Python Check if File Exists
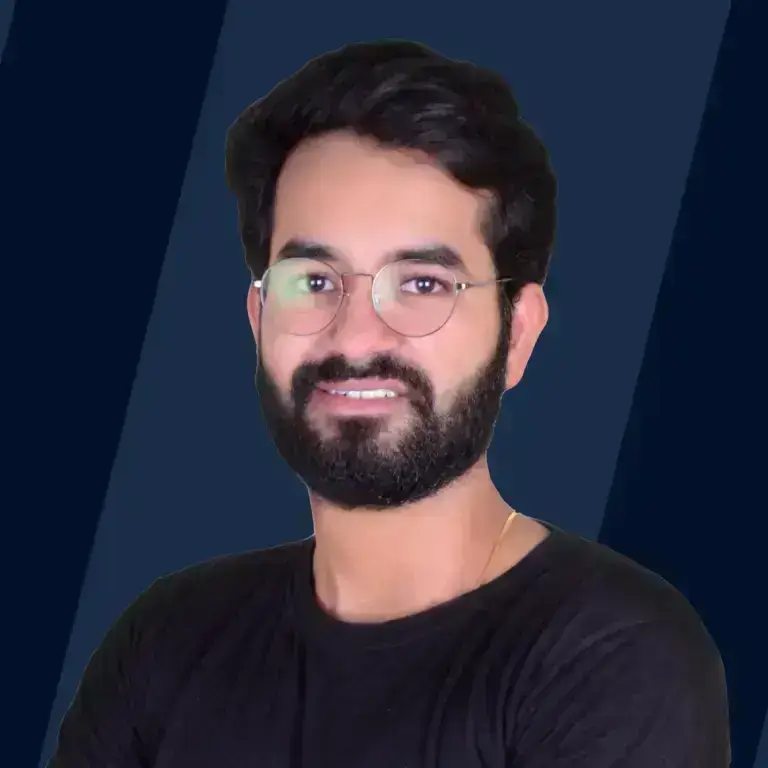
Before performing any operations on a file it is important that we know it exists on your machine. If it does not exist, we may not be able to perform operations on it. We have 4 methods in Python to check if file exists. Those methods use the os and pathlib modules.
How to Check if a File Exists in Python?
Say you were given some homework to do on a file. You already know python, and you decided that you'd play smart by writing some python code to do your tasks. Now once your job is done, you want all of your work to be written into a file. Obviously, all of your work would go to waste if you found out that the file in which you want to write your homework does not exist, or if your Python code has overwritten some of your other work. If your file does not exist, Python will throw a "FileNotFoundError" error.
So, before performing any read or write operations on a file, it would make sense if you checked if it exists. Further ahead in this article, we're going to learn how to check if a file exists, in Python.
There are 4 very common methods:
- Using os.path.exists()
- Using os.path.isfile()
- Using os.path.isdir() (for directories)
- Using pathlib.Path.exists()
Before we move on to the usage of these methods, let's take a look at the 2 modules used: os, and pathlib.
os Module:
The os module provides a plethora of functions that allow you to interact with the operating system. Basically, using these functions you will be able to use any functionality that is dependent on the operating system with python. In order to use this library, you can simply import this library and access the functions.
Implementation:
pathlib Module:
This module offers classes addressing filesystem paths with semantics fitting for various operating systems. These path classes are split between pure paths, which give absolutely computational tasks without I/O, and concrete paths, which acquire from pure paths yet additionally give I/O activities.
Method 1: Using os.path.exists()
Now, to python check if a file exists using the os module, we can use the os.path.exists() function from the os.path module. The os.path module, is a submodule of the os module in Python. It is used for the manipulation of common path names.
This os.path.exists() method will essentially check if the path given as input to this function exists or not.
Syntax:
Parameters:
Only one parameter is given as input to this function, which is the path we would like to check. This path is given in the form of a path-like object that represents the absolute file system path. This object is either a bytes object or a string that represents the path of the file.
Return type:
The return type of this function is boolean. If the path exists, the function returns True and if not, it returns False.
To use this method to check if a file exists in python, we must first import the module. Here's how we would implement it in code.
Note: This file does not currently exist on the machine that this code is run.
Example:
Output:
Since this file does not exist, currently, the code returns False. Once you create this file, you can test this code once more, and the output would be True.
The advantage of this function os.path.exists() is that it can not only be used for files or directories but for complete paths, which is not the case for the other alternatives for checking if a file exists.
Method 2: Using os.path.isfile()
The next method is the os.path.isfile() method. This function will check if the path given as input is an existing file or not.
Syntax:
Parameters:
The only parameter required for this function like the os.path.exists() is the path variable - which contains a path-like object (byte object or a string) to the file (absolute path).
Return Type: Boolean The function returns False if the file does not exist in the specified path and True otherwise.
Example:
The output would depend on whether this file exists locally on the machine.
Method 3: Using os.path.isdir()
Now, this function is not used to python check if a file exists or not, rather, as the name of this function suggests, this function is used to check if the path given as input is an existing directory or not.
The difference in this function is that it follows a symbolic link. This means, that if the path specified in the input is a symbolic link that points to a directory then this function returns True.
By definition, a symbolic link is a simple text string, which is automatically interpreted by the operating system and is followed as a path to a particular file or directory. This particular file or directory is called the "target" and this symbolic link is basically a second file which has an independent existence. Even if the symbolic link to a file or directory is deleted, it does not affect the target. However, if the target (file or directory) is moved to another location, the symbolic link does not update itself. It then simply points to a non-existing location.
Syntax:
Parameters:
This function too takes a single parameter path which is again a path-like object.
Return Type: Boolean Returns True if the directory exists, and False otherwise.
Note: Initially, this directory path does exist on the machine that this code is run.
Example:
If this function is run with the path given that leads to a file, it will return False.
If the input given is a symbolic link, here is how we will check if the target exists:
Since we just created this directory on the machine, the output is True. You can test this method by creating symbolic links to files and directories that exist or do not exist on your machine for practice.
Method 4: Using pathlib.Path.exists()
This last method to python check if file exists makes use of the pathlib module. Pathlib module was introduced in python version 3.4, which means that you can use this method only if you are using a version of python that is 3.4 or above.
The pathlib module will allow you to manipulate files and directories by making use of the object-oriented approach.
To use the pathlib module, we will use the pathlib.Path.exists() method.
Syntax:
Parameters:
The only parameter that this function takes is the path which is a path-like object that represents a file system path much like the other functions we studied earlier.
Return Type:
This method too returns a boolean value. It returns True if the path exists and False if the path does not exist.
Example:
The output will depend on whether or not this path exists. Since this path exists on the machine that this code is run, the code returns True.
You now know how to check if a particular file exists using Python, in 4 different ways!
Conclusion
- File Existence Check: Before any file operations, it's crucial to verify if the file exists to prevent errors.
- Using os.path.exists(): Checks if a path exists, returning True if found, irrespective of whether it's a file or directory.
- os.path.isfile(): Specifically checks if the path exists and is a regular file, returning True if so.
- os.path.isdir(): Verifies if the path exists and points to an existing directory, returning True accordingly.
- pathlib.Path.exists(): Provides an object-oriented approach to check path existence, returning True if found.
- Choice and Usage: Select the appropriate method based on whether you need to check for any existence, file existence, or directory existence.