Pointer To Object In C++
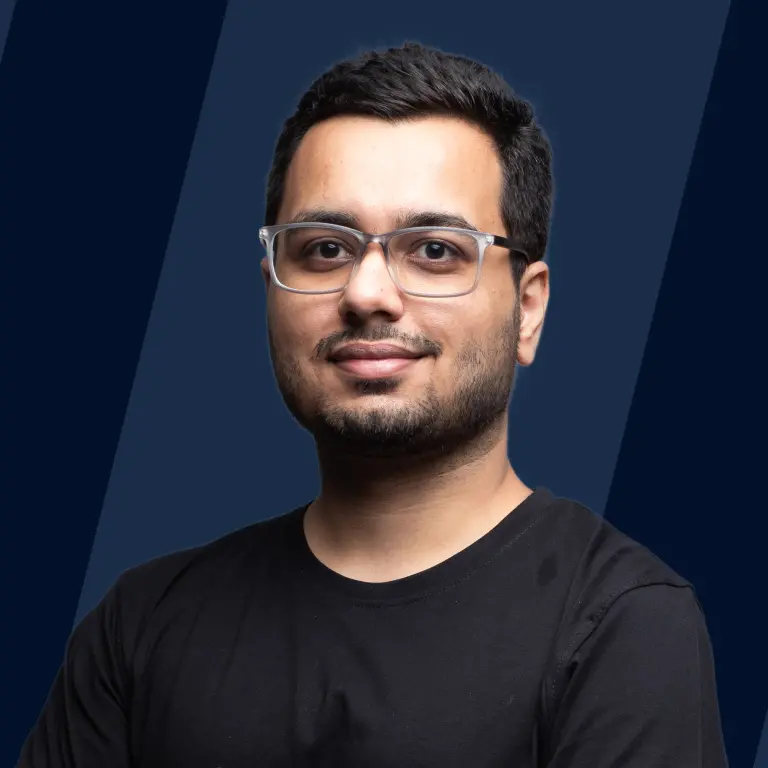
A pointer is a variable that stores the memory address of another variable (or object) as its value. A pointer aims to point to a data type which may be int, character, double, etc.
Pointers to objects aim to make a pointer that can access the object, not the variables. Pointer to object in C++ refers to accessing an object.
There are two approaches by which you can access an object. One is directly and the other is by using a pointer to an object in C++.
- A pointer to an object in C++ is used to store the address of an object. For creating a pointer to an object in C++, we use the following syntax:
For storing the address of an object into a pointer in c++, we use the following syntax:
The above syntax can be used to store the address in the pointer to the object. After storing the address in the pointer to the object, the member function can be called using the pointer to the object with the help of an arrow operator.
Examples
Now, let us see some examples and understand the pointer to the object in c++ in a better way.
Example 1. In the below example, a simple class named My_Class is created. An object of the class is defined as named object. Here a pointer is also defined named p. In the program given below program, it is shown how can we access the object directly and how can we use the pointer to access the object directly.
Output:
Explanation: The address of the object named object is accessed by using the address of (&) operator. Whenever there is any increment or decrement in the pointer, it changes in such a way that the pointer will aim at the next element of that particular base class. Also, the same thing happens whenever there is any change in a pointer to an object. The change here refers to any increment or decrement in the pointer.
For showing this, there are some modifications done in the above program so that the object will be a two-dimensional array of type My_Class. Now, the pointer p will be once incremented and then decremented for accessing the two elements in the array.
Output:
Example 2. In the below example, we used a pointer to an object and an arrow operator.
Output:
Explanation: A class name Complex is created in the above program. It contains two primitive data members. One is real and the other is imaginary. There are also two member functions inside the class. One is get_data and the other one is set_data. The work of function set_data is that it will take two parameters and assign the value of these parameters to the data members that are- real and imaginary. The work of function get_data is that it will print the values of data members that are real and imaginary.
The main program consists of an object that is created dynamically with the help of the new keyword. The address of this object is assigned to the pointer named ptr. Then the get_data is called. This calling of a member function is done by the pointer using the arrow -> operator. The set_data is then called by using a pointer with the help of the arrow -> operator.
Pointer ptr is used to call the member function get_data and set_data and it will print the values 1, 54 and 1, 4 respectively. Here we used an arrow operator instead of a dot (.) operator but it gives the same expected output.
Definition
let us have a brief grasp of the basic concepts of pointers that will help to understand pointer to object in c++ in a better way and that will be a prerequisite for a pointer to object in c++.
Pointers
In simple terms, a pointer is a variable that stores the memory address of another variable (or object) as its value. A pointer aims to point to a data type which may be int, character, double, etc. But a pointer always points to a data type of the same type. Pointers are created by using the * operator.
- Types of pointers in c++ There are majorly four types of pointers in C++:
- Null Pointer: As the name suggests, a null pointer is a type of pointer which represents nothing.
- Void Pointer: A void Pointer is a type of pointer which does not point to any data type. We can say that it is a general-purpose pointer.
- Wild Pointer: Wild pointers are similar to normal pointers but one thing that makes them different is they are only declared but not initialized. Since they are only initialized they point to unallocated memory.
- Dangling Pointer: A dangling pointer is a type of pointer that comes when we deallocate the object but forgets to modify the value of the pointer.
Objects
Objects in c++ are defined as the instance of a class. It is also referred to as a piece of code that represents the member of its class of variables of its class. An object can access all the members of its class. So, we can also say a class is a collection of many objects inside it. An object can be created many times but a class can be defined once only.
- Syntax for objects in c++: The syntax of defining an object in c++ is done as given below.
When we create an object, all the members of the class are defined inside that particular class. These objects can access the data members with the help of a dot(.) operator.
What are the Advantages of using Pointer to Objects in C++?
- Pointers can be used to store the memory address of a variable. The syntax for this is as follows.
Example In the example given below, ptr stores the address of an integer variable. Using this pointer we can access the memory address of that particular variable int.
- Using a pointer to an object in c++, we can save memory space and we can directly manipulate the memory.
- Execution becomes faster when we use pointers because a pointer manipulates the memory address of data rather than accessing the data.
- Pointer to object in c++ is used with data structures like two-dimensional arrays and multi-dimensional arrays.
- Pointers to objects can be used for File Handling.
- When we declare a pointer to the base class, then an object of the derived class can be accessed by the base class.
- Pointer to object in c++ can be used to dynamically allocate the memory.
Learn More About Pointers in C++
To learn more about pointers in C++ Read our Article.
Conclusion
- Pointer to object in c++ is defined as the pointer that is used for accessing objects.
- A pointer is a variable that stores the memory address of another variable.
- The types of the pointer are Null pointer, Void pointer, Wild pointer, and Dangling pointer.
- An object is defined as the instance of a class.
- These objects can access the data members with the help of a dot(.) operator.
- Pointer to object in c++ helps to execute a program faster.
- Pointer to object can be used for File Handling.