Python slice() Function
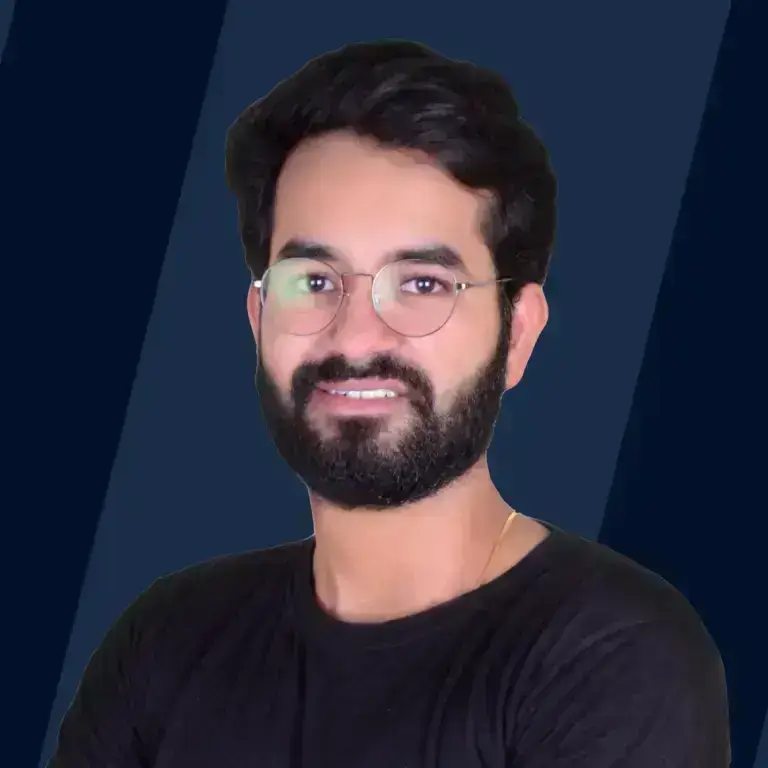
Overview
slice() function in python is used to extract a continous sequence(it may be an empty sequence, complete sequence or a partial sequence) of any object(array, string, tuple). It returns a slice object which can be used with the array/string which will return the continous sequence after slicing.
Syntax of slice() function in Python
The syntax of slice function in python is explained below:
The slice() function returns an object which has 3 parameters startIndex, endIndex and steps. After generating the object, this object is used for slicing of the sequence. This function takes 3 parameters in it, out of which two are Optional while one is Mandatory Parameter. Read along to know more about Parameters of Slice Function.
Parameters of slice() function in Python
Slice function in python accepts 3 parameters in it. These are:
- Start(Optional Parameter): Start index of the sequence where slicing is to be start. Default Value of Start in Slice Function is 0 i.e. the slicing will start from the starting of the string.
- Stop(Mandatory Parameter): End index of the sequence where slicing to be end.
- Steps(Optional Parameter): Integeral value which determines the increment between each index of slicing. Default Value of Step in Slice Function is None.
In case if user pass the invalid indices which exceed the last index of the list then it will raise the error INDEX OUT OF BOUND.
Return Value of slice() function in Python
It returns an object of slice class, which contains the startingIndex, endingIndex, and step. We then use that slice object with any sequence to slice it.
Example of slice() function in Python
In this example we are going to look over the result which is returned by the slice() in python. This is just a basic example. We will understand the syntax, parameters and other things about slice() after this example.
Code:
Output:
What is Slice Function in Python?
Ever wondered how you can get the domain name from the emailId of the user. Here slice function of python can be used to efficiently solve this problem. If we want to extract part of a string, or some part of a list, you use slice function in python. The slice function returns the sliced object which has all the value startIndex, endIndex, steps on the basis of which it slice any sequence.
Examples
Create a Slice Object for Slicing
The below code creates the slice object which in future is used for slicing of the sequence.
Code:
Output:
Slicing Substring Using Slice Object
As we learn how to create a slice object of the slice class. Now we are going to use that slice object to slice our given sequence. Let's write the code to implement that problem.
Code:
Output:
Explanation:
- Here we have created 3 types of slice objects.
- In first one we just pass end index as a parameter to the slice function.
- In second one we pass the starting and the end index as a parameter to the slice function.
- In third case we pass all the parameters to the slice function.
- We then use all the slice object on the string to slice the string on the basis of parameters passed to the slice function.
Slicing Substring Using Negative Index
In this example we will write a code for creating a slice object but the slicing function in this case will take negative parameters in it and then we slice a sequence with that generated slice object.
Code:
Output:
Explanation:
- First of all, we have created a slice object of the slice function.
- In the slice function we have passed the negative indices as the parameter.
- When we pass negative indices it will start slicing from the end of the sequence as represnted in the image.
Get Sublist and Sub-Tuple
In this example first we will create a slice object of the slice class. We then use that slice object with the array and tuple to get the sub-array/sub-tuple. The slice object splits the array/tuple from the startIndex to endIndex as passed in slice object.
Code:
Output:
Explanation:
- We have created two different sliced object one for slicing the array and other for slicing of tuple.
- We then used these sliced objects to get the desired result.
Get Sublist and Sub-Tuple Using Negative Index
As we know, the concept of negative index in python. Negative index starts from the end of the sequence i.e. -1 and continues till the start of the sequence. Below image explains the concept of negative index properly.
In this example we are going to create a slice object first but here we pass negative index as its parameter and then we will use that slice object to get a sublist or a sub-tuple.
Code:
Output:
Explanation:
- First of all, we have created a slice object of the slice function.
- In the slice function we have passed the negative indices as the parameter.
- When we pass negative indices it will start slicing from the end of the sequence as represnted in the image.
Using Indexing Syntax for Slicing
In this example we will slice an array by just using the indexing operator instead of using slice function in python. Here also, we will provide the start index, end index and steps to get the sliced sequence.
Code:
Output:
Explanation:
- Here we have used the indexing syntax which also accepts the same parameter like the slice function seperated by colons i.e. :.
- As we have passed start index as 0, end index as 9 and step of 2.
- So the slicing will start from 0 till 9th index (exclusive).
Using Negative Index
In this example we will slice an array by just using the indexing operator but we will provide negative index as parameter to them instead of using slice function in python. Here also, we will provide the start index, end index and steps to get the sliced sequence.
When we provide negative index for slicing the sequence then, the slicing process will start from the end of the list.
Code:
Output:
Explanation:
- First of all, we have created a slice object of the slice function.
- In the slice function we have passed the negative indices as the parameter.
- When we pass negative indices it will start slicing from the end of the sequence as represnted in the image.
Conclusion
- Slice function is used for slicing any kind of sequence(arrays, tuples, strings).
- Slice function accepts 3 parameters out of which 2 paramters are optional.
- Default value of startIndex and step is 0 and 1 respectively. It means if we haven't pass any parameter as start index it will start slicing from the beginning of the string. Also, if we haven't pass step it means that the slicing will be done after every iteration.
- Slice function can be used to find the last n elements or first n elements or even middle n elements of the list.
See Also
- Indexing in Array & Strings
- Immutable nature of Strings