Python Program to Get Hostname
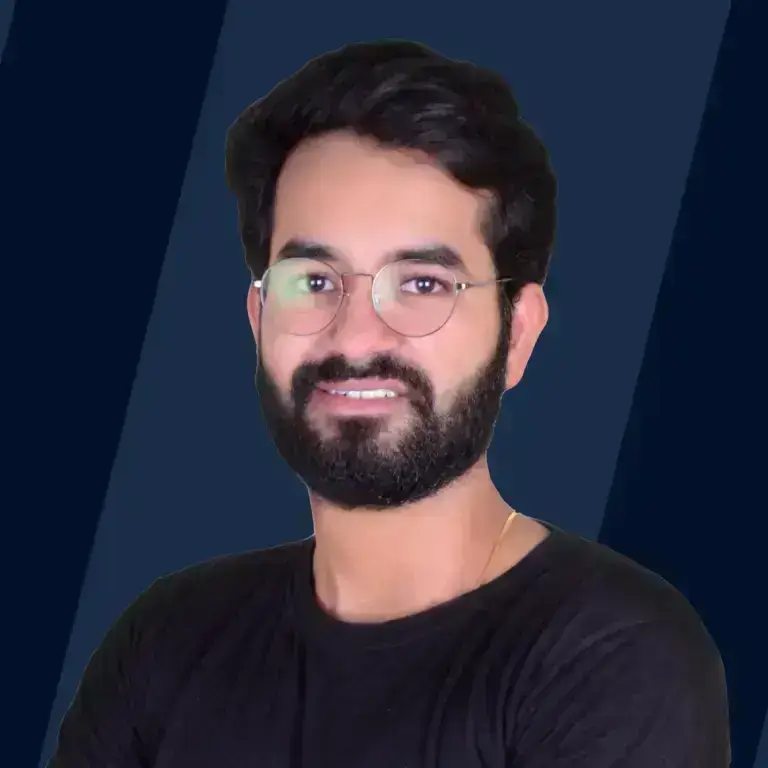
Overview
Python offers a wide range of networking-related applications. It is frequently used by developers as a means of communication with other users on your network. Hence, these communications require the hostname in Python. The hostname is the name that is provided to a device to identify it in a local network. Basically, by using the hostname, the machines can communicate within a network. In this article, we will learn about the Python get hostname function and various ways for the same.
What is Hostname?
The hostname is a name or alias that is used to identify a device that may be unique among all the devices linked to a network node. This hostname, which can be entirely distinct, can be used to distinguish between several devices.
Ascii character combinations are typically used as hostnames, but this can change depending on the network.
Multiple devices may share the same hostname in some situations if the hostnames are changed forcibly. However, they may have separate MAC addresses even though they share the same hostname.
Takeaway: The name provided to a device to identify the device in a local network is referred to as a hostname. The use of hostnames enables machine communication within a network. We will learn about Python get hostname in this article.
Get Hostname in Python
Python gets hostname function can be used to retrieve a network device's name or alias using either the device's IP address or domain name. In general, the hostnames refer to the name of the devices which can be used to differentiate them among different devices. Additionally, you can also know the hostname of the machine which is hosting your Python interpreter.
You can get the hostname in windows by the below command:
Also, you can fetch the hostname in Linux by the below command:
In Python, there are ample ways we can get the Hostname. They are as follows:
- Method 1: Using Platform Module
- Method 2: Using Socket Module
- Method 3: Using os Module
- Method 4: Using socket gethostbyaddr Function
Let us discuss each of them in brief.
Method 1: Using the Platform Module to Find the Hostname in Python
The Platform module in python basically provides the access to the underlying platform’s identifying data. It is widely used to access the platform information, which may include the hostnames, IP addresses, Operating system, and various other information. It can be used for Python to get a hostname.
There are several functions under the Platform module, but we will employ the node function from the platform module to find the hostname.
The platform.node module basically returns the computer's network name, however, sometimes it might not be accurate (according to the documentation). Also, an empty string is returned by the platform.node in case the value of the hostname cannot be determined.
Also, please note that we need to import the platform module before using the same.
Below given is the syntax for the Platform Module.
Syntax:
Parameters: The platform.node() function does not take any parameter as input.
Return Value: The platform.node() function returns a string, which contains the device's hostname, where the python interpreter has been installed.
Let us look into a few of the examples for the same.
Code 1:
Output:
Explanation: In the above code, we imported the platform module. After that, we wrote the platform.node() function to get the hostname of our device. The Node() function returns the device's hostname if the hostname of the device is available, otherwise returns an empty string if the hostname is not available.
Method 2: Using the Socket Module to Find the Hostname in Python
In Python, the Socket module is one of the most important and influential networking modules. Using the socket module, you can communicate with the sockets and even connect to the other devices using them.
The socket module can be used after importing the socket module in Python. Inside the Socket Module, there is a gethostname() function that can be used to find the hostname in Python, or, as Python get hostname.
Below given is the syntax for Socket Module.
Syntax:
Parameters: The socket.gethostname() function does not take any parameter as input.
Return Value: The socket.gethostname() function returns a string, which contains the machine's hostname, where the python interpreter has been installed.
Let us look into a few of the examples for the same.
Code 1:
Output:
Explanation: In the above code, we imported the socket module. After that, we wrote the socket.gethostname() function, which is responsible for fetching the hostname of our device. The gethostname() function will get the hostname of the computer, where the Python interpreter is running.
Method 3: Using the OS Module to Find the Hostname in Python
Python's OS Module is mainly responsible for interacting with the operating system. The OS Module contains several functions that can interact with the operating system and provides us with a portable way of using the operating system-dependent functionalities.
Below given is the syntax for OS Module.
Syntax:
Please note that the uname() function does not work for the Windows system. It works well for Linux systems.
Parameters: The os.uname() function does not take any parameter as input.
Return Value: The os.uname() function returns an object which consists of five attributes, which are as follows: sysname – the name of the operating system. nodename – the hostname, or the name of the machine on the network. release – the OS release version – the OS version machine – the hardware identifier.
Let us now look into the code for using the os.uname() function for windows machine:
Code 1:
Output:
Explanation: In the above code, we imported the platform and the os module. After that, we kept a check whether the system is windows or not by using the platform.system() == "Windows": command. If the system is windows, then we will check the hostname of the system through the command platform.uname().node, otherwise we can check the hostname of the system through the command os.uname()[1]. Please note that the uname() function does not work for the Windows system.
Method 4: Using the Socket gethostbyaddr() function to find the hostname in Python
The gethostbyaddr function is present under the socket module and returns the hostname(along with other pieces of information), provided that we pass the IP address of the system as a parameter.
Below given is the syntax for the gethostbyaddr() function.
Syntax:
Parameters: The socket.gethostbyaddr(ip_address) function takes the IP address as the parameter.
Return Value: The socket.gethostbyaddr(ip_address) function returns a tuple, which contains the below information:
- Host Name of the device
- Alias list for the IP address (if any)
- IP address of the host
Code 1:
Output:
Explanation: In the above code, we imported the socket module. After that, we wrote the socket.gethostbyaddr() function, passing the IP address as parameter() to get the hostname of our device. Normally, it returns the same hostname that was put in as a parameter on local networks, but on distant networks, it may return the remote hostnames.
Get Hostname by URL in Python
We often need to access the domain names, from the URLs which we already have. Python provides ample ways to do this. For example, some of the ways are urllib, Regex, string splitting, or using some other modules. All of these functions work properly, but we usually prefer the urllib module the most. The reason for this is that the urllib comes under the networking module and is also present to us by default. Hence, we need not put some extra lines of code for using the urllib module and its functions.
In this article we will be using the urllib.parse function from the urilib module to parse the URLs. The urllib.parse module defines functions that fall into two categories: URL parsing and URL quoting.
URL Parsing We will be using URL parsing to perform our task. The URL parsing functions are concerned with either splitting a URL string into its constituent components or combining URL components into a URL string. You can learn in detail about the URL parsing function in the Python docs.
Let us now look into the code for the same.
Code:
Output:
Explanation: In the above code, we have first imported the urlparse function from the urllib parser. This function can be used to parse the URLs into meaningful strings. Once we initialize the urlparse() with an URL, it further breaks down the URL into scheme, netloc, params, query, and fragment. After that, we can use the scheme and netlock to extract the protocol and hostnames from the given URL.
Get Hostname by IP in Python
Sometimes, you have the IP addresses in your code results. Then by using them, you can easily deduce the hostnames of the servers. But importantly, your IP should be working and reachable by your network. In the following example, I’ve used 8.8.8.8 as IP, which is Google’s DNS.
Let us look into a code example, where we can get the hostname by using the IP address in Python. In the example, I have used 8.8.8.8 as the IP address, which is Google's DNS.
Code:
Output:
Explanation: In the above code example, we have imported the socket module in Python. We have used the socket.gethostbyaddr(IP) function, passing our IP address as the parameter. It returns a tuple of data, which contains the Hostname, alias list, and the IP Address List. From that list, by tuple indexing, we have figured out the hostname of the server.
It returns a string containing the hostname of the machine where the Python interpreter is currently executing.
FAQs
Q: What does the socket.gethostname() Function do?
A: The socket.gethostname() function returns a string, which contains the hostname of the machine where the Python interpreter is currently executing.
Q: What does the socket.gethostbyaddr(ip_address) Function do?
A: The socket.gethostbyaddr(ip_address) the function takes the IP address as the parameter and returns a tuple which contains the hostname, aliaslist and the ip_address_list. The hostname is the primary host name responding to the given ip_address, whereas the alias list(usually empty) is a list of alternative hostnames for the same address. The ip_address_list is a list of IPv4/v6 addresses for the same interface on the same host and usually contains a single address.
Q: What does the platform.node() Function do?
A: The platform.node() function basically returns the computer's network name (hostname). Sometimes an empty string is returned if the value cannot be determined.
Conclusion
In this article, we have learned about the Python Program to Get Hostname, or, Python get the hostname. Let us note down the important points we studied till now --
- The name provided to a device to identify it in a local network is referred to as a hostname. The use of hostnames enables machine communication within a network.
- Python get hostname function can be used to retrieve a network device's name or alias using either the device's IP address or domain name.
- The platform.node module in Python returns the computer's network name, however sometimes it might not be accurate.
- Inside Python's Socket Module, there is a gethostname() function that can be used to find the hostname in Python.
- The os.uname() function from the OS module in Python can also be used to find the hostname, however, it does not work for the windows system.
- The gethostbyaddr function is present under the socket module, takes the IP address as a parameter and returns the hostname (along with other information as well).
- We can get hostname by URL in Python, by using the urllib.parse function of the urllib module.
- We can get hostname by IP address in Python by using the socket.gethostbyaddr() function from the socket module.