Read JSON File in Javascript
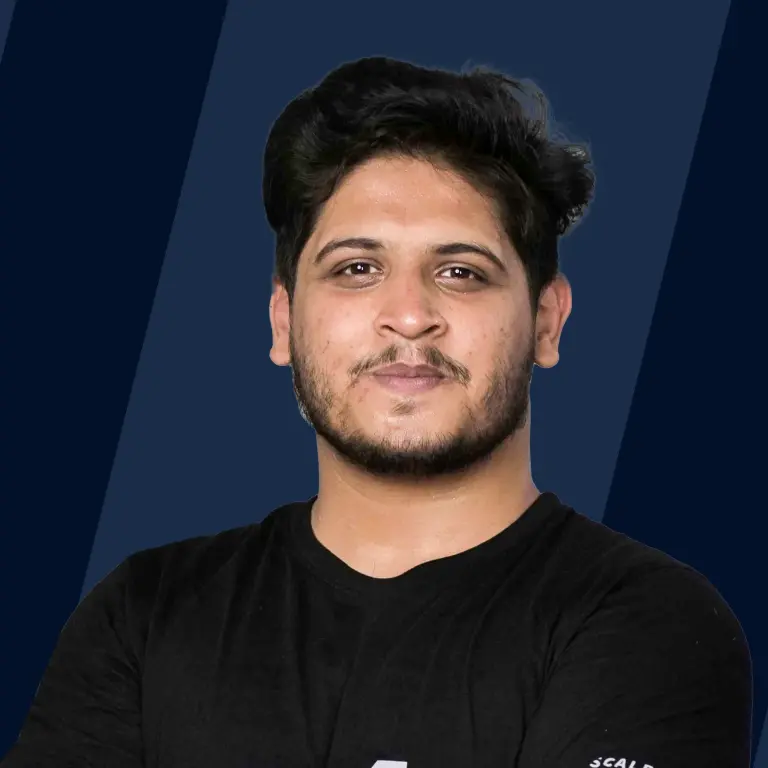
Overview
JSON also known as JavaScript Object Notation is a basic or standardized design used for the transportation and storage of data. It is a plain text written in JavaScript Object Notation which is language-independent. It is a lightweight data-interchange format. JSON is a self-describing format and it is easy to understand.
We can read JSON files in JavaScript using the following methods:
-
Using modules (NodeJs runtime environment) Here we will fetch the data inside the JSON file and execute our JS file in the NodeJs runtime environment using the required modules without using the web browser.
-
Using ES6 module (Web Runtime Environment only) Here we will fetch the data inside the JSON file and execute our JS file in the browser's runtime environment using the ES6 import module.
Introduction
Before learning about how we can read JSON files in JavaScript, let's learn a bit about JavaScript and JSON.
JavaScript is an object-oriented programming language used on web pages. JavaScript is the web programming language used on both the client and server sides to provide dynamic and interactive behavior in a webpage. It is a lightweight and easy-to-understand programming language. JavaScript is the most popular programming language in the world. It is a scripting language of a webpage that can be used even by non-browser environments like NodeJs, Adobe Acrobat, etc. Languages like HTML and CSS are used to provide structure and styling to a webpage respectively whereas JavaScript engages users by providing interactiveness to a webpage.
Now, let's learn about JSON in detail.
JSON is an open standard file and data interchange file format commonly known as JavaScript Object Notation which is readable by both machines and humans. Previously, XML was used to store data but was less human-readable and also not as user-friendly as JSON. That is why JSON is used as it is easy and fast to access and also manipulate as it only contains texts. JSON is a file format that stores data in the format of key-value pair which is similar to the object properties of JavaScript. The syntax for writing the key-value pair in a JSON file is writing them inside double quotes separated by a colon(:).
Now, let's have a look at how JSON is related to JavaScript to get a more clear understanding of the article.
As discussed earlier, JSON is called JavaScript Object Notation. It is called so because the JSON is syntactically similar to the code for the creation of a JavaScript Object. Syntactically, both JSON and JavaScript Objects are identical, but in terms of keys they are different as in JavaScript objects the keys are not written inside quotes in the form of a string. JavaScript programs can convert a JSON data file to fundamental JavaScript Objects without any discomfort.
Now, Let's Understand Why We use JSON
We know the fact that JSON is a preferred way of formatting and storing data but why is it so?
Let's understand with an example:
As we know, a variable is declared and when assigned a value to it, it's not the variable that contains the value rather the variable just contains the address of the memory space where the value is stored. When we have to transfer the same data to use somewhere else just like an API (Application Programming Interface) then one way to send the data is we have to transfer the entire system memory along with the address of the data which is required. The process of sending your system's entire memory is not at all safe. Now, JSON comes into the picture, JSON serializes the data to be transferred and converts the data into an easy and human-readable format which also makes it to be able to communicate and transferable.
Now, let's get back to the topic which is how to read JSON files in JavaScript. This can be done through:
- Using required modules (NodeJS Runtime environment)
- Using ES6 import module (Web Runtime environment only)
These methods have been explained using examples. Please refer to the next section.
How to read JSON files in javascript?
Let's discuss the following methods to understand the concept of reading a JSON file in JavaScript.
Method 1. Using Required Module
Let us consider we have a sample JSON file named config.json which contains the data of students that exists in the same directory of files where we have the JavaScript file. It contains the following set of data:
We can read or retrieve the data inside the JSON file by using the required modules. Here, we are working on our JS file in the NodeJs environment without using a browser.
The code used inside the JS file to retrieve or read the data inside the JSON file is as follows:
Explanation Here in the above program, we have initialized a variable dataJson using the require() module. In NodeJs, require() is a built-in function to include external modules that exist in separate files. In this way, we have included the external JSON file config.json so that we can read the JSON file, and then we have used console.log(dataJson) to show the data inside the JSON file.
Method 2. Using ES6 Import Module (Web Runtime Environment)
Let us consider, that we have the same JSON file we have used in the previous method which contains the data of students. It contains the following set of data:
We can retrieve or read the data inside the JSON file by using the ES6 (ECMAScript 6) import modules. Here, we are running our JS file in the browser runtime environment. Use this Online JavaScript Formatter to format your code.
The code is written inside the HTML file:
The code used inside the JS file (test.js) to retrieve or read the data inside the JSON file is as follows:
Output The output will be the data stored inside the JSON file.
Explanation Here in the above program, we have imported a variable dataJson using the import module. In JavaScript, import is a module used to import external packages that exist in separate files. In this way, we have imported the external JSON file config.json so that we can read the JSON file.
We can use the above two methods to retrieve or read the data stored inside any JSON file in JavaScript.
Conclusion
- JSON is an open standard file and data interchange file format commonly known as JavaScript Object Notation which is readable by both machines and humans.
- JSON is a file format that stores data in the format of key-value pair which is similar to the object properties of JavaScript.
- JSON files can be validated by a JSON validator.
- JSON is syntactically similar to the code for the creation of JavaScript Objects.
- JavaScript program can convert a JSON data file to fundamental JavaScript Objects without any discomfort.
- JSON serializes the data to be transferred and converts the data into an easy and human-readable format which also makes it to be able to communicate and transferable.
- JSON is a self-describing format and it is easy to understand.
- The syntax for writing the key-value pair in a JSON file is writing them inside double quotes separated by a colon(:).
- In JavaScript, we can read JSON files using the following methods:
- Using modules (NodeJs runtime environment)
- Read the data inside the JSON file in the NodeJs runtime environment using the required modules without using the web browser.
- Using ES6 import module (Web Runtime Environment)
- Read the data inside the JSON file in the browser's runtime environment using the ES6 import module.
- Using modules (NodeJs runtime environment)