Pair in Java
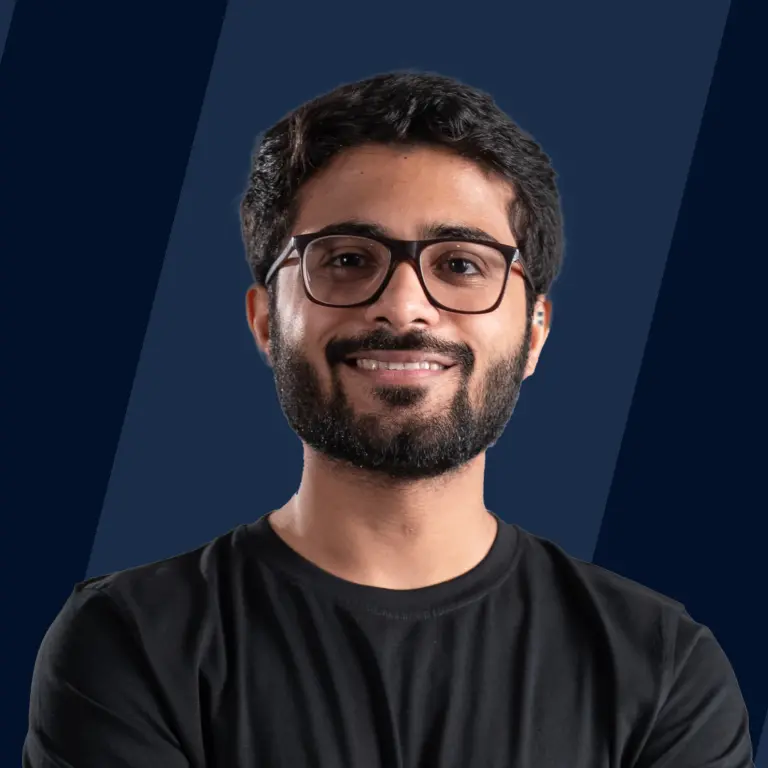
Overview
A Pair is a really useful data structure which is used to store two values together. Pairs are useful when we need to return two values from a method which are associated with each other such as a Student name and marks, a number and its square, etc.
Java supports the Pair data structure using the Pair class available in javafx.util package post Java 8. Pair class in Java stores paired data in a key-value pair combination, this combination is also known as tuple.
Introduction to Java Pair Class
Since Java 8, Java provides a Pair class to store a pair of values together. These two values can be of any data type independent of each other. For example, one value can be of String type, and the second one can be an Integer.
Now, let's see how we can use the in-built Pair class in Java.
1. Importing Pair class
For importing Pair class in Java we use:
2. Declaring a Pair object
Let us now see how to declare a Pair object in Java.
Syntax:
Example:
As a result of this statement, a Pair object of the type <Integer, String> is created. The constructor will take the value 7 and Hello and store them in the Pair object pr.
3. Accessing values
Using getKey() and getValue() methods we can access the two values stored in a Pair object.
- getKey(): gets the first value.
- getValue(): gets the second value
Now, let's see an example of implementing Pair class in Java.
Output:
Explanation:
As we can see, using the getKey() method, we can access the first value in the pair and using the getValue() method, we can access the second value. Both these methods are defined in the Pair class to access the first and second field values of a Pair object.
Note:
Here, <Key, Value> simply refers to a pair of values that are stored together.
Do not confuse it with the <Key, Value> used in a HashMap or HashTable.
Methods Provided by the javafx.util.Pair Class
Pair class in Java contains methods which make it easier to operate on Pair objects. Let's see some of these methods, their use cases and how to implement them in our Java programs.
1. boolean equals()
Two Pair objects are said to be equal if and only if the corresponding keys and values in both objects are equal. equals() performs a deep comparison which means only the basic values need to be equal.
Declaration:
Example:
Output:
2. String toString()
toString() method returns a String representation of a Pair object. toString() method in Pair class overrides the toString() method defined in Object class and returns a String view of the Pair object. The default name/value delimiter '=' is always used between the key and value of the Pair object.
Declaration:
Example:
Output:
3. getKey() Method
This method returns the key for the given specific pair in Java and is declared as:
Example:
Output:
4. getValue() Method
This method returns value for the given pair in Java and is declared as:
Example to understand the `getValue()`` method of Pair class in Java:
Output:
5. int hashCode()
This method generates a hash code for the given pair in Java, which is calculated using the key and value associated with the Pair object. This method overrides the hashCode() method declared in the Object class.
Declaration:
Example:
Output:
Note: The hashCode values for pair1 and pair2 are the same as the key, value are the same in both Pair objects.
Table for Pair Class Methods
Method | Return Type | Description |
---|---|---|
getKey() | K | Returns the key of the pair. |
getValue() | V | Returns the value of the pair. |
hashCode() | int | Generates a hash code for the given pair. Hashcode for equal Pair objects will be the same. |
toString() | String | String representation of the pair. |
equals(Object o) | boolean | If the object is not a Pair object or is null, equals() method returns false |
Types of Pair Classes
- MutablePair Class: Mutable classes allow the value to be altered at any time during our program. getters and setters methods can be used to alter and access the value of a given object after being defined.
MutablePair is defined in the Apache Commons Lang which is why before using it in our program we have to make sure the package has been added to the classpath.
We can add the below dependencies section of pom.xml if we're using Maven.
Maven is a build automation tool used primarily for Java projects.
How to import:
Creating MutablePair objects:
Update Values:
Accessing Elements:
Example:
Output
- ImmutablePair Class: In the ImmutablePair class we cannot change the value of an object once it’s defined, it remains constant and the setValue() method cannot be used to alter the constant value.
Since ImmutablePair is also defined in the Apache Commons Lang, we need to add the package and import the same way we did in MutablePair.
Let's see how we can create an ImmutablePair object:
Accessing Elements:
Now let's implement ImmutablePair class in Java program.
Output
Why do We Need Pair Class?
The following are a few cases when we need to use the Pair class:
-
The Pair class in Java is used for managing a pair of values which are somehow associated with each other, for example, a player and score, a student and marks, etc.
-
We can use a pair class in Java for returning a pair of values from a method.
-
Pair class is especially useful while performing operations on a Tree. When we perform recursion on a tree data structure, it becomes easy to return values to the tree nodes towards the top with certain computations performed on them like a max or min operation on the subtree of a tree node.
Custom Pair class in Java
We can create a Generic Pair class in Java with Java Generics. Generics means the data types will be dynamic or parameterized types. The data type of the member fields used inside a class will be provided in the class declaration.
Example:
Here we have created a Generic class which specifies the types of its member fields in its declaration.
Object Creation:
In a similar way, we can design a custom Pair class in Java using Java Generics. The Pair class will have its own public constructor for instantiation as we have seen above.
We can define two data fields in the Pair class i.e. first and second. The data types of first and second data fields will be defined in the Pair class declaration as shown in the code below.
We can define additional methods such as:
- getKey(): To retrieve the values of first data field.
- getValue(): To retrieve the values of second data field.
- toString(): To return a String presentation of a Pair object.
Example:
Output
Explanation:
- Here we have implemented a custom generic Pair class with standard methods implemented inside them.
- Then we have created a method getManOfTheMatch() to find out the maximum scorer and return the name of the player and score with it.
- As it is visible in the data, Dhoni is the maximum scorer with the total score of 99. Hence, its the output.
Conclusion
- Pair class in Java stores paired data in a key-value pair combination.
- We use the parameterized constructor provided by the javafx.util.Pair class to create a Pair object containing two associated values.
- There are various in-built methods provided by the Pair class such as getKey(), getValue(), equals(), toString() and hashCode() to perform various operations on Pair objects and retrieve their values.
- There are two types of pair classes, ImmutablePair and MutablePair classes.
- The Pair class in Java is used for managing key to value association, returning multiple values, and performing operations on Tree data structure.
- Using Java Generics, we can define a custom Pair class with necessary in-built methods such as given below. We can also define other methods as per certain specific requirements such as a method to find the maximum of two Pair objects.