Python List Remove()
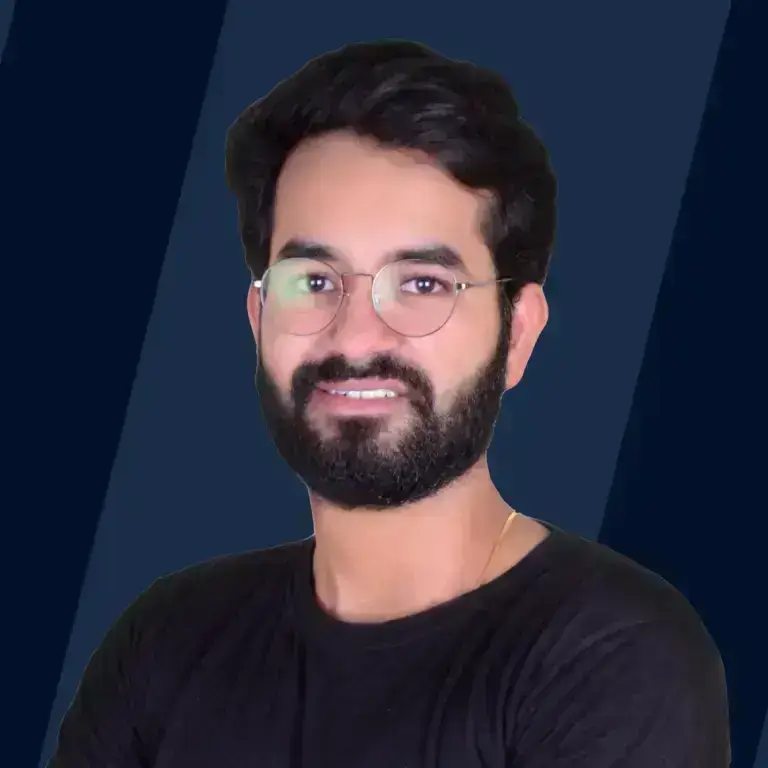
Overview
The remove function in python is used to remove that element from the list, which is passed as the parameter to the function.
The remove function updates the original list instead of creating a new list. After removing the element from the list, the list rearranges itself in the same order.
What is Remove in Python?
Have you ever wondered if you have a list of students in the class, And at any instance, if you want to remove the name of any student from that list, What can you do? Remove function in python is the best approach to this problem. Remove function python is used to remove the element present in the list. We have to pass that element as a parameter to the remove function, which then removes the element.
If there are several occurrences of the same element, it will remove the first occurrence of the element. If someone passes that object not present in the list, then it will raise an error Val, not in list.
Example
Suppose you have a list containing the names of students in the class. If you want to remove the element from the list, you can use the remove function in python. The remove function accepts the value of the element that is to be removed from the list. It doesn't accept the index of the element that is to be removed.
CODE:
OUTPUT:
Syntax
The syntax of the remove function in python is explained below:
The remove function is used with the list to remove an item from the list. Read along to learn more about its parameter.
Parameter
We can see that it accepts a parameter as we have understood the syntax of the remove function in python. This parameter is explained below:
- Val (Mandatory Parameter): Val represents the list value we want to remove.
Return Value
The remove function doesn't return anything, and it just removes an element from the list.
How to Use remove() in Python?
To remove the list element, we have to use the remove function in python. We need to call the remove() on the list object. After calling the function, we must pass the element as the parameter to the remove function. After deleting the element from the array, the array re-arranges itself.
Let's move on to the example to see how we can practically use the remove function in python.
How to Delete Elements From the List?
Suppose you have a list of common programming languages. You can use the remove function in python for removing the element from the list.
CODE:
OUTPUT:
Example
Remove an Element from the list
In this example, we will demonstrate how can we remove an element of the list with the help of the remove function in python.
CODE:
OUTPUT:
EXPLANATION:
We have created an array that stores the number in it. We have used python remove function to remove element 5 from the list.
Remove an Element having multiple occurrences in the list
In this example, we will learn what will happen if there are multiple occurrences of the same element we want to remove from the list.
In that case, the remove function in python removes the first occurrence of the element present in the list.
CODE:
OUTPUT:
EXPLANATION:
We have created an array that stores the number in it. We have used python remove function to remove element 1 from the list. If there are several occurrences of element 1 in the array, then the remove function deletes the first occurrence of the element from it.
Removing all Elements having multiple occurrences in the list
In this example, we will learn how to remove all the occurrences of the element present in the list. We have to use a loop to remove all the occurrences.
CODE:
OUTPUT:
EXPLANATION:
We have created an array that stores the number in it. We have used python remove function to remove element 2 from the list. If there are several occurrences of element 2 in the array, then the remove function deletes the first occurrence of the element from it.
To remove all the occurrences of 2 from the list, we have to use a loop to delete all occurrences of 2. The steps are as follows:
- Count the occurrence of the element to be removed from the list.
- Use a loop from 1 to `the number of occurrences of that element.
- During each iteration, use the remove function to remove that element from the list.
After these steps, you will get an updated list where every occurrence of element 2 is removed from the list.
Passing the Value as Parameter which is not present in the list
In this example, we will understand what will happen when the user passes a wrong element/value as a parameter to the remove function in python. In that case, the code will raise the error that value is not present in the list.
CODE:
OUTPUT:
EXPLANATION:
We have created an array that stores the number in it. We have to use the python remove function to remove element 10 from the list. As we know, element 10 is not present in the list. So, it will raise an error that an element is not in the list.
Conclusion
- The remove function is used in Python to remove an item from the list,which is passed as it's parameter.
- The syntax of the remove function in python is : list.remove(val)
- If there are multiple occurrences of an element we want to remove from the list,the remove function in python removes the first occurrence of the element present in the list.
- To remove all the occurrences of the element present in the list, we have to use a loop with remove().