What is Range Based for Loop in C++?
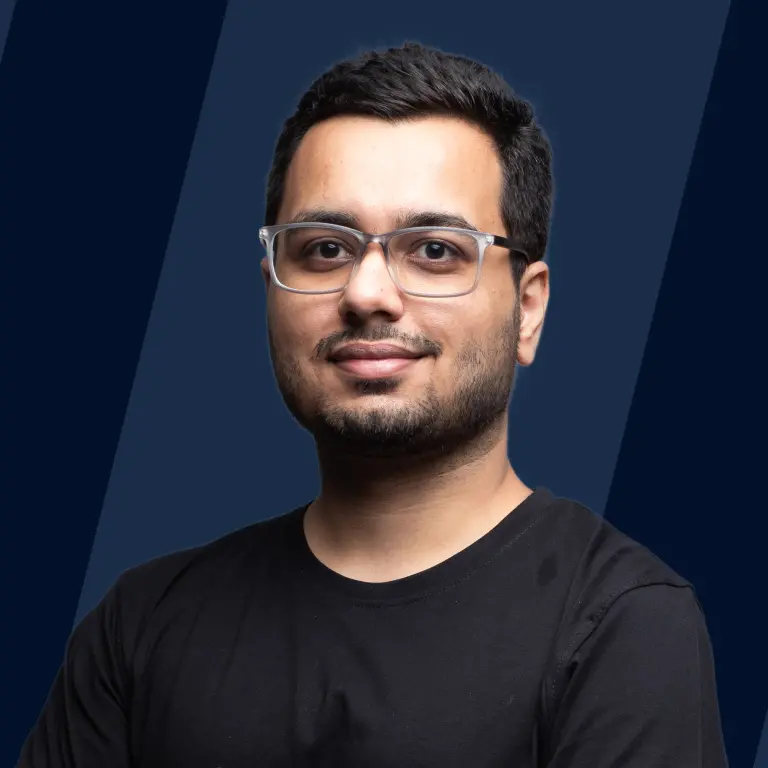
Introduction
Range-based for loop was a new addition to the C++ language introduced into its C++ 11 version. The intention was to provide an easy and simple syntax to work with the for loop compared to the traditional long syntax. It does not require lengthy operations to be listed in the loop syntax, and we need to bother about the number of elements in that data structure. All we need is an iterator of the type the elements are in the array, and we can iterate over the whole collection using that iterator only.
Syntax
The loop requires two terms to initiate the run: 1. iteratorVariable - a variable or an iterator, of the datatype same as the elements of the array or the collection. 2. theArray - the actual array, list, or the collection of elements that we have to iterate.
How to Print Each Element of an Array using a Range-based Loop in C++?
Syntax
Example
Let's see an example of how we can print each element of an array using the range-based for loop
Program for Range-based for loop C++
Output
Both the loops are printing the same thing, but it can be easily observed how convenient it has become with the range-based loop.
How to Print Each Vector Elements using a Range-based Loop?
Syntax
Example
Let's see an example of how we can print each vector element using the range-based for loop.
Program for Range-based for loop C++
Output
Here also, we can see how convenient it has become to iterate over a vector using a range-based loop.
What is a Nested Range-based for Loop in C++?
Description
Almost all of us have worked with nested loops, but mostly in those situations, we have been using the traditional for or while loops, but how to do the same thing using the range-based for loop? First of all, what is a nested loop? A nested loop means a loop inside another loop. One general example can be simply a 2D array, in which we have an array, which is an array of certain elements.
Let's look at the syntax first, then we will understand it with an example.
Syntax
Example
Program for Range-based for loop C++
Output
What is the Difference Between a Range-based Loop and a Standard Loop in C++?
There is almost no difference in the working of both the for loops, be it the traditional for loop or the range-based for loop. The only difference is in the syntax of how they are written.
The traditional for loop takes three parameters, the index variable, which will be used to iterate over the array or the collection, and the second loop termination condition, i.e., the condition specifying till what point the loop will keep getting executed. And the last parameter is the increment or decrement condition for the loop.
The range-based for loop only takes two parameters, the first being the iterator variable of the type same as the array type and the second being the actual array itself. The range-based for loop is slightly more convenient than its traditional version. Here, we need not worry about the termination condition, the array's length, increment or decrement operations, etc.
However, the range-based for loop also comes with certain shortcomings. Let's look at why we should and should not use the range-based for loop.
Advantages of Range-based for Loop
- Convenient and easy to use.
- We need not calculate the size or length of the array or the collection. The loop automatically handles that.
- If in some cases, we do not know the data type of the list or the collection of the elements, then we can use the auto keyword specifier, which will make the compiler auto-detect the should-be datatype and will not throw any error.
- Here, we do not need the termination condition and increment or decrement operations in the loop syntax.
- Works very effectively when we want to iterate through a complete array or a collection.
Disadvantages of Range-based Loop
- As mentioned above, the range-based for loop iterates over the whole array or the collection, meaning that, unlike traditional loops, we can not target any specific index or element. If it does so, then it will become very complex.
- The loop iterates over the whole array using the iterator, not the index, so skipping a value at any specific index or a group of indices can not be done.
- The array or the collection can not be reversely iterated directly using the range-based for loop.
Learn More
Learn More about Loops in C++
Conclusion
- Range-based loops in C++ are a convenient form of the traditional for loops. It was introduced in the c++ 11 standards and is available in later versions.
- The intention behind this was to provide an easy and simple syntax to work with loops and conveniently iterate over a group of elements.
- The range-based for loop only takes two parameters, the first being the iteratorVariable and the second being the array itself.
- It can be preferred over the traditional for loop for many reasons like no need for termination condition, collection length, manually doing increment or decrement operations, etc.
- But with all these advantages, it also comes with certain shortcomings. For example - targeting a specific element is not possible. The loop iterates over the collection completely, meaning we can not directly skip certain indices in the container as it uses the iterators to iterate the loop instead of indices, reverse printing of the elements can not be done easily, etc.