Read JSON File in Python
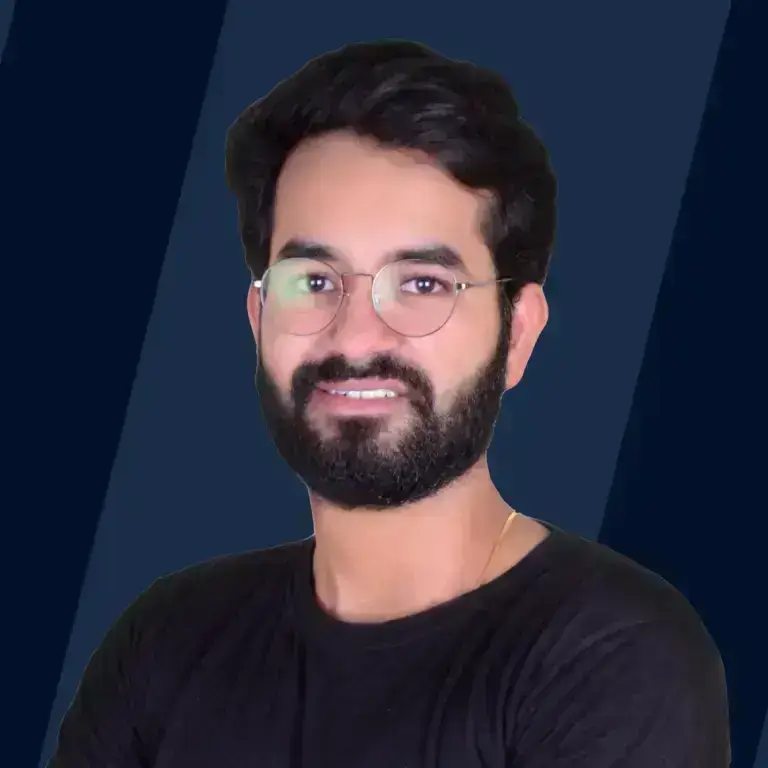
Overview
JSON (JavaScript Object Notation) is a language-independent data format used to store or represent structured data. A web application and its server can transmit and receive data using the JSON format. Python supports JSON through a built-in package named JSON. To use this functionality, we have to import the JSON package into our python program.
Reading from JSON in Python
Python provides a built-in JSON package, which is used to work with JSON (string, or file containing JSON object). This JSON module provides us with a lot of methods to perform operations such as parse, read and write, etc among which loads() and load() methods are widely used to read JSON files in Python.
In Python, JSON is stored as a string.
Example:
The conversion of JSON objects into their respective Python objects is known as Deserialization. We can use load() or loads() method from the python in-built JSON module to deserialize the JSON objects and read JSON file in Python.
The below-given table shows JSON objects and their equivalent Python objects:
JSON Object | Python Equivalent |
---|---|
object | dict |
array | list, tuple |
string | str |
number | int, float |
true | True |
false | False |
null | None |
Importing JSON Module in Python
To read JSON file in python, we need to import the json module before we can use it. json module makes it easy to parse JSON strings and files containing JSON objects.
Parsing refers to the process of reading data (in formats like strings), analyzing it, and drawing useful inferences from it.
The text in the JSON file is stored using quoted strings which contain the data in key-value pair mapped within {}.
Examples of Reading JSON File in Python
Example 1 : Using json.load() method
json.load(): This method accepts a file object, parses the JSON data, creates a Python dictionary with the data, and returns it back.
Syntax:
Suppose, we have a file named example.json which contains the following JSON object
Code:
Below is the implementation of how to read JSON files in python.
Code:
Output:
Explanation:
In the above program, we used the open() function in python to read JSON file and then parsed the file using json.load() method which gives us data which is of the type dictionary.
If you do not know how to read and write files in Python, you can check How to Read a File in Python.
Example 2: Using json.loads() method
json.loads(): This method does not take the file path, but the contents of a string file as a Python string object and converts it to a python dictionary.
Syntax:
The below program shows how to read JSON files in python from both string and JSON files.
Code:
Output:
Example 3: Pretty Print JSON in Python
json.dumps(): this method converts a Python object into a JSON string.
Syntax:
After learning how to read json file in python, we have to analyze and debug JSON data, and for this we should print it in a more readable format. This can be done by passing additional parameters indent and sort_keys in json.dumps() method.
Code:
Output:
In the above program, in json.dumps() method we have set indent=4, which means 4 spaces of indentation, and sort_keys=True, which means keys are sorted in ascending order.
The default value of indent is None and sort_keys is False.
Conclusion
- JSON stands for JavaScript Object Notation, and is a file format that stores data. This format helps in storing data and enables communication with servers during deployment
- The conversion of JSON objects into their respective Python objects is known as Deserialization.
- Parsing refers to the process of reading data (in formats like strings), analyzing it, and drawing useful inferences from it.
- We use load() or loads() method from the in-built json module to deserialize the JSON objects and read json file in python.