Python Collection Module
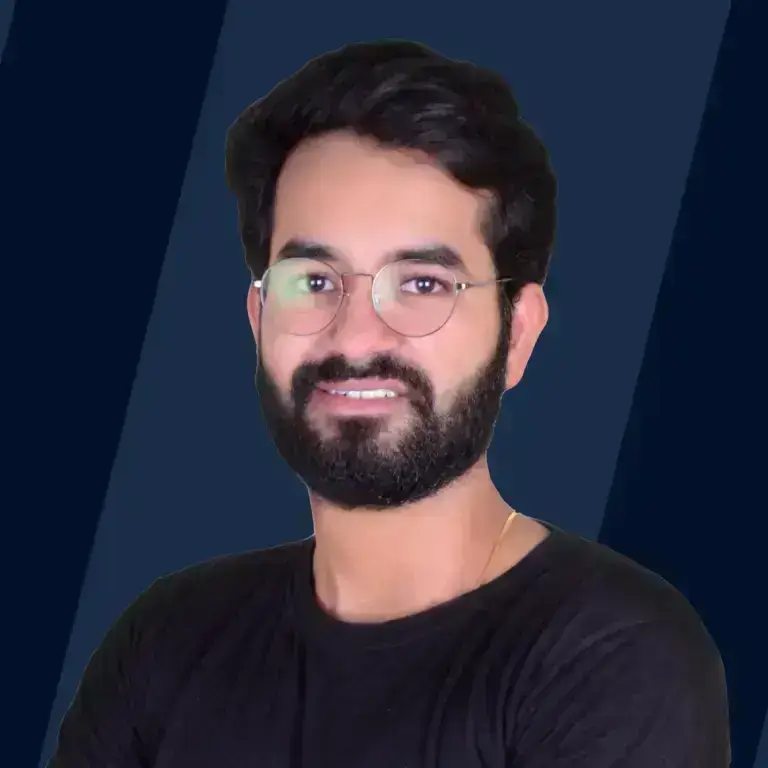
Overview
Collection Module in Python has various types of containers which are available to use easily by importing them into your program.
A Container is a type of object that can be used to hold multiple items while simultaneously providing a way to access and iterate over them, such as a Tuple or a list.
Introduction to Modules
The Modules in Python are simply the ".py" or python files that contain Python code that can contain code for specific inbuilt function and can be imported into some other Python program to make use of inbuilt functions easier.
A module can be thought as a code library or a file containing a group of methods and functions that you want to include in your python program. We can use modules to group together related functions, classes, or code blocks in the same file. As a result, splitting large Python code blocks into modules comprising code is regarded a best practise for building larger Python code.
Collection Module
The collection Module in Python has various types of containers which are available to use easily by importing them into your program using the collection module.
A Container is a type of object that can be used to hold multiple items while simultaneously providing a way to access and iterate over them, such as a Tuple or a list.
There are different containers which comes under collections module:
- namedtuple()
- OrderedDict()
- defaultdict()
- Counter()
- deque()
- Chainmap
Now lets talk about all these Containers in detail.
namedtuple()
The collections module's namedtuple() function is used to return a tuple with names for each of the tuple's positions. Each value of the tuple can be called using their corresponding names. Regular tuples have a difficult time remembering the index of each field of a tuple object. The namedtuple was created to address this problem, and it solves the index problem for regular tuple objects.
for importing and using the namedtuple() we use the below syntax:
Syntax:
After importing, you can simply just initialize the object and can use it according to the code.
namedTuple function takes the object name as its first parameter followed by the names as its positions. First define the structure of namedtuple() object using the namedtuple() function with names as its positions and initialize the tuple object by passing the values to the initialzed object.
Lets see some examples related to namedtuple() function.
Example 1:
First we will define the structure of namedtuple() object using the namedtuple() function with names as its positions.
We will initialize the tuple object by passing the values to the initialzed object.
Now you can use the initialised object to get the values by using the names as the tuple’s positions.
Output: w The program will print the values from the tuple object by using the names as their positions
Example 2:
In this example, we will make use of a list to create the tuple and we will access the values both by using indexes and the names.
using a list for creating the tuple helps us to access the values of the tuple by both using indexes and the names.
Output:
The program will print the values from the tuple object by using the indexes of the list passed and also by the names as their positions.
Using the indexes to get the values
Name is: ABC
Address is: Street No.1 ABC complex
Profession is: Teacher
Using the names to get the values
Name is: ABC
Address is: Street No.1 ABC complex
Profession is: Teacher
Simply printing the tuple
tuple1(name='ABC', address='Street No.1 ABC complex', profession='Teacher')
OrderedDict()
OrderedDict is a dictionary in which the keys are always inserted in the same order. The order of the keys in a dictionary is preserved if they are inserted in a specific order. Even if the value of the key is changed later, the position will not change.
So if we will iterate over the Dictionary it will always print out the values in the order they are inserted.
for importing the OrderedDict() we use the below syntax:
Syntax:
After importing, you can simply just initialize the object and can use it according to the code.
First define the structure using the OrderedDict() function and initialize the dictionary object by passing the values according to the keys.
Further you can use inbuilt function for dictionary like:
- d.clear()
- d.get(
[, ]) - d.items()
- d.keys()
- d.values()
- d.pop(
[, ]) - d.popitem()
- d.update(
)
Lets see some examples related to OrderedDict() function.
Example 1:
First we will define the structure using the OrderedDict() function and we will initialize the dictionary object by passing the values according to the keys.
Now you can use the dictionary to get the key-values.
We have simply initiated 4 keys and values in the ordered dictionary.
Output:
You can see that the order is preserved when we print the dictionary.
OrderedDict([('key1', 'value1'), ('key2', 'value2'), ('key3', 'value3'), ('key4', 'value4')])
Example 2:
In this example, we will make an OrderedDict and then we will change the key values but the original order will be maintained.
Changing the value of key "A" from 1 to 5 will not affect the ordering of the keys in the dictionary.
Output:
You can see that the order is preserved even after changing the key-value of the dictionary.
OrderedDict([('A', '5'), ('B', '2'), ('C', '3'), ('D', '4')])
defaultdict()
In Python, a dictionary is an unordered collection of data values that can be used to store data values in the same way that a map can. The Dictionary has a key-value pair and the key must be unique and immutable and it throws error when we try to access non-existent key. The only difference between defaultdict() and simple Dictionary is that when you try to access a non-existent key in defaultdict(), it does not throw an exception or a key error.
For importing defaultdict() we use the below syntax:
Syntax:
After importing, you can simply just initialize the object and can use it according to the code.
First define the structure using the defaultdict() function and initialize the dictionary object by passing the values according to the keys.
Further you can use inbuilt function for dictionary like:
- d.clear()
- d.get(
[, ]) - d.items()
- d.keys()
- d.values()
- d.pop(
[, ]) - d.popitem()
- d.update(
)
Lets see some examples related to defaultdict() function.
Example 1:
First we will define the structure using the defaultdict() function and we will initialize the dictionary object by passing the values according to the keys.
Now you can use the dictionary to get the key-values.
We have simply initiated 4 keys and values in the ordered dictionary.
Output:
This example simply prints the dictionary values.
defaultdict(None, {'k1': 'v1', 'k2': 'v2', 'k3': 'v3', 'k4': 'v4'})
Example 2:
In this example, we will try to access the key which is not present in the dictionary but the defaultdict will not give any key error.
defaultdict is advantageous in the conditions where inexisting keys are accessed as error does not occurs in those cases.
Output:
While initializing the defaultdict, if we don't pass an object reference such as int, bool, it will result in a key error and won't be able to deduce the fallback default value in such scenarios.
In this case, we have set default value as int so in case of missing key it will return 0.
You can see that when we tried to access the value 4 in the dictionary, it just returned 0 instead of returning KeyError.
0
Counter()
A counter is a built-in data structure that counts the number of times each value in an array or list appears.
Hashable objects are counted using the Counter subclass. counter() object constructs an iterable hash table when called.
For importing Counter() we use the below syntax: Syntax:
After importing, you can simply just initialize the Counter object and can use it according to the code. Lets see some examples related to Counter() function.
Example 1:
First we will initialize the structure using the Counter() function and we will pass the list in the counter object and a hashable object will be created
Now you can get the key-values of the hash.
Counter object makes a dictionary of all distinct keys and their counter values.
Output:
This example simply prints the counter object created. Counter({1: 5, 2: 2, 3: 2, 4: 1})
Example 2:
we will pass the list containing the string values and hence the dictionary of string as keys will be created.
Now you can get the key-values of the hash.
Output:
This example simply prints the counter object created. Counter({'a': 5, 'b': 4, 'c': 3})
deque()
Deque is a more efficient variant of a list for adding and removing items easily. It can add or remove items from the beginning or the end of the list.
It is a double ended queue which has insertion and deletion operations available at both the ends.
For importing deque() we use the below syntax:
Syntax:
After importing, you can simply just initialize the deque() object and can use it according to the code.
After importing, you can simply just initialize the object and pass the list in the deque object.
Further you can use inbuilt function for deque like:
- append(): append to right of deque()
- appendleft(): append to left of deque()
- pop(): pop an element from the right of the deque()
- popleft(): pop for the left of deque()
- index(ele, beg, end): get the index of the element from deque()
- insert(i, a): insert at a particular index of the deque()
- remove(): remove an element from deque
- count(): count occurences of an element in deque()
- reverse(): reverse the deque()
- rotate(): rotate the deque() by specified number. Lets see some examples related to deque().
Example:
First we will initialize the deque object and we will pass the list in the deque object.
Now we will be applying the removal and insertion on both the ends of the deque.
We added the items at both ends using append() and appendleft() function and then printed it.
Now finally we have removed the items from both the ends using pop() and popleft() function.
We have performed every above mentioned functions on our deque.
Output:
First simply a list is passed in the deque() function, Then we added the items at both ends using append() and appendleft() function and then printed it.
Now finally we have removed the items from both the ends using pop() and popleft() function.
Original Deque is: deque(['Hi', 'This', 'is', 'Scaler'])
Deque after adding to both the ends is: deque(['!!', 'Hi', 'This', 'is', 'Scaler', '!!'])
Deque after removal from start is:** deque(['!!', 'Hi', 'This', 'is', 'Scaler'])**
Deque after removal from beginning is: deque(['Hi', 'This', 'is', 'Scaler'])
Deque after insertion is: deque(['Hi', 'New', 'This', 'is', 'Scaler'])
Deque after removal of value New is: deque(['Hi', 'This', 'is', 'Scaler'])
count of Scaler in deque is: 1
Deque after reversing is: deque(['Scaler', 'is', 'This', 'Hi'])
Deque after rotation by 1 element is: deque(['Hi', 'Scaler', 'is', 'This'])
Chainmap
ChainMap returns a list of dictionaries after combining them and chaining them together. ChainMaps can encapsulate a multiple dictionaries into a single object or unit and has no limitations for the number of dictionaries to contain.
For importing Chainmap() we use the below syntax:
Syntax:
After importing, you can simply just initialize the Chainmap() object by passing the dictionaries you want to encapsulate.
Further you can use inbuilt function for chainmap like:
- keys(): it is used to get all the keys of chainmap
- values(): it is used to get all the values of chainmap
- maps(): it is used to get all the keys and their particular values in the object
- newchild(): It adds a new dictionary at the beginning of the chainmap
- reversed(): It is used to reverse the chainmap object.
Lets see some examples related to Chainmap().
Example:
First we will initialize the Chainmap object and we will pass all the dictionaries that we wanna encapsulate in the chainmap object.
Finally we will print the final chainmap object.
Output:
We Simply Just printed out the chainMap object after encapsulating the dictionaries to the Object.
ChainMap({'k1': 1, 'k2': 2, 'k3': 3}, {'k4': 4})
All the keys of the chainmap is: ['k2', 'k3', 'k1', 'k4']
All the values the chainmap is: [2, 3, 1, 4]
All the keys-value pairs of the chainmap is: [{'k1': 1, 'k2': 2, 'k3': 3}, {'k4': 4}]
All the keys of the chainmap is: ['k2', 'k3', 'k1', 'k4']
chainmap after addition at beginning is: ChainMap({'k5': 5}, {'k1': 1, 'k2': 2, 'k3': 3}, {'k4': 4})
Reversed chainmap is: [{'k4': 4}, {'k1': 1, 'k2': 2, 'k3': 3}, {'k5': 5}]
Conclusion
- The Modules in Python are simply python files that contain Python code that can contain code and can be imported.
- The collection Module in Python has various types of containers.
- There are different containers which comes under collections module:
- namedtuple()
- OrderedDict()
- defaultdict()
- Counter()
- deque()
- Chainmap
- The namedtuple() function from collections module is used to return a tuple with names for each of the tuple’s positions.
- OrderedDict() is a dictionary that always keeps the order in which the keys are inserted.
- defaultdict() dictionary is an unordered collection of data values that can be used to store data values in the same way that a map can.
- A counter is a built-in data structure that counts the number of times each value in an array or list appears.
- Deque is a more efficient variant of a list for adding and removing items easily. It can add or remove items from the beginning or the end of the list.
- ChainMap returns a list of dictionaries after combining them and chaining them together.