What is Operator Precedence in Python?
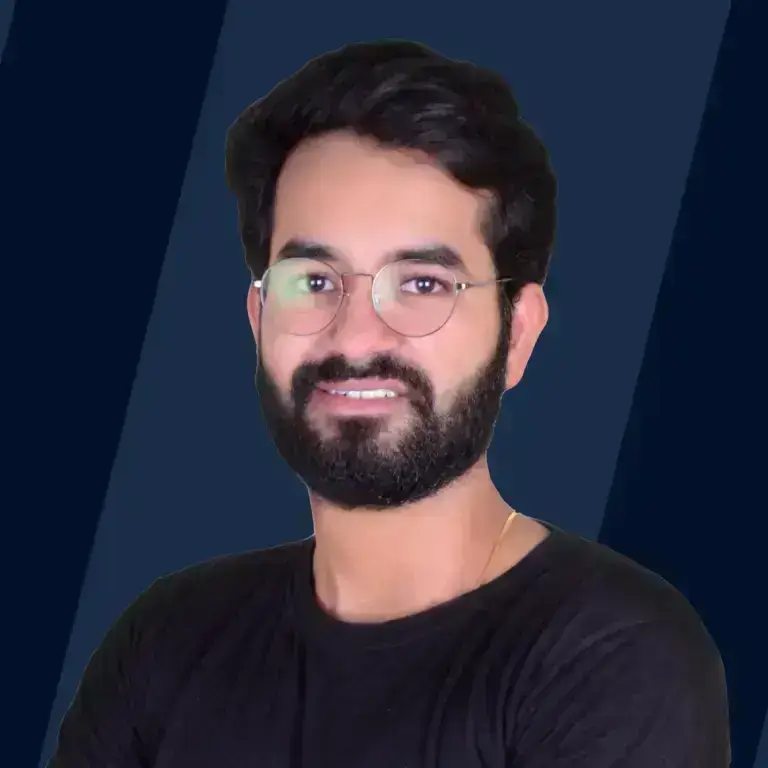
What is Operator Precedence in Python?
An expression in python consists of variables, operators, values, etc. When the Python interpreter encounters any expression containing several operations, all operators get evaluated according to an ordered hierarchy, called operator precedence.
Python Operators Precedence Table
Listed below is the table of operator precedence in python, increasing from top to bottom and decreasing from bottom to top. Operators in the same box have the same precedence.
Operator | Description |
---|---|
:= | Assignment expression |
lambda | Lambda expression |
if-else | Conditional expression |
or | Boolean OR |
and | Boolean AND |
not x | Boolean NOT |
in, not in, is, is not, <, <=, >, >=, !=, == | Comparisons, membership and identity operators |
| | Bitwise OR |
^ | Bitwise XOR |
& | Bitwise AND |
<<, >> | Left and right Shifts |
+, – | Addition and subtraction |
*, @, /, //, % | Multiplication, matrix multiplication, division, floor division, remainder |
+x, -x, ~x | Unary plus, Unary minus, bitwise NOT |
** | Exponentiation |
await x | Await expression |
x[index], x[index | Subscription, slicing, call, attribute reference |
() Parentheses | (Highest precedence) |
Python Operators Precedence Rule - PEMDAS
Operator precedence in python follows the PEMDAS rule for arithmetic expressions. The precedence of operators is listed below in a high to low manner.
Firstly, parantheses will be evaluated, then exponentiation and so on.
- P – Parentheses
- E – Exponentiation
- M – Multiplication
- D – Division
- A – Addition
- S – Subtraction
In the case of tie means, if two operators whose precedence is equal appear in the expression, then the associativity rule is followed.
Associativity Rule
All the operators, except exponentiation(**) follow the left to right associativity. It means the evaluation will proceed from left to right, while evaluating the expression.
Example-
In this case, the precedence of multiplication and division is equal, but further, they will be evaluated according to the left to right associativity.
Let's try to solve this expression by breaking it out and applying the precedence and associativity rule.
- Interpreter encounters the parenthesis (. Hence it will be evaluated first.
- Later there are four operators , , and .
- Precedence of and Precedence of .
- We can use the only operator precedence if all operators belong to different-different levels from the hierarchy table, which is not the case in our example.
- Associativity rule will be followed for operators with the same precedence.
- This expression will be evaluated from left to right, = = .
- Now, our expression has become .
- Left to right Associativiy rule will be followed again. So, our final value of expression will be, = = .
Comparison Operators
All comparison operations, such as <, >, ==, >=, <=, !=, is, and in, have the same priority, which is lower than arithmetic, shifting, and bitwise operations. Unlike in C, Python follows the conventional mathematical interpretation for expressions like a < b < c.
Comparisons in Python produce boolean values, either True or False.
Python allows chaining of comparisons, such as x < y <= z, which is equivalent to x < y and y <= z. It's important to note that while y is only evaluated once in the chain, z is not evaluated at all if x < y is found to be false.
Output:
Explanation: Before evaluating the expression a > b == c >= d, it's important to note that comparison operators in Python have equal precedence and are evaluated from left to right. The expression is transformed based on the comparison chaining rule:
The expression a > b == c >= d is equivalent to (a > b) and (b == c) and (c >= d).
- The expression is evaluated from left to right. The first comparison a > b is performed. Since a is 100 and b is 80, the result is True.
- Next, the second comparison b == c is evaluated. Both b and c have the value 80, so this comparison is also True.
- Finally, the last comparison c >= d is checked. Since c is 80 and d is 19, the condition holds True.
- Evaluating the chained comparisons in order, we have True and True and True, which results in the final output of True.
Note that comparisons, membership tests, and identity tests, all have the same precedence and have a left-to-right chaining feature.
Examples
Below are two examples to illustrate the operator precedence in python. See the explanation to get a good idea of how these things work internally.
1. Precedence of Operators
Output:
Explanation:
-
In the first expression, we have 4 operators: .
- Precedence of and Precedence of .
- The 'associativity rule' will be followed for operators with the same precedence.
- See the transformations shown below to get an understanding of how the entire evaluation will be followed.
- 12 * 3 % 34 / 8 = 36 % 34 / 8 = 2/8 = 0.25
- Now, we have only single operator; hence it would be evaluated simply, 10 + 0.25 = 10.25.
- Hence, the final value is: .
-
In the second expression, we have four operators: XOR, .
- Precedence of Precedence of Precedence of Precedence of .
- After Evaluation of , 48//24 = 2 our expression will become (4 ^ 2 << 3 + 2).
- After Evaluation of , 3+2 = 5 our expression will become (4 ^ 2 << 5).
- After Evaluation of , 2<<5 = 64 our expression will become (4 ^ 64).
- After the Evaluation of XOR, 4^64 = 68, our expression will become 68.
- Hence, the final value is: .
2. Associativity Rule of Operators
Output:
Explanation:
- Operator precedence in Python is the same for all operators of this example. It is simple to understand that the associativity rule will be followed for evaluation.
- Left to right associativity will be followed in the first two expressions.
- The point worth noticing here is that the exponentiation operator follows right to left associativity.
Conclusion
- The operator works on operands according to their specific order while evaluating the expression. This hierarchy is called operator precedence in python.
- In case of the same precedence, the left to right associativity is followed. But the exponentiation operator is an exception that follows right to left associativity.