Differences Between List, Tuple, Set and Dictionary in Python
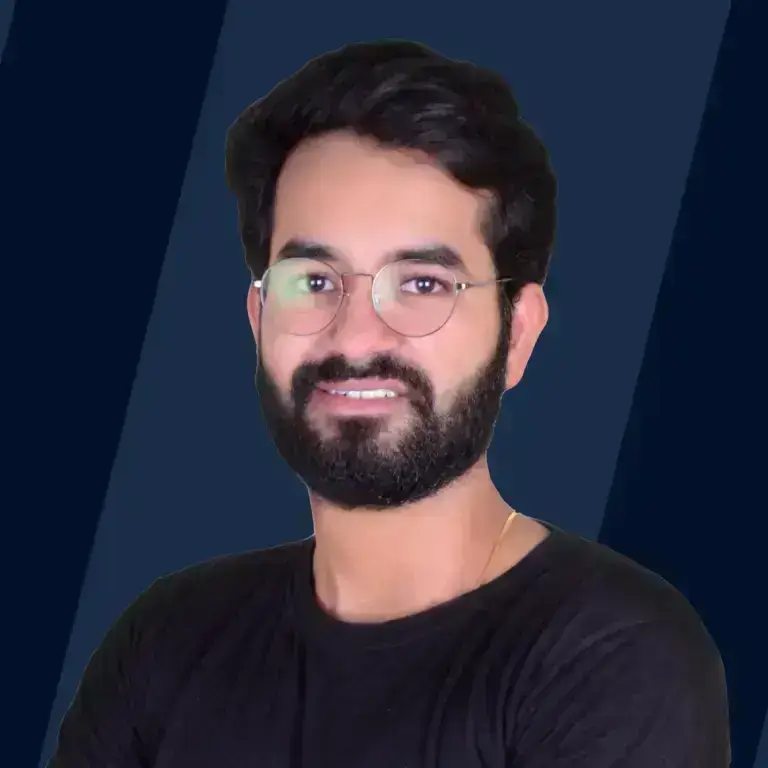
Overview
Data structures in python provide us with a way of storing and arranging data that is easily accessible and modifiable. These data structures include collections. Some of the most commonly used built-in collections are lists, sets, tuples and dictionary.
Introduction
Having a hard time understanding what Lists, tuples, sets and dictionaries are in python? Everyone who works on python at least once has been confused about the differences between them or when to use them. Well, say no more, as we'll break down these Data structure collections' differences and understand them so that we may never forget them!
Let’s take a closer look at what each of these collections are :
Key Difference Between List, Tuple, Set, and Dictionary in Python
Mutability:
- List: Mutable (modifiable).
- Tuple: Immutable (non-modifiable).
- Set: Mutable, but elements inside must be immutable.
- Dictionary: Mutable; keys are immutable, but values can change.
Order:
- List: Maintains order of elements.
- Tuple: Maintains order of elements.
- Set: No guaranteed order.
- Dictionary: As of Python 3.7+, insertion order is preserved.
Uniqueness:
- List: Allows duplicates.
- Tuple: Allows duplicates.
- Set: Only unique elements.
- Dictionary: Unique keys, values can be duplicated.
Data Structure:
- List: Ordered collection.
- Tuple: Ordered collection.
- Set: Unordered collection.
- Dictionary: Collection of key-value pairs.
Difference Between List VS Tuple VS Set VS Dictionary in Python
Parameters | List | Tuple | Set | Dictionary |
---|---|---|---|---|
Definition | A list is an ordered, mutable collection of elements. | A tuple is an ordered, immutable collection of elements. | A set is an unordered collection of unique elements. | A dictionary is an unordered collection of key-value pairs. |
Syntax | Syntax includes square brackets [ , ] with ‘,’ separated data. | Syntax includes curved brackets ( , ) with ‘,’ separated data. | Syntax includes curly brackets { , } with ‘,’ separated data. | Syntax includes curly brackets { , } with ‘,’ separated key-value data. |
Creation | A list can be created using the list() function or simple assignment to []. | Tuple can be created using the tuple() function. | A set dictionary can be created using the set() function. | A dictionary can be created using the dict() function. |
Empty Data Structure | An empty list can be created by l = []. | An empty tuple can be created by t = (). | An empty set can be created by s = set(). | An empty dictionary can be created by {}. |
Order | It is an ordered collection of data. | It is also an ordered collection of data. | It is an unordered collection of data. | Ordered collection in Python version 3.7, unordered in Python Version=3.6. |
Duplicate Data | Duplicate data entry is allowed in a List. | Duplicate data entry is allowed in a Tuple. | All elements are unique in a Set. | Keys are unique, but two different keys CAN have the same value. |
Indexing | Has integer based indexing that starts from ‘0’. | Also has integer based indexing that starts from ‘0’. | Does NOT have an index based mechanism. | Has a Key based indexing i.e. keys identify the value. |
Addition | New items can be added using the append() method. | Being immutable, new data cannot be added to it. | The add() method adds an element to a set. | update() method updates specific key-value pair. |
Deletion | Pop() method allows deleting an element. | Being immutable, no data can be popped/deleted. | Elements can be randomly deleted using pop(). | pop(key) removes specified key along with its value. |
Sorting | sort() method sorts the elements. | Immutable, so sorting method is not applicable. | Unordered, so sorting is not advised. | Keys are sorted by using the sorted() method. |
Search | index() returns index of first occurrence. | index() returns index of first occurrence. | Unordered, so searching is not applicable. | get(key) returns value against specified key. |
Reversing | reverse() method reverses the list. | Immutable, so reverse method is not applicable. | Unordered, so reverse is not advised. | No integer-based indexing, so no reversal. |
Count | count() method returns occurrence count. | count() method returns occurrence count. | count() not defined for sets. | count() not defined for dictionaries. |
Let us know more about Lists, Tuples, Sets and Dictionaries in following topics:
List
Lists are one of the most commonly used data structures provided by python; they are a collection of iterable, mutable and ordered data. They can contain duplicate data.
Certainly! Here are the code snippets extracted and organized with suitable subheadings for each data structure:
Syntax
Code:
Output:
Indexing
Code:
Output:
Adding New Element
Code:
Output:
Deleting Element
Code:
Output:
Sorting Elements
Code:
Output:
Searching Elements
Code:
Output:
Reversing Elements
Code:
Output:
Counting Elements
Code:
Output:
Tuple
Tuples are similar to lists. This collection also has iterable, ordered, and (can contain) repetitive data, just like lists. But unlike lists, tuples are immutable.
Syntax
Code:
Output:
Indexing
Code:
Output:
Set
Set is another data structure that holds a collection of unordered, iterable and mutable data. But it only contains unique elements.
Certainly! Here are the code snippets for the "Set" section of the table, including the Syntax and Searching Elements sections:
Syntax
Code:
Output:
Dictionary
Unlike all other collection types, dictionaries strictly contain key-value pairs.
- In Python versions < 3.7: is an unordered collection of data.
- In Python v3.1: a new type of dictionary called ‘OrderedDict’ was introduced, which was similar to dictionary in python; the difference was that orderedDict was ordered (as the name suggests)
- In the latest version of Python, i.e. 3.7: Finally, in python 3.7, dictionary is now an ordered collection of key-value pairs. The order is now guaranteed in the insertion order, i.e. the order in which they were inserted.
Syntax
Code:
Output:
Indexing
Code:
Output:
Adding New Element
Code:
Output:
Deleting Element
Code:
Output:
Sorting Elements
Code:
Output:
Searching Elements
Code:
Output:
If you’re questioning if both orderedDict and dict are ordered in python 3.7. Then why are there two implementations? Is there any difference?
We can leave that for when we talk about dictionaries in detail. But until then, we should know what dictionaries are, and their purpose, as that is something that will never change.
Collection VS Features | Mutable | Ordered | Indexing | Duplicate Data |
---|---|---|---|---|
List | ✔ | ✔ | ✔ | ✔ |
Tuple | 𐄂 | ✔ | ✔ | ✔ |
Set | ✔ | 𐄂 | 𐄂 | 𐄂 |
Dictionary | ✔ | ✔ | ✔ | 𐄂 |
NOTE: An ordered collection is when the data structure retains the order in which the data was added, whereas such order is not retained by the data structure in an unordered collection.
- Sets, on one hand, are an unordered collection, whereas a dictionary (in python v3.6 and before) on the other may seem ordered, but it is not. It does retain the keys added to it, but at the same time, accessibility at a specific integer index is not possible; instead, accessibility via the key is possible.
- Each key is unique in a dictionary and acts as an index as you can access values using it. But the order in which keys are stored in a dictionary is not maintained, hence unordered. Whereas python 3.7’s Dictionary and ‘OrderedDict’ introduced in python 3.1 are ordered collections of key-value data as they maintain the insertion order.
Let’s dig deeper into the differences between these collections :
Key Differences Between Dictionary, List, Set, and Tuple
Syntax
- Dictionary: Uses curly brackets { } with key-value pairs separated by commas.
- List: Employs square brackets [ ] with comma-separated elements.
- Set: Utilizes curly brackets { } with comma-separated elements.
- Tuple: Employs parentheses ( ) with comma-separated elements.
Order
- Dictionary: Maintains order in Python 3.7+ but is unordered in Python 3.6.
- List: Maintains order.
- Set: Unordered.
- Tuple: Maintains order.
Duplicate Data
- Dictionary: Keys are unique, values can be duplicated.
- List: Allows duplicate elements.
- Set: Does not allow duplicate elements.
- Tuple: Allows duplicate elements.
Indexing
- Dictionary: Key-based indexing.
- List: Integer-based indexing starting from 0.
- Set: No index-based mechanism.
- Tuple: Integer-based indexing starting from 0.
Adding Elements
- Dictionary: Uses key-value pairs.
- List: New items can be added using append() method.
- Set: Uses add() method.
- Tuple: Being immutable, new data cannot be added.
Deleting Elements
- Dictionary: Uses pop(key) method to remove specified key and value.
- List: Uses pop() method to delete an element.
- Set: Uses pop() method to remove an element.
- Tuple: Being immutable, no data can be popped or deleted.
Sorting Elements
- Dictionary: Keys can be sorted using the sorted() method.
- List: Uses sort() method to sort elements.
- Set: Unordered, so sorting is not applicable.
- Tuple: Being immutable, data cannot be sorted.
Searching Elements
- Dictionary: Uses the get(key) method to retrieve value for a specified key.
- List: Uses index() method to search and return index of first occurrence.
- Set: Unordered, so searching is not applicable.
- Tuple: Uses index() method to search and return index of first occurrence.
Reversing Elements
- Dictionary: No integer-based indexing, so no reversal.
- List: Uses reverse() method to reverse elements.
- Set: Unordered, so reversing is not advised.
- Tuple: Being immutable, reverse method is not applicable.
Counting Elements
- Dictionary: count() not defined for dictionaries.
- List: Uses count() method to count occurrence of a specific element.
- Set: count() is not defined for sets.
- Tuple: Uses count() method to count occurrence of a specific element.
Conclusion
- Lists and Tuples vary in mutability as lists are mutable, whereas tuples are not.
- Set is the only unordered collection in python 3.7.
- Dictionaries store data in the form of key-value pairs in python and remain a controversy when it comes to whether they’re ordered or unordered. As this varies with multiple versions of python. Python v<=3.6 has dictionaries as unordered, but at the same time, orderedDict was introduced in python 3.1, and then finally python 3.7 has both orderedDict and Dictionary and now both are ordered.
Hope this information gives more clarity on what are the differences between list, tuple, set and dictionary in python. And what use cases do they serve and hope it gives you clarity on when to use which collection.