Python Comprehensions
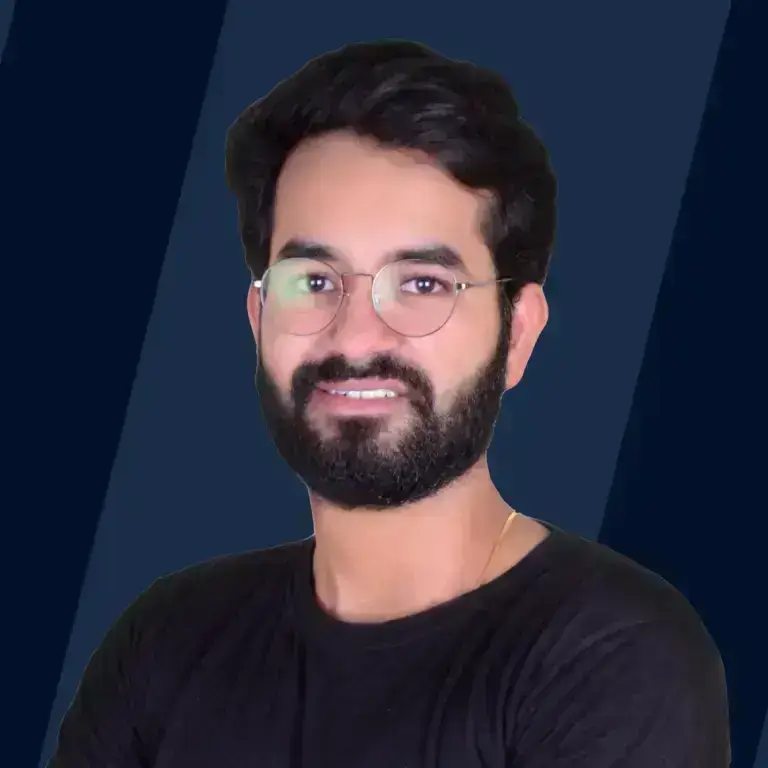
Overview
Comprehension in python provide a concise and expressive way to create lists, dictionaries, and sets. They allow you to generate these data structures in a single line of code, making your code more readable and efficient.
Types of Comprehensions in Python
List comprehensions in Python
List comprehensions in Python provide a concise way to create lists. They allow you to create a new list by applying an expression to each item in an existing iterable (e.g., a list, tuple, or string), optionally with a condition to filter items.
Syntax:
- new_list: new list being created.
- expression: refers to the expression that would be used to manipulate the elements in the list. It could be a formula or a simple value.
- item: refers to the original element in the list.
- iterable: refers to the original sequence being used to create a new_list.
- condition: It is an optional parameter used to reduce the length of the new_list, making it shorter than the original sequence.
Now, let’s understand this better with some examples.
Example 1:
Considering the example of converting a list of red balls to red. All we do is create a red_list from the existing blue_list by naming each element as red in the new list.
Output:
Example 2:
Considering you have a list of consecutive even numbers and want to create a list of consecutive odd numbers, you can do it by adding 1 to every element of the list. This is achieved by considering the item in even_list as var and then adding 1 to it, as shown in the following code:
Output:
Dictionary comprehensions in Python?
Dictionary comprehensions are used to create a fresh dictionary from an existing sequence of dictionaries or lists by manipulating its elements. They have a similar syntax to list comprehensions and set comprehensions but are used to generate dictionaries.
Syntax:
- new_dict: new dictionary being created.
- key: The expression for the dictionary key.
- value: The expression for the dictionary value.
- item: The variable representing an element in the original iterable.
- iterable: The existing sequence you are iterating over.
- condition (optional): An optional filtering condition. The item is included in the new dictionary only if this condition is true.
Let’s understand this better with some examples.
Example 1:
Consider a list of dictionary objects containing words and their meanings where each dictionary is in the format:
We aim to create a sequence of dictionaries where each dictionary object is in the format:
To do so, we create a new dictionary using dictionary comprehension as follows:
In the above code, d[‘word’] represents the value held by the key, word and the d[‘meaning’] represents the value held by the key meaning.
Output:
Example 2:
Consider the following example of 1 list, one containing the months of a year and another consisting of natural numbers up to 12. We aim to create a dictionary where the key is the number of the month, and the value is the month's name. A dictionary using dictionary comprehension for the given purpose would be created as follows:
Output:
Set comprehensions in Python
In Python, set comprehensions are used to create a new set from an existing sequence (set) by manipulating its elements.They have a similar syntax to list comprehensions, but they generate sets instead.
Syntax:
- new_set: new set being created.
- expression: The operation or value to be included in the new set.
- item: The variable representing an element in the original iterable.
- iterable: The existing sequence you are iterating over.
- condition (optional): An optional filtering condition. The item is included in the new set only if this condition is true.
Let’s understand this better with some examples.
Example 1:
Considering the given example of converting a set of red balls to red. All we do is create a red_set from the existing blue_set by naming each element as red in the new set as follows:
The entire code for this example is given below:
Output:
Example 2:
Consider you have a list of consecutive even numbers and want to create a set of consecutive odd numbers. You can do it by adding 1 to every element of the set. This is done by considering each item in the existing set even_set as var and then adding 1 to var, as shown in the code below:
Output:
Generator comprehensions in Python
This is one of the most rarely used and least known comprehensions in Python. In Python, generator comprehensions are used to create a new generator from an existing list by manipulating its elements. A generator in Python is a function that returns an iterator that holds a sequence of values instead of a single value. Generators are usually memory efficient as they allocate memory on the generation of items rather than at the beginning.
Syntax:
- new_gen: new generator being created.
- expression: The operation or value to be included in the new set.
- item: The variable representing an element in the original iterable.
- iterable: The existing sequence you are iterating over.
- condition (optional): An optional filtering condition. The item is included in the new set only if this condition is true.
Now, let’s understand this better with an example.
Consider you have been given a list of the first 6 even numbers, and you want to generate a list consisting of the first 6 odd numbers from it. You would simply add a 1 to the numbers given in the existing list. The same can be coded as follows:
Output:
Conclusion
- Comprehension in Python are used to create new sequences from existing ones, and the type of the new and original sequence might not be the same.
- Comprehension in Python are classified into four types based on the sequence generated. These are list comprehensions, dictionary comprehensions, set comprehensions, and generator comprehensions.
- Comprehension in Python can be used to make the code more readable and also make the code run faster in most cases since Python first allocates memory for the list and then adds elements.
- Comprehension in Python are more often used when you want to sophisticatedly filter lists, use conditional logic to create them, or map elements.