Palindrome Program in C++
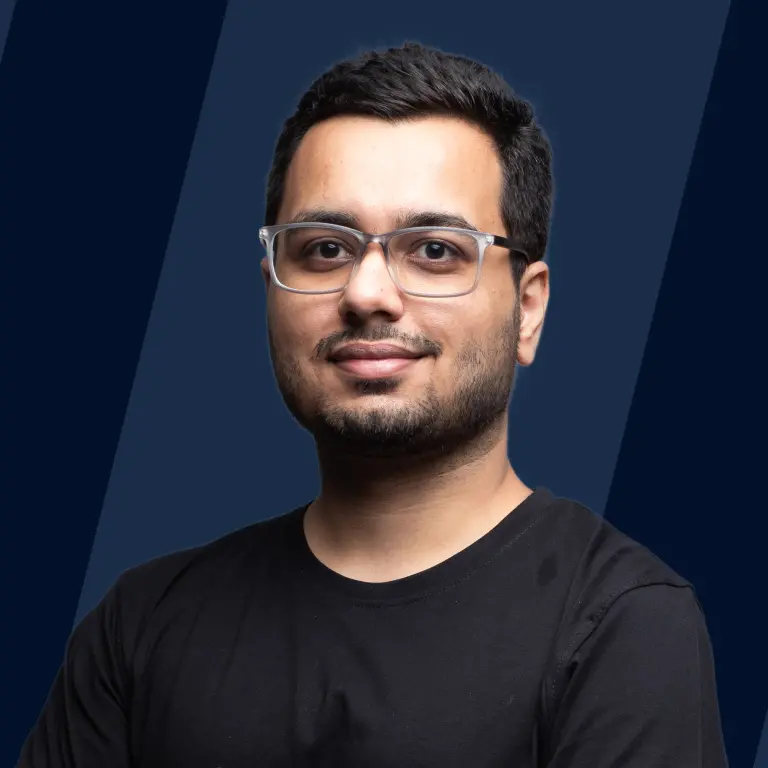
Introduction
A palindrome is any number that remains unchanged upon reversal, i.e., it reads the same backward as forward. Palindromes can be found and observed in nature and all around us in our day-to-day lives. Consider the numbers 1331 or 515. Both of these numbers can be read the same way backward or forward, which is why they are known as palindromes.
Let's look at the algorithm to check whether a number is a palindrome or not, along with the Palindrome program in C++.
C++ Palindrome Number Algorithm
Now, let's take a look at the generic algorithm to check whether a number is palindrome or not:
- Step_1: Take a number as input from the user to check whether it is a palindrome or not.
- Step_2: Calculate the reverse of the given number and store it in another variable.
- Step_3: Check whether the reversed number generated in Step_2 is the same as the input number. If yes, print "Palindrome" and go to Step_5, else go to Step_4
- Step_4: Print "Not a Palindrome."
- Step_5: END
In the above algorithm, the most important step is Step_2, i.e., to calculate the reverse of the given input number as it determines the time complexity of the whole algorithm. Hence, let's focus on this step and find out how we can calculate the reverse of a number:
We can find the reverse of a number by splitting the number into individual digits and then combining the digits in reverse order. This can be achieved by using an iterative method in which we extract the digits from the number and store them in reverse order to check the palindrome.
Let's look at this iterative method's algorithm:
- Step_1: Take the input number n from the user.
- Step_2: Make a copy of the input number by assigning it to a temporary variable, i.e., (temp = n).
- Step_3: Initialize a new variable called reverse to store the reverse of the input number and digit to store the digits of the number.
- Step_4: Start a while loop.
- Step_5: Extract the last digit from the number by using the modulus operator (n % 10).
- Step_6: Add this digit to the reverse variable after multiplying the past value of the reverse variable by 10. (reverse = reverse * 10 + digit)
- Step_7: Add a decrement statement that updates the variable n such that the next digit from the left-hand side can be extracted. (n = n / 10)
- Step_7: Repeat the steps from Step_5 to Step_7 until n becomes equal to 0
- Step_8: END
The above algorithm uses three variables, namely (n, temp, reverse), to find the reverse of a number. For the input number 1234, let's trace the values of these three variables in every iteration of the while loop to understand the working of the iterative method for checking palindrome in C++.
Initial Value:
- n = 1234
- reverse = 0
- temp = 1234
Iteration 1:
- n = 123
- reverse = 4
- temp = 1234
Iteration 2:
- n = 12
- reverse = 43
- temp = 1234
Iteration 3:
- n = 1
- reverse = 432
- temp = 1234
Iteration 4:
- n = 0
- reverse = 4321
- temp = 1234
Here, we can observe that after every iteration, a digit is removed from the input number and is stored in the reverse variable. The loop terminates once all the digits are stored in reverse order in the reverse variable. This reversed number is compared with the number stored in the temp variable to check whether the input number is a palindrome or not.
Hence, upon implementing the above-described algorithm, the reverse variable will contain the reverse of the input number, whereas the temp variable will hold the original input number. Let's look at how we can implement this iterative method of checking palindrome numbers in C++.
Using reverse() Function in STL
The simplest approach is to use the inbuilt reverse() function in the STL.
Algorithm :
- Step 1: Take the input string S.
- Step 2: Create a copy of the input string by assigning it to another string variable, say P.
- Step 3: Reverse the string P using the reverse() function from the STL.
- Step 4: Compare the original string S with the reversed string P.
- Step 5: If S is equal to P, print "Yes." Otherwise, print "No."
Code:
Output :
Complexity Analysis
Time Complexity: O(N) Space Complexity: O(N)
Where N represents the total length of the string S.
Checking Palindrome Number in C++ by Traversing the String
Algorithm:
-
Step 1: Start by taking an input integer, num.
-
Step 2: Convert the integer to a string, using the to_string function.
-
Step 3: Initialize two variables, left and right, to 0 and the length of numStr minus 1, respectively.
-
Step 4: Start a loop while left is less than right:
- Check if numStr[left] is not equal to numStr[right.
- If they are not equal, return "No" as the number is not a palindrome.
- Increment left by 1 and decrement right by 1.
-
Step 5: If the loop completes without finding any unequal characters, return "Yes" as the number is a palindrome.
-
Step 6: END
Code :
Output :
Complexity Analysis
Time Complexity: O(N) Space Complexity: O(N)
Where N represents the total number of digits in the number.
Check Palindrome Number using Function
For convenience, we can also create a user-defined function that takes the number as a parameter and checks whether the input number is a palindrome. The user-defined program will contain the iterative method of reversing a number to check whether the input number is a palindrome or not.
This can be implemented in C++ using the below code:
Output :
In this palindrome program in C++, we are using the iterative method inside a user-defined function checkPalindrome() that takes an integer as a parameter and checks whether the given number is a palindrome or not.
Conclusion
- A palindrome is any number that remains unchanged upon reversal, i.e., it reads the same backward as forward.
- To check if a number is a palindrome, you must find it's reverse first.
- The palindrome program in C++ is implemented using the Iterative method or User-defined functions.
- In the iterative method, we reverse the number by iteratively extracting the digits from the number and storing them in reverse order.