Remove Whitespace From String in Java
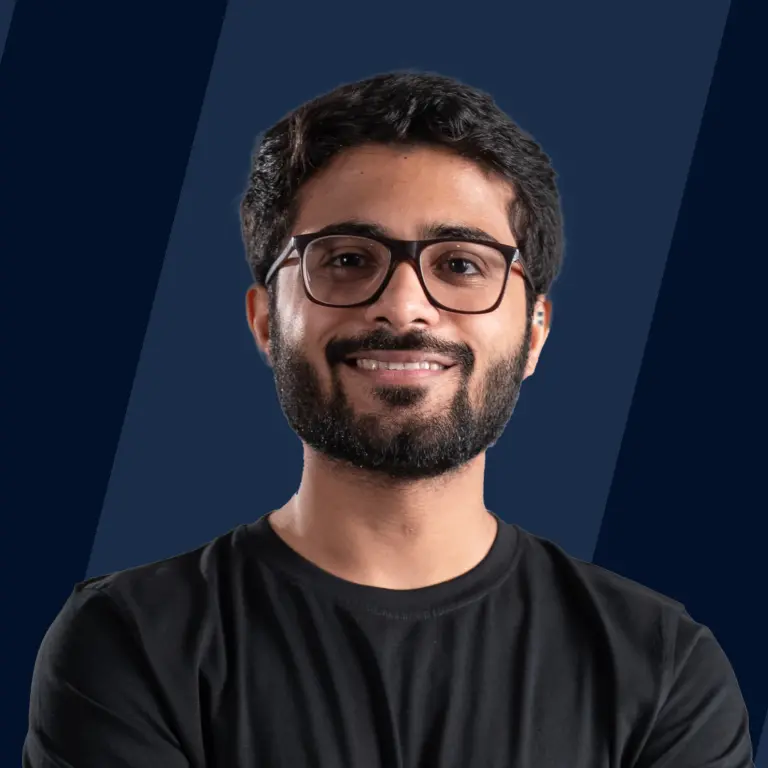
Overview
In Java, removing whitespace from a String is a common need when manipulating strings. This article will discuss eliminating all or a portion of the existing whitespace in the string. We'll look at numerous built-in Java ways to accomplish the same thing. Let's explore common scenarios of removing whitespace from a String in Java.
Introduction
Suppose you have to display a list of strings, but each of these strings has some spaces at the end, added mistakenly. This needs to be removed before displaying. Likewise, there can be spaces in between or at the beginning of the strings.
We shall be looking at Java programs to remove these spaces. Also, Java provides numerous in-built methods to perform this task. To list a few:
-
trim(): It removes the leading and trailing spaces present in the string. You can see this in the image below:
-
strip(): It removes the leading and trailing spaces present in the string. Also, it is Uniset/Unicode character aware. We shall learn more about it in a section ahead.
-
stripLeading(): It removes the spaces present at the beginning of the string.
-
stripTrailing(): It removes the spaces present at the end of the string.
-
replaceAll(): It replaces all the matched substrings with a new string. We shall be discussing the methods along with the implementation ahead.
trim() method in Java
This is the most common method to remove white spaces present at the beginning and end of the string. For trim() method, space character is a character having ASCII values <= 32 (‘U+0020’) .It checks for this Unicode value at the beginning and end of the string. If present, it removes the spaces and returns the modified string.
Signature: trim() method is defined as:
Syntax: trim() method is written as
Parameters: trim() method takes no parameters.
Return Values: It returns the modified version of the original string, with no spaces at the end and beginning.
Exceptions: It throws
- NullPointerException: If the string on which method is called is null.
Output:
Explanation: In the above program, the value of the string is null; hence exception is thrown.
Let us look at the correct implementation. Program to Remove Leading and Trailing Whitespaces
Output:
Explanation:
The trim() method is used in the program above to trim the strings s and s1. In contrast to s1, which contains numerous spaces, s only has one space at the beginning and one at the end. These spaces are eliminated once the method trim() is called and the output is printed.
strip() method Java 11
The strip() method was introduced in Java 11. It also removes leading and trailing spaces present in the string. But that is exactly what trim() does. What's the difference between the two?
strip() method is Unicode aware, a modification over trim().The reason behind the addition of this method is that there exist numerous space characters as per the Unicode standards having ASCII value > 32(‘U+0020’); the strip() method can remove them as well.
trim() | strip() |
---|---|
It was introduced in Java 1. | It was introduced in Java 11. |
It makes use of ASCII values. | It makes use of the Unicode value. |
It removes space characters with ASCII values less than or equal to 32(‘U+0020’) | It removes all the space characters as per the Unicode standards having ASCII value greater than 32(‘U+0020’) |
Signature: strip() method is defined as:
Syntax: strip() method is written as
Parameters: strip() method takes no parameters.
Return Values: It returns the modified version of the original string, i.e., with no spaces at the end and beginning.
Exceptions: It throws
- NullPointerException: If the string on which method is called is null.
Output:
Explanation: In the above program, the value of the string is null; hence exception is thrown.
Let us look at the correct implementation and understand the difference between the two. Program to Remove Leading and Trailing Whitespaces
Output:
Explanation:
In the above program, we have appended a whitespace character with ASCII value: ‘U + 2003’. So as you can see, the trim() method does not remove it as the value is not less than 'U+0020'. Whereas in the case of strip(), it gets removed. This pretty much explains the advantage of strip() over trim().
stripLeading() method Java 11
stripLeading() method removes all the leading spaces or the spaces present at the beginning of the string. It was introduced in Java 11. Just like strip(), it can remove the space characters space characters as per the Unicode standards having ASCII value greater than 32(‘U+0020’).
Signature: stripLeading() method is defined as:
Syntax: stripLeading() method is written as
Parameters: stripLeading() method takes no parameters.
Return Values: It returns the modified version of the original string, i.e., with no spaces at the beginning. Exceptions: It throws
- NullPointerException: If the string on which method is called is null.
Output:
Explanation: In the above program, the value of the string is null; hence exception is thrown.
Below is the correct implementation:
Program to Remove Leading Whitespaces
Output:
Explanation:
In the above program, the stripLeading() method is called on two strings. One of these has a space character with a codepoint value > 32(‘U+0020’). As you can see, in both cases, the spaces present at the beginning get removed; however, the ones at the end are still present.
stripTrailing() method Java 11
This method is exactly the opposite of the stripLeading() method. It removes all the trailing or the spaces present at the end of the string. It was also introduced in Java11. See the image below for reference:
Signature: stripTrailing() method is defined as:
Syntax: stripTrailing() method is written as
Parameters: stripTrailing method takes no parameters.
Return Values: It returns the modified string with no spaces at the end.
Exceptions: It throws
- NullPointerException: If the string on which method is called is null.
Output:
Explanation:
In the above program, the value of the string is null; hence exception is thrown.
Below is the correct implementation.
Program to Remove Trailing Whitespaces
Output:
Explanation: In the above program stripLeading() method is called on two strings. One of these has a space character with a codepoint value > 32(‘U+0020’). As you can see, in both cases, the spaces present at the end get removed. However, the ones at the beginning are still present.
replaceAll(String regex, String replacement)
replaceAll() is a very commonly used method for string manipulation in Java. This method replaces all the substrings of the string matching the specified regex expression with the specified replacement text.
A regular expression or regex is a string pattern that we use for some searching or matching kind of operations.
We can use this method to remove spaces from the string. All we need to do is specify the regex expression for the kind of spaces that need to be removed, i.e., trailing or leading, or both. The following table shows the regex expressions for the same:
Syntax | Description |
---|---|
\s+ | For all the spaces |
^\s+ | For the leading spaces |
\s+$ | For the trailing spaces |
Signature: replaceAll() method is defined as:
Syntax: replaceAll() method is written as
Parameters: replaceAll method takes two parameters:
- String regex: The regular expression that is used for matching the substring.
- String replacement: The string that replaces the matched substring.
Return Values: It returns the modified string, where all the occurrences of matched substrings are replaced by the string specified as a parameter. Exceptions: It throws :
- PatternSyntaxException : If the syntax of the regex is invalid.
Output:
Explanation: So in the above program, the syntax defined for regex is incorrect; hence exception is thrown.
Program to Remove All Whitespaces and Leading, Trailing Whitespaces
Output
Explanation In the above program, replaceAll() is called with different regular expressions as parameters to remove leading, trailing, and all the spaces.
Note: We can not directly use "" in Java, escape character is required. Thus, "\" is used instead.
Conclusion
- Java has inbuilt methods to remove the whitespaces from the string, whether leading, trailing, both, or all.
- trim() method removes the leading and trailing spaces present in the string.
- strip() method removes the leading and trailing spaces present in the string. Also, it is Uniset/Unicode character aware.
- stripleading() method removes the spaces present at the beginning of the string.
- striptrailing() method removes the spaces present at the end of the string.
- replaceall(String regex, String replacement) method replaces all the matched substring with a new string.