Python Keywords and Identifiers
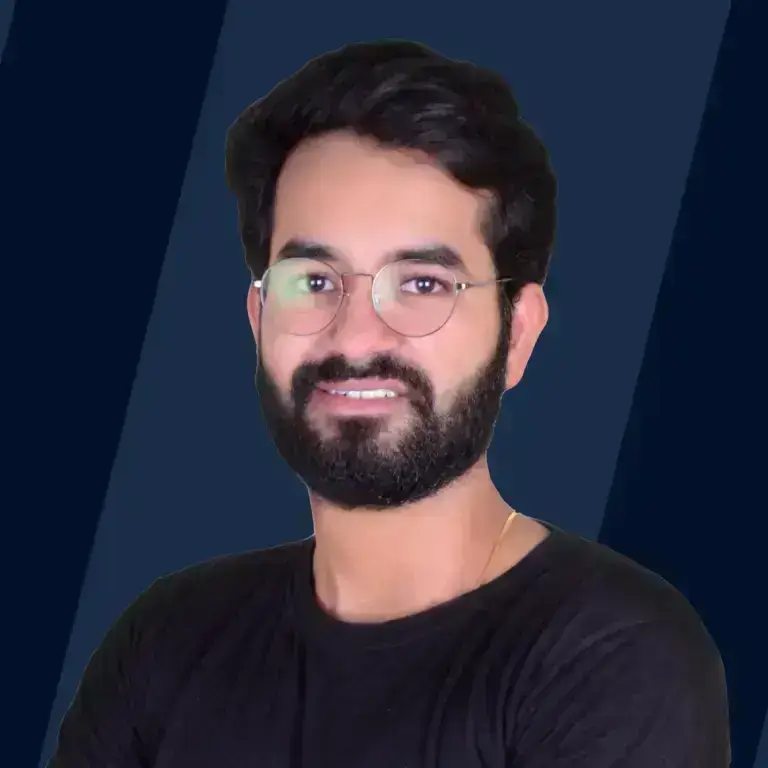
Keywords in Python are unique reserved words that cannot be used as a variable, function, or other identifier. These special words define the syntax and structure of the Python language. On the other hand, an identifier is a name used to identify entities like class, functions, variables, etc.
Python Keywords
In Python programming, keywords are reserved words that hold a specific meaning and are a part of the language's syntax. Understanding the rules governing using keywords is essential for writing syntactically correct and efficient Python code. Here are the key rules and guidelines related to Python keywords:
Rules for Keywords in Python
- Reserved Use: Keywords have specific, predefined uses and cannot be altered.
- Case Sensitivity: Python keywords are case-sensitive.
- No Identifier Usage: Keywords cannot be used as variable names, function names, or other identifiers.
- Fixed Number: The keywords are fixed and vary with Python versions.
- Non-Modifiable: Keywords are part of Python's syntax and cannot be modified.
- Contextual Keywords: Some words act as keywords only in specific contexts but can be used as identifiers elsewhere.
- Syntax Compliance: Keywords must be used according to Python's syntax rules.
List of Python Keywords
Keyword | Description |
---|---|
False | Represents the boolean value false. |
None | Represents the absence of a value or a null value. |
True | Represents the boolean value true. |
and | A logical operator used to combine conditional statements. |
as | Used to create an alias while importing a module. |
assert | Used for debugging purposes to check if a statement is true. |
break | Breaks out of the current closest enclosing loop. |
class | Used to define a new user-defined class. |
continue | Continues to the next iteration of the current loop. |
def | Used to define a function. |
del | Used to delete an object. |
elif | Used in conditional statements, same as else if. |
else | Used in conditional statements. |
except | Used with exceptions, what to do when an exception occurs. |
finally | Used with exceptions, a block of code that will be executed whether there is an exception or not. |
for | Used to create a for loop. |
from | Used to import specific parts of a module. |
global | Declares a global variable. |
if | Used to make a conditional statement. |
import | Used to import a module. |
in | Checks if a value is present in a list, tuple, etc. |
is | Tests object identity. |
lambda | Creates an anonymous function. |
nonlocal | Declares a non-local variable. |
not | A logical operator used to invert the truth value. |
or | A logical operator used to combine conditional statements. |
pass | A null statement, a statement that will do nothing. |
raise | Used to raise an exception. |
return | Used to exit a function and return a value. |
try | Makes a try-except statement. |
while | Used to create a while loop. |
with | Used to simplify exception handling. |
yield | Ends a function, returns a generator. |
Python Identifiers
In Python, identifiers are names used to identify a variable, function, class, module, or other object. They are essentially user-defined names to represent these entities in a program. The purpose of identifiers is to make the code readable and meaningful, as they can describe the role of the entity they are naming.
Rules for Naming Python Identifiers
-
Start with Letters or Underscore: Identifiers must begin with a letter (A-Z, a-z) or an underscore (_). They cannot start with a digit.
-
Case Sensitive: Python identifiers are case-sensitive. This means myVar, MyVar, and myvar are three different identifiers.
-
Contain Alphanumeric Characters and Underscores: After the first letter or underscore, identifiers can contain letters, digits (0-9), and underscores.
-
No Special Characters: Identifiers cannot contain special characters like @, $, !, etc.
-
Avoid Keywords: Identifiers should not be any of Python’s reserved keywords. For example, you cannot name an identifier if or for.
-
Length: There is no length limit for Python identifiers. However, keeping them within a reasonable length is good practice to maintain readability.
-
Conventions: Python follows certain naming conventions like using lowercase for variable names, uppercase for constants, and camelCase or snake_case for multi-word identifiers. Python does not enforce these, but they are good practices.
Examples of Python Identifiers
Valid Python Identifiers:
- Variables: height, max_value, _data
- Functions: calculate_area(), get_info()
- Classes: User, StockPrice
- Constants: MAX_LIMIT, PI
Invalid Python Identifiers:
- Starting with a digit: 1variable (invalid)
- Containing special characters: user-name (invalid)
- Using Python keywords: for, if (invalid)
These examples follow the naming rules and conventions for Python identifiers. Identifiers are a critical part of the code as they give meaning to the different parts of the program, making it easier to understand and maintain.
Python Keywords and Identifiers Examples
Let's go through some examples of Python keywords and identifiers:
Example 1: Use of async and await Keyword
Output:
Example 2: Use of from Keyword
Output:
Example 3: Use of is Keyword
Output:
Example 4: Use of import Keyword
Output:
Example 5: Use of finally Keyword
Output:
Example 6: Use of pass Keyword
Output:
Example 7: Use of assert Keyword
Output:
Example 8: Example of yield Keyword
Output:
Example 9: Use of global Keyword
Output:
Example 10: Use of del Keyword
Output:
Example 11: return Keyword
Output:
Example 12: lambda Keyword
Output:
Example 13: try, except, raise
Output:
Example 14: def, if, and else Keywords
Output:
Example 15: for, in, if, elif, and else Keywords
Output:
Example 16: break, continue Keywords and Identifier
Output:
Example 17: and, or, not, True, False Keywords
Output:
These examples provide a practical overview of how different Python keywords and identifiers are used in programming and their expected outputs.
Conclusion
- Python keywords are reserved words with specific, pre-defined meanings and roles in the language's syntax.
- Identifiers are user-defined names given to various programming entities like variables, functions, classes, etc.
- Keywords are case-sensitive and have rules governing their use, ensuring syntactical correctness in Python code.
- Identifiers also follow specific naming conventions, including starting with a letter or underscore, being case-sensitive, and avoiding using special characters and Python keywords.
- Practical examples of keywords and identifiers demonstrate their usage in real-world Python programming, providing insights into how these elements contribute to the language's structure and functionality.
- Understanding and correctly implementing Python keywords and identifiers is crucial for writing efficient, error-free, and readable Python programs.