Passing Array to Function in C++
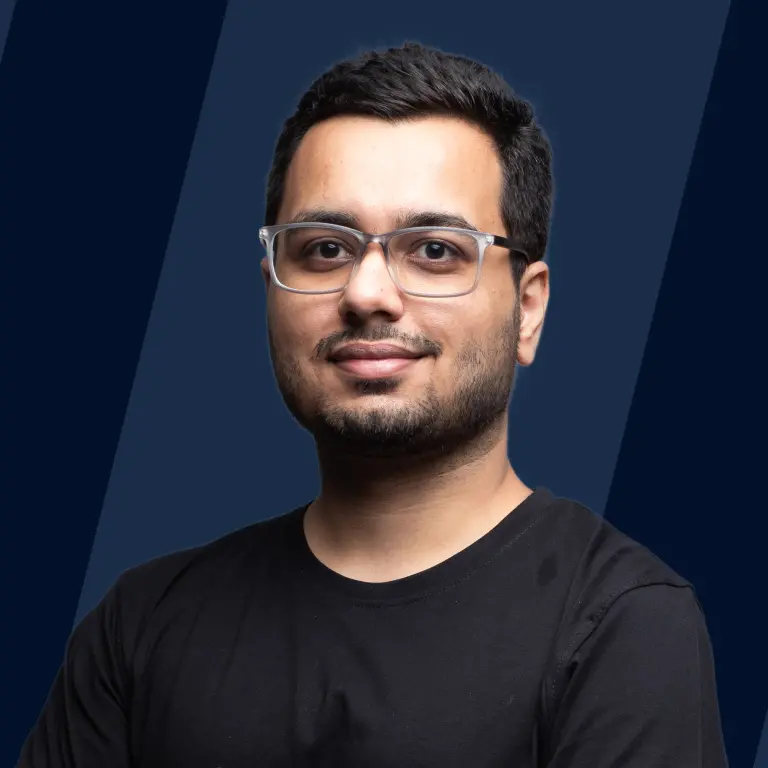
An array is the most important, easiest, and widely used data structure in programming languages.
As arrays are the most widely used and important data structures we should have a clear idea to process them and pass them to other functions to solve various use cases.
In this article, We will see how to pass an array to a function
Passing Array to Function in C/C++
Just like normal variables, Arrays in c++ can also be passed to a function as an argument, but in C/C++ whenever we pass an array as a function argument then it is always treated as a pointer by a function. Pointers not only have the capability of storing the address of a single variable but they can also store the address of cells of an array.
Note: The array name is treated as the address to the first element of an array, hence we can use pointers to access the elements of arrays.
There are mainly 3 following ways to pass an array to a function in C/C++:
- Passing Individual Array Elements
- Passing Arrays to Functions
- Passing multidimensional arrays to functions
Passing Individual Array Elements
In c++ Passing array elements to a function is similar to passing variables to a function.
Passing Arrays to Functions
Passing arrays to functions in C++ can be done by passing the array as a parameter. When you pass an array to a function, you are actually passing a pointer to the first element of the array. Here's how you can pass arrays to functions in C++:
Passing multidimensional arrays to functions
Just like single-dimensional arrays, we can also pass multi-dimensional arrays to the function as arguments but we have to take care of the indexes at the time of dealing with multi-dimensional arrays.
The below simple program shows how to pass and handle the multi-dimensional array in C/C++.
Methods to pass an array
In C/C++ whenever we pass an array as a function argument, then it is always treated as a pointer by the function. While programming, Functions can be invoked mostly in two ways: Call By Value and Call By Reference ( pass array by reference ) based on the use-cases.
1. Call By Reference:
In c++ we can pass array by reference. It copies the address of an argument into the formal parameter of that function. In this method, the address is used to access the actual argument used in the function call. It means that changes made in the parameter will alter the passing argument.
2. Call By Value:
It copies the value of an argument into the formal parameter of that function. Hence, changes made to the parameter of the main function do not affect the original values that are passed as arguments.
In order to pass an array as call by value, we have to wrap the array inside a structure and have to assign the values to that array using an object of that structure. This will help us create a new copy of the array that we are passing as an argument to a function.
Let's understand this with a simple example.
- Create a structure that will act as a wrapper and will declare an array inside it.
- Assign the values to the array declared inside a structure using the object of a structure.
- Pass the address of the object to the function call so as to pass the complete array to a function.
Returning Array From the Function
We know that a function can not return more than one variable in C/C++. In some problems, we may need to return multiple values from a function, in such cases, an array can be returned from the function. To return an array from a function we have to return the pointer of a data type of array. Let's try to implement this with a simple sorting example.
The function call (sort) accepts an array as a function argument and will return an array with the sorted values.
Conclusion
- An array is a collection of homogeneous elements stored in a contiguous memory location and the most widely used data structure in programming.
- In C/C++ an array when passed as a function argument is always treated as a pointer by a function.
- Ways to pass an array to a function in C/C++ are Formal parameters as pointers, Formal parameters as sized arrays, and Formal parameters as unsized arrays.
- It is possible to return an array from a function by changing the return type of the function to the pointer of the data type of the array.
FAQs
Q. Can I modify the original array when I pass it to a function?
A. Yes, for passing array in c++ you can modify the original array when you pass it to a function. In C++, when you pass an array to a function, you are actually passing a pointer to the first element of the array. This means that any modifications you make to the array inside the function will affect the original array outside of the function.
However, if you want to prevent modifications to the original array, you can pass it as a constant pointer or use const qualifiers in the function's parameter list to indicate that the function should not modify the array.
Q. How do I pass a multi-dimensional array to a function?
A. To pass a multi-dimensional array to a function in C++, you need to specify the array dimensions in the function parameter list. For example, if you have a 2D array, you would declare the function parameter as a 2D array, and you need to specify the size of both dimensions. Here's an example:
In this case, int arr[][3] represents a 2D array with 3 columns, and you also pass the number of rows (rows) and columns (cols) to the function.
Q. Can I return an array from a function in C++?
A. In C++, you cannot return a raw array from a function directly. Instead, you can return a pointer to an array or use containers like std::vector or std::array to return a collection of values from a function. Containers provide dynamic sizing and better memory management. If you need to return an array, you should allocate memory dynamically, populate the array, and return a pointer to it. Be cautious with memory management to avoid memory leaks. Here's an example of returning a dynamically allocated array:
Remember to release the dynamically allocated memory using delete[] when you're done with the returned array to prevent memory leaks.