Bitwise Complement | 14 | Right to Left |
() new | Cast Object Creation | 13 | Right to Left |
* / % | Multiplication Division Modulus | 12 | Left to Right |
+ - | Addition Subtraction | 11 | Left to Right |
>> << | Bitwise Right Shift Bitwise Left Shift | 10 | Left to Right |
< <= > >= instanceof | Less than Less than or equal Greater than Greater than or equal Type Comparison | 9 | Left to Right |
== != | Equal to Not equal to | 8 | Left to right |
& | Bitwise AND | 7 | Left to Right |
^ | Bitwise XOR | 6 | Left to Right |
| | Bitwise OR | 5 | Left to Right |
&& | Logical AND | 4 | Left to Right |
|| | Logical OR | 3 | Left to Right |
?: | Ternary Conditional | 2 | Right to Left |
= += -= *= /= %= | Assignment Addition Assignment Subtraction Assignment Multiplication Assignment Division Assignment Modulus Assignment | 1 | Right to Left |
Examples of Operator Precedence in Java
Example 1
Output
The precedence of * and / is greater than that of + operator hence they will be evaluated. Also, * and / are of the same precedence so they will be associated in left to right order.
Therefore -
is equivalent to
Example 2
Output
The precedence of ++ and -- is greater than that of -, and + operators. Hence,
is equivalent to
Example 3
Output
The precedence of ++ and -- is greater than that of + and << operators. So Hence, they will be evaluated first. Also, the precedence of << is greater than that of + operator. Therefore -
is equivalent to
which will be solved like -
Practice Questions
Question 1
Evaluate the following expression -
Answer - 29
Explanation
Since the precedence of * and / is greater than that of + and - operators.
is equivalent to
Question 2
Evaluate the following expression -
Answer - 26
Explanation
Since the precedence of * and / is greater than that of |, + and - **operators.
is equivalent to
.
Question 3
Evaluate the following expression -
Answer - 9
Explanation
Since parentheses have the highest precedence among all the operators, expressions written inside them will be evaluated first.
.
Question 4
Predict the output of the following code
Output
Explanation
The precedence of the assignment operator = is the least, and equal to the operator (==) is left to right-associative.
is equivalent to
true
Question 5
Predict the output of the following code
Output
Explanation
The above code results in a compile-time error because Java parses the statement as ++(++x) and the term ++x increases the value by 1 and evaluates it to 6 now we will have ++6 which is illegal because the pre-increment operator in java can only be applied to a variable, not to a value.
Conclusion
- Operator precedence in java determines the order in which the operators given in a particular expression are evaluated if there are multiple operators in a single expression.
- Operators with higher precedence are evaluated before the operator(s) with lower precedence.
- If two operators have the same level of precedence then the tie is broken by their associativity.