Styling in React Using CSS
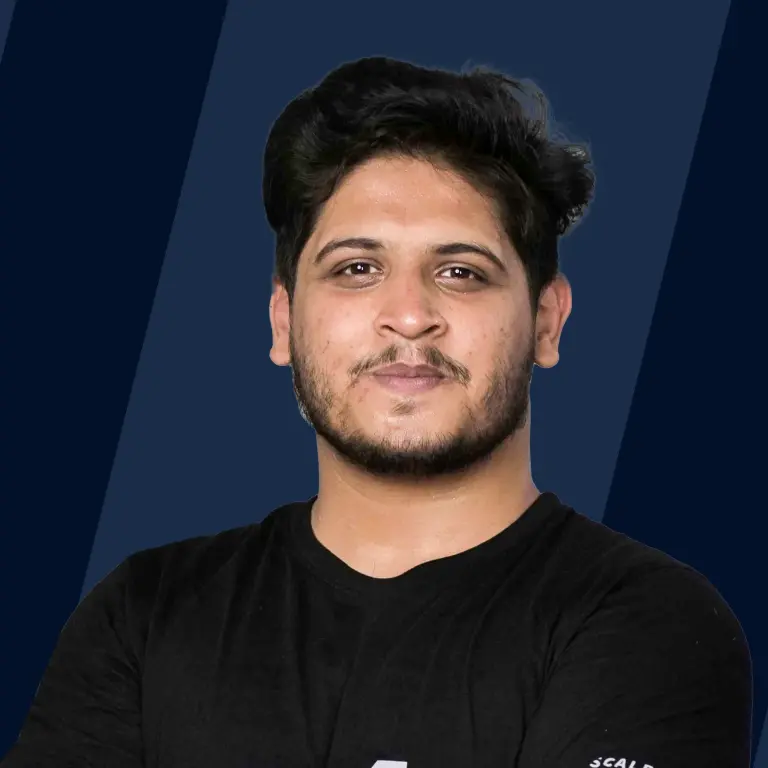
Overview
React is a popular library and the first preference for a majority of developers. Applications made with React require styling and we have a lot of options when choosing to style our react apps. We have inline styles, external CSS, SCSS, tailwind, etc.
To pick the best CSS react option a developer should have a basic understanding of the method. We will discuss these options in detail.
Introduction
When it comes to styling your react application, there is not any single preferred way. We have many CSS react options to choose from. We will discuss in detail the top options along with examples and the pros and cons of each of them.
Styling in react is similar to how to style normal HTML websites but there are a few changes as React uses JSX instead of plain HTML. So using CSS in JSX we have to follow its syntax. Don't worry we will take a close look at everything.
Inline Styling
Inline styling is a way to style the elements by writing the styles in the JSX itself. In HTML documents we used the style attribute to style the tags.
Inline styles are a direct way to style in react and for this type of styling, we do not need to create a separate stylesheet.
Also, the styles applied directly to the elements have higher precedence than styles contained in a stylesheet. This indicates that inline styles "override" any other style directives that might be used on the element.
Let's take an example to see how inline styles work:
Output
If you have styling in HTML documents then you might have some differences in the code we have written.
Remember that React uses JSX and not HTML. Inline styling in JSX is a little different, here are a few things we should keep in mind:
camelCased Property Names
In CSS if the property consists of two words then they are separated by a dash - but in JSX this is not the case. Example If we want to use the background-color property then we will use camelCase and it will look like backgroundColor
Here is a code example:
Here, observe the first div, we have used backgroundColor. The reason we have to use camelCase is that we are passing a JavaScript object to the element.
Let's talk more about objects.
JavaScript Object
The style attribute takes an object as an argument.
In JavaScript objects are written inside curly braces {} and to use JavaScript expression inside JSX we need a pair of curly braces {}. So, to use inline styles in JSX we have to write the style inside two curly braces {{}}
Since we have to pass objects to style components then we can pass objects instead of writing inline styles Here is how our code from the preivous example will look
At the first glance, you can see that this looks cleaner than inline styles. One other great thing is we can store all these objects in a separate file and can import objects from there.
Now its the time for pros and cons of Inline Styles
PROS
- Higher precedence
- Simple to use
- Suitable for small projects
CONS
- Code becomes unreadable
- Cannot use animations, selectors, etc.
- Not scalable
- Lot of code repetetion
CSS Stylesheet
In react we can use CSS stylesheets. CSS stylesheet is a file with a .css extension that stores all the styles. We can then refer to the styles from this file.
Suppose we have a CSS file with the name styles.css and we have defined a simple style inside it
Now we can use this in our App component
In JSX we do not use the class keyword as we do in HTML to refer to CSS classes because class is a reserved keyword in JavaScript, instead we use className to refer to CSS classes.
CSS Modules
A CSS Module is a CSS file in which all class names are scoped locally to a particular component. By default CSS class names are scoped globally. This can cause conflict, especially when the stylesheet is large. CSS modules solve this problem.
A CSS module is a .css file that is compiled. CSS modules generate styles dynamically for a particular component. When a CSS module is compiled it produces a CSS file where all the styles are present with new names and a JavaScript object is produced that maps the original CSS name with the new names.
React has out-of-the-box support for CSS modules so we do not have to make any modifications to use these.
Here is our CSS module file app.module.css
This is how we use CSS module in component
Notice how we use styles.mainDiv to access the CSS
Coming to Pros and Cons of CSS modules PROS
- Code is written like normal CSS
- No setup is required
- Clean code
- Locally scoped styles
CONS
- For Typescript we have to generate interfaces
- Tricky to reference classnames
Tailwind CSS in React
Tailwind CSS is a utility-first approach.
But what is a utility-first approach? This utility-first approach offers basic utility classes that enable us to create entirely custom designs without ever leaving your HTML. CSS.
Tailwind provides us with utility classes that we can directly use with JSX and thus we do not need to write any CSS. Tailwind is a full CSS framework that supports grids, flex, etc.
Tailwind is a third-party library, and using it with React requires a small amount of setup. To set up your react app with Tailwind please refer to the offical documentation
Now let's see how Tailwind looks in action
Output:
Although you must become familiar to tailwind classes, they are fairly comparable to standard CSS, so it won't take long to get used to them.
Tailwind comes with pre-configured classes. If we want to apply margin to a div then we do not write margin : 10px, instead we use tailwind class m-2 to apply a margin of 0.5rem. Similarly tailwind has classes for all CSS properties like p-2 for padding, margins, etc.
Coming to the Pros and Cons
PROS
- Smaller bundle size
- Don't have to remember class names
- Customizable
- Faster development
CONS
- Have to think according to Tailwind
- Requires initial setup
Sass in React
The website of Sass mentions CSS with superpowers, we will discuss why it is the case. Sass is an acronym for Syntactically Awesome Style Sheets. Sass is an extension of CSS. It adds additional features to CSS like variables, nesting and mixins.
To use Sass we simply have to install it as a dev dependency npm install sass --save-dev That's it, now we can import the Sass files directly into our react components.
Lets take a look a SCSS example
Here you can see that we have used &:hover inside .button In normal CSS we had to do something like
Thus SCSS really gives superpowers to CSS
Here comes the pros and cons PROS
- Features like nesting, variables, etc.
- Les boiler plate
- Reusable
CONS
- Styles are global
- Requires some setup and time to get used to
Different Ways to Write CSS in React
React is a versatile library and with its rising popularity, many ways of styling react apps have been developed.
We have looked at some of the ways by which we can style ours react applications. Let's look at some other ways to write CSS react.
Importing External Stylesheets
React being a frontend library naturally supports CSS files. In react we can simply import CSS files as we do in HTML.
Suppose we have a CSS file Home.css, we can import it into our react app like
Write Inline Styles
We have discussed inline style before in this article and discussed its pros and cons. With the knowledge of pros and cons now we can decide when to use inline styles.
Inline styles are the most simple way to add styles in React. Here is an example
If the number of properties increase then we can create objects and pass those objects in the style attribute.
Use CSS Modules
A CSS module stylesheet is similar to a normal stylesheet the difference being, all the class names are scoped locally. This means that we can use the same class name in multiple files. A CSS module file is scoped to that component only.
CSS modules are supported out of the box in react, the only change we have to do is to change the extension from .css to .module.css Here is an example
Use Styled-components
Styled components are a CSS-in-JSS approach to writing styles. A Styled component is a react component with styling applied to it. After creating a styled component we can simply import that component like we import other components in react.
Styled components is an npm package and we have to install it using npm npm install styled-components
Here is an example to use styled components
Styled components use special syntax with backticks to write CSS in javascript and thus can be used for css react. These components require some time to get used to. Things like handling props require learning the syntax.
Conditional Styling
Since styled components are react components themselves this means that they are functional, which means that we can use props within CSS.
Lets look at an example
Conclusion
This article discussed the various ways to style our react apps. We have also talked about the pros and cons of each of them.
Here is a quick summary
- Styling depends on the dev team's preference and the requirement of the application.
- Inline styling refers to writing CSS inside the JSX of react elements.
- This requires us to write CSS property names in camelCase format
- The styles are passed as a JavaScript Object
- CSS Stylesheet is a file with a .css extension that can be directly imported into a react component
- CSS modules are CSS files with .module.css extension. CSS modules scope the variable names locally instead of globally.
- Tailwind CSS is the utility's first approach to write style react components. It does not require writing CSS but it uses its classes.
- SASS stands for Syntactically Awesome Style Sheets, it provided additional features to CSS like nesting, variables, and mixins.
- Styled component is a library that creates react components that have styles applied to them. Styled components support conditional styling.