What are Private, Public, and Protected in C++?
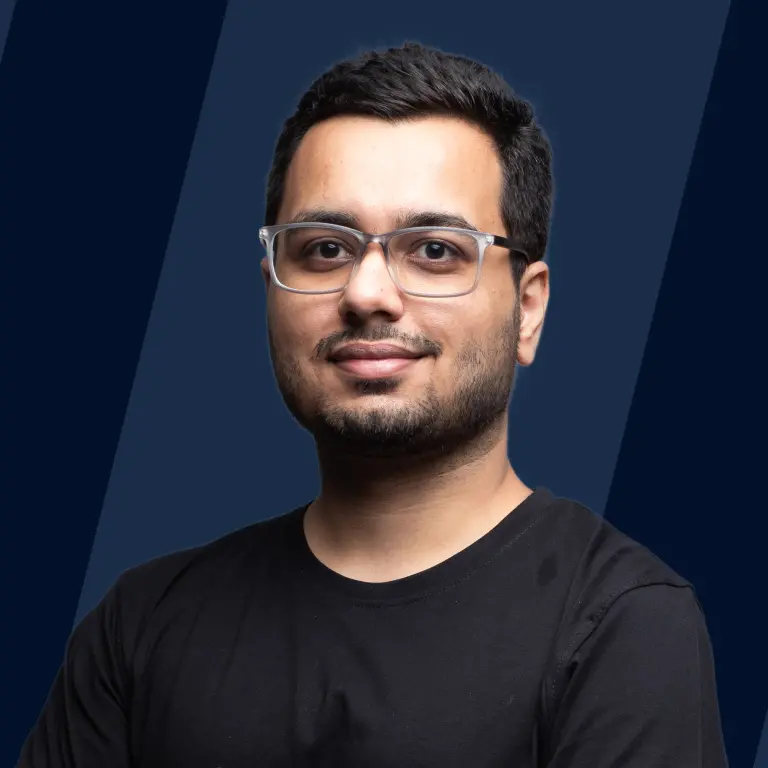
Data hiding is one of the important features of object-oriented programming in C++, which allows us to hide internal object details i.e. the data members, and prevents the functions of a program from directly accessing the internal representation, data members, and member functions of a class.
The access restriction to the class member functions is specified by access modifiers. Access modifiers are also known as visibility modes in C++, and private, public, and protected are all types of visibility modes. We will look at each of them in detail in the upcoming sections of this article.
Visibility modes allow us to know how the base class will be inherited by the derived class and which members of the base class will be acquired and will be accessible by the derived class. The name visibility mode or access specifier is given as they are used to define the accessibility and the visibility level of class members.
Access specifiers are mainly used in inheritance when a member function in the base class can be used by the objects of the derived class. The general syntax of using an access specifier while deriving a child class from the base class is:
In the above code, we have a base class Base and a derived class Derived, and the access_specifier which tells us which members of the base class will be acquired and will be accessible by the derived class.
A class in C++ can have multiple public, protected, or private sections. We can see in the code below how we can use visibility modes in a class:
In the above code, we have a base class base, having multiple sections divided by the access specifiers.
NOTE: If no visibility mode is specified then, by default the private mode is considered.
The Private Members
In the private visibility mode, when we inherit a child class from the parent class, then all the members (public, private and protected members) of the base class will become private in the derived class.
The access of these members outside the derived class is restricted and they can only be accessed by the member functions of the derived class or by the friend functions.
The following block diagram can show how data members of the base class are inherited when the derived class access mode is private.
As you can see from the image above, the private variable a is not accessible in the derived class because it is of the private type inside the base class, so it is only accessible by the member functions within the base class itself. The public variable c and the protected variable b of the base class will become private in the derived class and they will only be accessed by the member functions of the derived class.
We can understand when there is a need for which access specifier with the help of a very basic real-life example, suppose that on any social media app, you want to update the status but you have some requirements and restrictions on who can see this status on the Internet. So, if you want that no one should see this status or it should be visible “only for me”, then that means that you are following the concept of "Private access specifier".
Example
Let's see an example to understand the concept of private visibility mode and the restrictions provided by it:
In the above code, as the visibility mode is private, so, all the members of the base class have become private in the derived class. The error is thrown when the object of the derived class tries to access these members outside the derived class.
Now, let's take one more C++ program to demonstrate the working of the private access specifier when the object of the base class tries to access the private data member x outside the class.
The output of the above program will be compile time error as we are not allowed to access the private data of members of a class directly from outside the class.
However, we can access the private data members of a base class indirectly by calling the public member functions of the base class.
Accessibility
The accessibility of the private mode can be summarized as follows:
Accessibility | Private mode |
---|---|
Same class | Yes |
Derived class | No |
Other class | No |
Anywhere in the program | No |
The members defined under the private access specifier are accessible only in the same class, and they are not accessible in the derived class or any other class of the program.
The Public Members
In public visibility mode, when we inherit a child class from the parent class, then the public, private, and protected members of the base class remain public, private, and protected members respectively in the derived class as well. The public visibility mode retains the accessibility of all the members of the parent class.
The public members of the parent are accessible by the child's class and all other classes. The protected members of the base class are accessible only inside the derived class and its inherited classes. However, the private members are not accessible to the child class.
The following block diagram can show how data members of the base class are inherited when the derived class access mode is public.
As you can see from the image above, the private variable a is not accessible in the derived class because it is of the private type, so it is only accessible by the member functions of the base class. The public variable a will remain public and will be accessible to the child's class and all others. The protected variable b of the base class will remain protected in the derived class and will be accessible only inside the derived class and its inherited classes.
Coming to the real-life example of updating the status on any social media app, we have taken above, suppose you want that your status should be visible to anyone on the internet, then, that means that you are following the concept of "Public access specifier".
Example
Let's see an example to understand the concept of public visibility mode and the restrictions provided by it:
In the above code, the protected variable x2 will be inherited from the Parent class and will be accessible in the Child class and its inherited classes, similarly, the public variable x3 will be inherited from the Parent class and will be accessible in the Child class and other classes, but the private variable x1 will not be accessible in the Child class.
Now, let's take one more C++ program to demonstrate the working of public access specifier when the object of the base class tries to access the public data member x outside the class in the main() function.
The output of the above program would be:
In the above program, the data member x is declared as public and so it could be accessed in any class and also from outside the class.
Accessibility
The accessibility of the public mode can be summarized as follows:
Accessibility | Public mode |
---|---|
Same class | Yes |
Derived class | Yes |
Other class | Yes |
Anywhere in the program | Yes |
The members defined under the public access specifier are accessible everywhere, in the same class, in derived classes, in any other class, or even outside the class.
The Protected Members
In the protected visibility mode, when we inherit a child class from the parent class, then all the members of the base class will become the protected members of the derived class.
Because of this, these members are now only accessible by the derived class and its member functions. These members can also be inherited and will be accessible to the inherited subclasses of this derived class.
The following block diagram can show how data members of the base class are inherited when the derived class access mode is protected.
As you can see from the image above, the private variable a is not accessible in the derived class because it is of the private type, so it is only accessible by the member functions of the base class. The public variable a will become protected in the derived class and will be accessible only by the inherited subclasses of this derived class. The protected variable b of the base class will remain protected in the derived class and will be accessible only inside the derived class and its inherited classes.
Again, coming to the real-life example of updating the status on any social media app, we have taken above, suppose, now, if you want that your status should only be visible to your friends and your friends of friends, then, that means that you are following the concept of "Protected access specifier".
Example
Let's see an example to understand the concept of protected visibility mode and the restrictions provided by it:
In the above code, as the visibility mode is protected, the protected and the public members of the Parent class will become the protected members of the Child class.
The protected variable x2 will be inherited from the Parent class and will be accessible in the Child class, similarly, the public variable x3 will be inherited from the parent class as the protected member and will be accessible in the Child class, but the private variable x1 will not be accessible in the Child class.
Now, let's take one more C++ program to demonstrate the working of protected access specifiers when the object of the base class tries to access the protected data member x outside the class in the main() function.
The output of the above program would be:
In the above program, the data member x is declared as protected and so it could be accessed by any subclass (derived class) of the base class.
Accessibility
The accessibility of the protected mode can be summarized as follows:
Accessibility | Protected mode |
---|---|
Same class | Yes |
Derived class | Yes |
Inherited subclasses of the derived class | Yes |
Other class | No |
Anywhere in the program | No |
The members defined under the protected access specifier are accessible inside the derived class and its inherited classes.
Difference between Private, Public, and Protected in C++
Private | Public | Protected |
---|---|---|
The class members declared as private can be accessed only by the functions inside the base class. | The class members declared as public can be accessed from anywhere. | The class members declared as protected can be accessed in the base class and its derived classes. |
Private members are declared using the keyword private followed by a colon (:) character. | Public members are declared using the keyword public followed by a colon (:) character. | Protected members are declared using the keyword protected followed by a colon (:) character. |
Private members are not inherited in class. | Public members can be inherited in class. | Protected members can be inherited in class. |
It provides high security for its data members. | It doesn't provide any kind of security for its data. | It provides less security than private access specifier. |
Private members can be accessed with the help of a friend function. | Public members can be accessed with or without the help of a friend function. | Protected members cannot be accessed through the friend function, they are inaccessible outside the class but they can be accessed by any subclass(derived class) of that class. |
We can summarize the understanding of the above three visibility modes in C++:
Learn More About
Conclusion
- Access specifiers have been used to determine the availability of the class members (data members and member functions) beyond that class.
- In inheritance, it is important to know when a member function in the base class can be used by the objects of the derived class, this is known as accessibility and access specifiers are used to determine this.
- The private, public, and protected are all the types of access specifiers in C++. In case, no visibility mode is mentioned or specified, then, by default, the private mode is considered.
- When the members of the class are declared as public, then they are accessible from anywhere.
- When the members in the class are declared as protected, then, they are accessible in the base class and the derived classes.
- When the members in the class are declared as private, then, they are only accessible by the members of the base class and by the friend functions.
- Private access specifier provides more security to its data members as compared to the public and protected access specifier.
- It is considered good practice to declare your class attributes as private (as often as you can), as it will help in increasing data security.
- Access modifiers are mainly used for data hiding and encapsulation as they can help us to control what part of a program can access the members of a class. So that misuse of data can be prevented.