Python Do While Loop
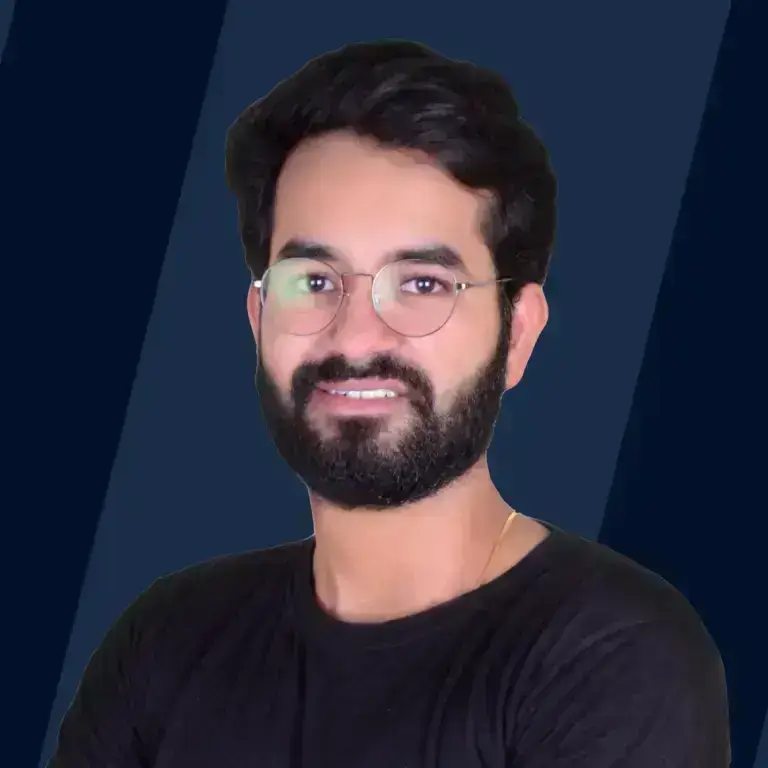
Overview
In general, a "Do While" Loop is a control flow structure that repeatedly executes a block of code while a certain condition is true. The difference between a "Do While" Loop and a regular "While" loop is that a "Do While" Loop executes the block of code at least once, regardless of whether the condition is true or false.
What is a While loop in Python?
Python While Loop is used to execute a block of statements repeatedly until a given condition is satisfied, and when the condition becomes false, the line immediately after the Loop in the program is executed.
Code
What is a do while loop in Python?
A do while loop in python is referred to as an “exit controlled” loop since the looping condition (or predicate) is checked after the code block wrapped inside the do-while Loop is executed once. In contrast, for-loop and while-loop are entry controlled loops. That is, the condition for looping is checked before the code block in the Loop is executed even once.
In short, the do while loop in python executes at least once, irrespective of whether its looping condition is true, while for Loop and while Loop is not executed in a similar case. One of the important examples where this Loop is used is in menu-driven programs, where the menu must be shown to the user at least once and every time they return to the menu screen.
This article will explain the code and the logic for implementing the do-while Loop in Python. Since Python does not explicitly provide its do-while Loop (like C and C++ do), we will have to make a workaround using the existing loops in Python, for-loop, and while-loop. But first, let us look at the control flow for the do-while Loop. A do-while loop in a logic flow diagram (or a flow chart) looks as follows:
The control of the program enters the start of the Loop. The code wrapped within the do while loop in python is executed for the first time, and then after its complete execution, the control goes on to check the looping condition.
Emulating A Do While Loop In Python
Let us build the solution logically by listing what all our code needs to do:
- Enter a code scope irrespective of the looping condition
- Execute the code block
- Check the looping condition
- Repeat until the looping condition becomes false
Seeing the 4th point, we understand we will have to use some looping mechanism. We need what is called an infinite-loop, which provides an unconditional infinite loop and, consequently, allows us to enter the code block without any restriction for the first time. Python, by default, provides two loops, the for-loop and the while loop. And you know what? We can implement a do-while loop in Python using both of them! Let's see them one by one.
1. Using a For Loop to emulate the Do-while loop in Python
Beginners in Python must have used the for Loop over some range, or some list, dictionaries, using some iterable. Now, we will use what is referred to as an iterator (since it provides a procedure to implement infinite loops). To implement the same, we call another method iter().
The syntax for using the iter method to solve our problem is:
Where data_type can be any class, while the arg argument can be any object of any class but not equal to the object returned by that class’ default constructor.
The following table shows the various data types vs. the various possible args, which will allow us to emulate an infinite loop.
int | 1, -2, 1.4, ‘v’, “a random string”, [1,3,4] (anything other than 0) |
---|---|
float | 1, 0.1, 0.001, -3, (anything other than 0 or 0.0) |
dict | Anything other than {} |
list | Anything other than [] |
tuple | Anything other than () |
str | Anything other than ‘’ |
Readers are free to explore more such data_type and arg pairs. But for the sake of simplicity, we will use and suggest the following code snippet for emulating a do-while loop in Python using a for Loop.
Or equivalently
Explanation:
- The control enters the for Loop. After every execution, the Loop will try to check if the looping variable (_), which was initially 0 (because 0 is the value returned by constructor int()), has been able to become equal to the value of the second parameter, that is 1. Since we make no changes to the iterating variable _ inside the code block, it never becomes equal to 1, and therefore, we get an infinite loop.
- While executing the code block inside the loop block, if the condition is evaluated to be true, then nothing will happen. The execution will continue to the next iteration. Otherwise, the control will break out of the Loop.
2. Using a While Loop
It is much simpler than implementing an infinite loop using for Loop in Python. The general structure of a while loop in Python is given below:
Now, if we want to enter the while loop unconditionally, we can give it a true value forever. Can you guess what such a value can be? Well, the boolean True itself!
Now, this has become an infinite loop that will execute unbounded. But we want a condition check, don’t we? So, just like we did above in for loop implementation, we add an if-else block to check the looping condition and break out of the Loop if the condition is not met.
Or equivalently
Example
Output:
Explanation:
The control enters the beginning of the Loop and evaluates the predicate given in front of while. Since it is true by default, the control enters the code block and executes statements present inside. The variable i is incremented, and it becomes 11. Further, since the condition (11<10) evaluates to false, the control breaks out of the Loop.
An interesting thing to notice is that the code executes at least once even though our looping condition requires that i < 10.
Similar code using the for loop implementation:
Output:
Explanation:
The control arrives at the loop block, and since _ is not equal to 1 (as it is equal to 0), the control enters the loop block and executes the statements in it. Since i is equal to 10, the condition i>=10 is considered true, and the control breaks out of the loop block.
Let’s see one more example of printing the first five multiples of 2 using both implementations.
Ouput:
Equivalently:
Output
The explanation for these two programs is similar to the code samples above.
Conclusion
The article can be summarized as follows:
- Since there is not an in-built do while loop in python, we implemented the do-while Loop in Python using for-loop and while-loop, which are already present in the language.
- For-loop uses the iter() method for emulating the do-while Loop in Python
- To convert a while-loop into a do-while loop in Python, we require just a True boolean as Loop conditional. Both methods further require if-else conditions to break out of the infinite loop at the desired execution stage.