How to Print Array in Java
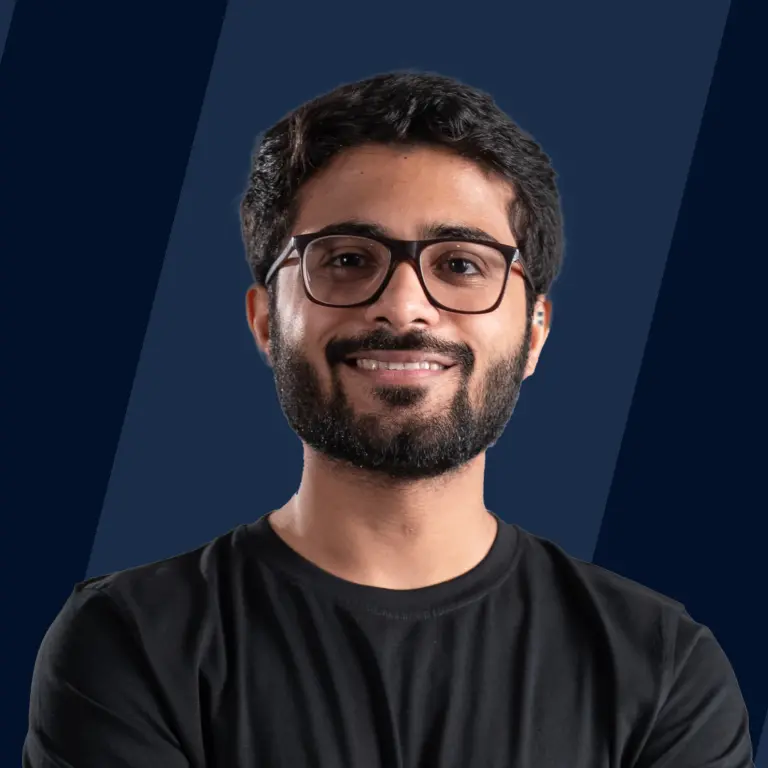
In Java, the array is an important data structure that is used to store similar types of data in contiguous memory locations. In this article, we'll look at all the different ways how to print an array in Java using loops and other methods such as:
- Java for loop
- Java for-each loop
- Java Arrays.toString() method
- Java Arrays.deepToString() method
- Java Arrays.asList() method
- Java Iterator Interface
- Java Stream API
Print an Array in Java Using for Loop
Let's learn how to print array in Java using for loop:
Java for loops can be used to iterate over an array until a particular condition is satisfied or till we get to the end of the array. It is one of the simplest methods to traverse over and print an array in Java. In the below-provided example, we are initializing an array of size 3 and using for loop to print all of its elements. Code:
Output:
Time and Space complexity:
For an array of size n, we will have to iterate the array n times and we are also not using any extra space.
Time complexity = O(n) Space complexity =O(1)
Print an Array in Java Using for-each Loop
Java for-each loop is another simpler and more elegant way to traverse over and print an array in Java. It works in a similar way to a for loop, just have a slightly different syntax. In a for-each loop, unlike a simple for loop, instead of creating a loop counter variable, we create a variable of the same type as that of the array values followed by name of the array. This variable holds one element of our array at a time during each iteration until it finally reaches the end of the array.
Let's learn how to print array in Java using for-each loop:
Syntax:
Code:
Output:
Time and Space complexity:
Time complexity = O(n) Space complexity =O(1)
Print an Array in Java Using Arrays.toString() Method
Array.toString() is a Java static method that is part of java.util package which contains several methods that can be used to manipulate the array. Array.toString() is used to convert the array that is passed to it as an argument to its string representation and instead of printing the array by looping over each element, we can print this string representation instead.
Let's learn how to print array in Java using Arrays.toString() method:
Syntax:
You can pass an array of any primitive data type to the Array.toString() method and it returns a string representation of that array which contains a list with array elements.
Code:
Output:
In this way, we can print an array with a single line of code without looping over the array.
Time and Space complexity:
If we look at the source code for the Array.toStirng() method, we will see that it appends each of our array elements to a string builder using a for loop and returns the final string. Also, if the size of our array is n, our new string will also be of size n.
It will also take O(n) time to print the string representation of our array.
Time Complexity: O(n) Space Complexity: O(n)
Print an Array in Java Using Arrays.deepToString() Method
Let's learn how to print array in Java using Arrays.deepToString() Method:
The Arrays.deepToString() method in Java is particularly useful for printing multidimensional arrays.
Syntax:
We will look at the above example only this time we will use the Arrays.deepToString() method.
Code:
Output:
This is how you can print a multidimensional array using the Arrays.deepToString() method.
Time and Space complexity:
Similar to the Array.toString() method,
Time Complexity: O(n) Space Complexity: O(n)
Print an Array in Java Using Arrays.asList() Method
Let's learn how to print array in java using Arrays.asList() Method:
Arrays.asList() method returns a fixed-size list backed by the specified array and then that ArrayList can be directly printed.
This method also accepts an array as an argument and then returns the list view of that array.
Code:
Output:
Time and Space complexity:
Arrays.asList() method doesn't copy array elements into a new list so,
Time Complexity: O(1) Space Complexity: O(1)
Print an Array in Java Using Iterator Interface
Let's learn how to print array in Java using Iterator Interface:
An iterator object can be created by calling the iterator() method which is part of the Collections interface.
Java iterator interface allows you to traverse over a java collection so we'll first have to convert our array to a list using the Arrays.asList() method as discussed before and then using the iterator, we will be able to traverse through it.
Code:
Java iterator interface contains 4 methods
- hasNext(): If there are still elements in the collection, this method returns true.
- next(): This function yields the traversal's subsequent element. It throws NoSuchElementException if there are no more elements to traverse.
- remove(): This function eliminates the final member that the iterator returns after navigating the underlying collection.
- forEachRemaining(): This function requires an input. An action is accepted as a parameter. An action is nothing more than a task that needs to be completed. The method does not have a return type. Until all of the components are eaten or the action throws an exception, this method does the particularized operation to every left component in the collection.
Output:
Time and Space complexity:
Time Complexity: O(n) Space Complexity:O(1)
Print an Array in Java Using Stream API
Let's learn how to print array in Java using Stream API:
This Java program initializes an array of strings and utilizes the Stream API to iterate through the elements. It invokes the forEach() method to print each element of the array to the console using method reference syntax.
Code:
Output:
Time and Space complexity:
Time Complexity: O(n) Space Complexity:O(1)
Conclusion
- Traditional for loops and for-each loops offer simple and effective ways to print array in Java.
- The Arrays.toString() method provides a concise solution for printing single-dimensional arrays.
- For multidimensional arrays, the Arrays.deepToString() method efficiently prints nested arrays.
- Arrays.asList() method converts arrays to lists, allowing for direct printing or further manipulation using collection methods.