Recursion in Javascript
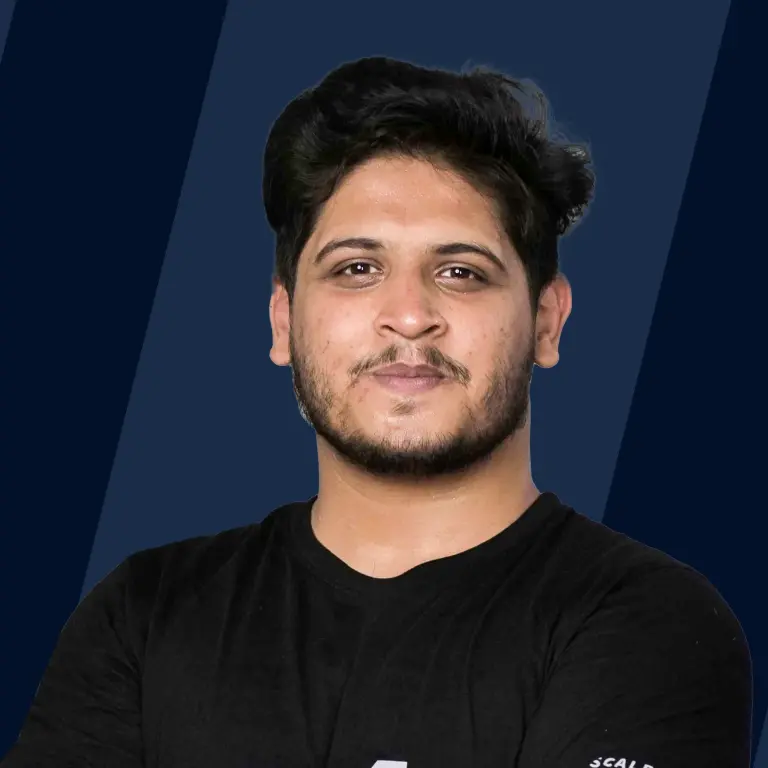
Recursion in JavaScript involves a function calling itself, repeatedly executing until it reaches a base case, akin to a loop. It's a powerful technique for solving problems where solutions depend on smaller versions of the same problem. For instance, in the Fibonacci sequence, each number is the sum of the two preceding ones, defined recursively as F(i) = F(i-1) + F(i-2).
Syntax for Recursion in JavaScript
Consider Below the syntax of the recursive function in JavaScript:
We used recursion() as the recursive function in the above example. In this case, the recursive function is recursion() . In the function, it makes a call to itself until the base condition is met and it stops executing. The second recursion function that occurs outside of the function code takes arguments and only calls the recursive function once.
The Three Parts of a Recursive Function
The recursion in JavaScript works the same as in any other language. Let's analyze the syntax structure shown in the recursion function earlier. While writing a recursive function(), you must have the following three basic components of that syntax:
- The Function Declaration.
- The Base Case.
- The Recursive Call command.
Let’s understand these three fundamental components.
1. The function Definition
This is where the intended recursive function is declared. The process is similar to how a new function is normally declared in JavaScript.
2.The Base Condition
The base case is the most critical component of each recursive function. When the problem is divided into smaller jobs, it is the smallest component of the problem to be solved. It can also be seen as the command that, if the set condition is true, it can end the recursion process.
3. The Recursive Call
The Recursive Call command in recursion is only responsible for triggering a new recursive call. The Recursive Call command is the actual command that addresses the main problem that you want to solve.
Using if...else statement to prevent infinite recursion
To prevent infinite recursion in javascript, you can incorporate an if...else statement within the recursive function to establish a base case where the function stops calling itself. This base case serves as a condition that halts the recursive calls, ensuring the termination of the recursion. For example, in a recursive function calculating factorial:
In this implementation, the base case checks if the input 'n' is equal to 0 or 1, in which case the function returns 1, halting further recursive calls. Otherwise, the function calls itself with 'n-1' until the base case is met.
How do you define a recursive function?
Defining a recursive function involves writing a function that calls itself within its code. To create a recursive function in JavaScript, follow these steps:
Define the base case(s): Identify the condition(s) under which the function should stop calling itself and return a specific value. This prevents infinite recursion.
Write the recursive case: Define the logic for calling the function recursively, typically with modified arguments or conditions, moving closer to the base case(s) with each recursive call.
What is a base condition, and Why do you need It?
A base condition, also known as a base case or termination condition, is a critical aspect of recursion. It is the stopping criterion for recursive functions, preventing infinite recursion and ensuring termination. Base conditions define scenarios where the function should stop calling itself and return a result, effectively breaking the recursive chain. Recursive functions would endlessly call themselves without base conditions, leading to stack overflow errors and incorrect results.
Recursion vs Loops
Recursion and loops are control flow mechanisms used in programming but with distinct characteristics.
recursion in javascript involves a function calling itself, repeatedly executing until a base case is reached. It's elegant for solving problems where solutions depend on smaller versions of the same problem. Conversely, loops iterate over a block of code until a condition is met, providing a more straightforward approach for repetitive tasks. While recursion can be more concise and expressive for specific problems, loops are often more efficient in performance and memory usage. Choosing between them depends on the specific requirements and constraints of the problem.
Examples of a recursive function
Consider the examples below to better understand how recursion in javascript works.
Print Numbers
Output
Explanation:
- If the input number is strictly greater than 1, the recursive function calls itself again by reducing the value by 1.
- it will print the number in descending order; in a similar way, we can print the numbers in an ascending way.
Find Factorial
Output
Explanation:
- Function factorial() will recursively call itself by decreasing the number when you call it with a positive integer. This cycle is repeated until the number becomes 1.
Computing Factorial Using Recursion
To compute the factorial of a number using recursion in Python, you can define a function that calls itself until it reaches the base case where the factorial of 0 or 1 is 1. In the above section, we have seen how to compute the factorial of a number in a recursive way. Now, let's see the process of computing the factorial.
image reference for showing the process
The above image illustrates the recursive function's iterative process, depicting how each function call invokes itself until reaching the base case, visually capturing the execution flow.
Conclusion
- A function that calls itself is recursion until it doesn’t (Base case).
- The base case is the smallest condition of the initial problem to be solved.
- Recursion in javascript is a technique for solving problems when the result depends on solutions to the lesser versions of the same problem.
- Recursion uses functions that call themselves from within their code to solve such recursive problems.
- there must be a condition for a recursive function to stop calling itself. Otherwise, the function gets invoked and called infinitely.
- We have discussed in detail how recursion in javascript works with the help of various examples.