What are Relational Operators in C++?
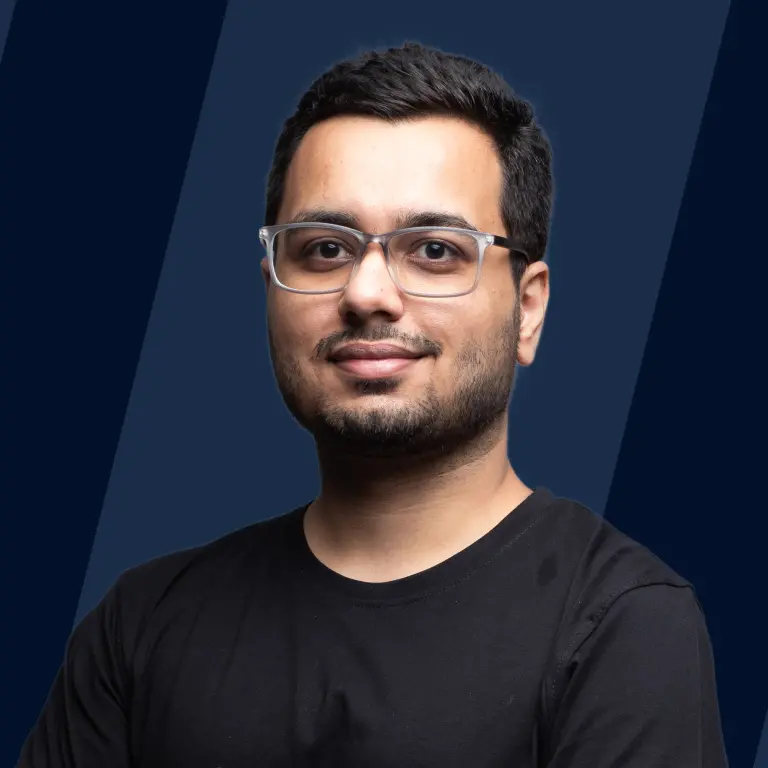
In a program, there can be cases where we are required to compare between the operands and their values. Arithmetic or unary operators are useless in such circumstances. Hence, special operators called Relational Operators are employed in this situation.
Relational operations are a notion in computer science that specifies the relationship between two or more entities in a program. Relational Operators establish this relationship when comparing two or more numerical values recorded in an operand, whether they are numerically equal, unequal, greater than or less than, etc.
Relational operators look for connections or relationships between two or more operands or entities. They are important for decision-making.
Two operands are required when we use relational operators and hence they are binary operators. Also, they have Left to right associativity. When two operators with the same precedence are next to one other, left to right associativity states that the leftmost operator is evaluated first.
Syntax of Relational Operator in C++
The syntax of a relational operator in C++ is:
Where operand1 and operand2 can be variables or numerical values (constants). Operator refers to a relational operator. There are two operands involved because relational operators are binary. Moreover, the above format where the operator is represented between the two operators is called the infix format.
What is the Result of A Relational Expression in C++?
After executing the relational operation, i.e., comparison, the relational operator returns a value for the Boolean data type. Only one of two true or false values may be found in Boolean Datatype values.
How Does a Program Treat True and False?
Relational operators in programming languages like Java and Pascal yield a True or False result based on the dependencies between the operands. Relational operators in the C and C++ programming languages return an integer value of 0 or 1, where 0 denotes False and 1 denotes True.
Types of Relational Operators in C++
C++ offers six relational operators. They include:
- Greater than (>)
- Less than (<)
- Greater than or equal to (>=)
- Less than or equal to (<=)
- Equal to (==)
- Not equal to (!=)
Greater Than (>)
This 'greater than' operator checks if the value of the first operand is greater than the value of the second operator or not. The symbol for this operator is ‘>’. If the first operand is greater than the second, the operator will return 1 (true) else 0 (false).
Output:
Less Than (<)
The 'less than' operator checks if the value of the first operand is less than the value of the second operator or not. The symbol for this operator is ‘<’. If the first operand is less than the second, the operator will return 1 (true) else 0 (false).
Output:
Greater Than or Equal To (>=)
This operator checks if the value of the first operand is greater than or equal to the value of the second operator or not. The symbol for this operator is ‘>=’. If the first operand is greater than or equal to the second, the operator will return 1 (true) else 0 (false).
Output:
Less Than Or Equal To (<=)
This operator checks if the value of the first operand is less than or equal to the value of the second operator or not. The symbol for this operator is ‘<=’. If the first operand is less than or equal than the second, the operator will return 1 (true) else 0 (false).
Output:
Equal To (==)
This operator checks if the value of the first operand is equal to the value of the second operator or not. The symbol for this operator is ‘ == ’. Note that whereas the symbol ‘=’ is used for equality generally, in computer science, ‘=’ is used to assign a value to a variable and hence called the assignment operator, and == is used to check whether the two operands are equal or not. In javascript, further a symbol ‘===’ is used to also check for the type of the operands. However, we focus on C++ in this article. If the first operand is equal to the second, the operator will return 1 (true) else 0 (false).
Output:
Not Equal To (!=)
This operator checks if the value of the first operand is NOT equal to the value of the second operator. The symbol for this operator is ‘!=’. This operator works opposite to the ‘==’ operator. If the first operand is not equal than the second, the operator will return 1 (true) else 0 (false).
Output:
Relational Operators in C++ Precedence and Relationship to The Other Operators
All operators in C++ are assigned a precedence rank in a particular order. This order is required because if more than one type of operator is present in a calculation without specifying any brackets, this order will determine which operator will perform its function first (or precede other operators). This is similar to the BODMAS rule in Mathematics. BODMAS is a precedence order for mathematical operators, standing for “Brackets, Of, Division, Multiply, Add, Subtract”, stating that the operators coming before in this rule have higher precedence than the others Let’s see where the relational operators lie in this precedence order. The precedence order is as follows:
Symbol | Type |
---|---|
) | Brackets |
*, /, % | Arithmetic |
+, - | Arithmetic |
>, >=, <, <= | Relational |
==, != | Relational |
=, *=, /=, %=, +=, -= | Assignment |
Relational Operators have higher precedence than the assignment and mathematical operators but less than arithmetic operations. Also, note that less/greater than operators have higher precedence than equal to/not equal to operators. Let’s go through an example to understand how this precedence works in a C++ code:
Output:
Here, in the expression , there are two types of operators present: arithmetic and relational operators. Out of both, arithmetic operators have higher precedence, hence first they will perform their intended function, i.e and are calculated first. Given the values of a and b as 5 and 10, the calculated values come out to be 15 and 2, now the calculation will be performed, and the greater than relational operator outputs 1 (true) for this case. We can also say that the above expression is equivalent to because the expressions in the brackets are calculated first.
Other Examples
Comparing Characters with Relational Operators
Computers work on the binary language. Each and every number, be it an integer, or float or a double, is stored in binary format in the memory. Also, each different possible character possible in the character set of the language is assigned a unique numerical code which is also stored in the binary format. Hence, because the characters are also stored as numbers in the memory, relational operators can be used to compare characters (their numerical codes, actually). How does this help? Because the codes for the english alphabet (both lowercase as well as uppercase) and numerical characters are stored in incremental order, the same as their actual order. Hence, for example, will return a 1 (true). For characters ‘a’ to ‘z’ , their integer values range from and for ‘A’ to ‘Z’ range, from . So is true and is false. The above logic is illustrated in the below code:
Output:
Comparing Strings with Relational Operators in C++
Strings can also be compared using relational operators. The reason behind this is the same with which we were able to compare characters: the integer value of characters! The integer value of each character of the left string is one by one compared to the corresponding character of the right string from left to right. So in a way, this is an iterative character comparison only. If a string is less than another using relational operators, this means that a character of the left string is less than the corresponding character of the right string (i.e., at the same position), and all characters before that character are equal). It can also be said that the first string is ‘lexicographically’ smaller than the right string. The above logic is illustrated in the below code:
Output:
Conclusion
In this article, we discussed:
- Relational operators are used to comparing variables or constants
- They output boolean values, true or false, or 1 or 0 equivalently in C++
- They are binary operators and require two operands
- There are six types of relational operators in C++
- ‘==’ is an equality operator while ‘=’ is an assignment operator
- The precedence of relational operators in C++ is less than arithmetics operators but more than assignment operators
- Within the relational operators in C++, the greater or less than operators have higher precedence than equal/not equal to operators
- Relational operators in C++ can also be used to compare characters as well strings.