What is the Rest Operator in Javascript?
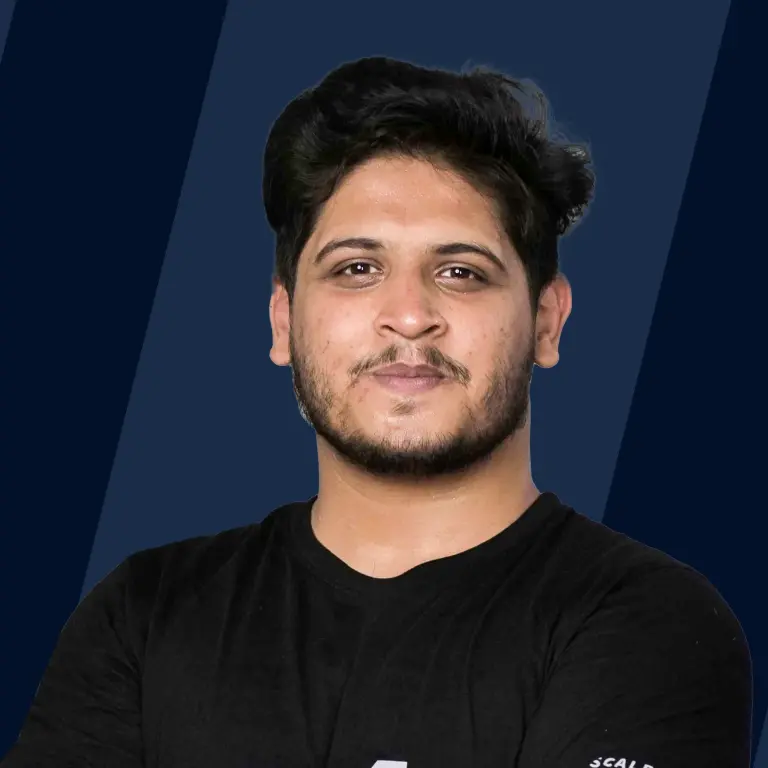
The rest operator in javaScript allows a function to take an indefinite number of arguments and bundle them in an array, thus allowing us to write functions that can accept a variable number of arguments, irrespective of the number of parameters defined. These types of functions in JavaScript are called variadic functions (i.e functions of indefinite arity). The rest operator in javaScript was introduced in ES6 (or ES2015) to handle function parameters efficiently and easily.
The rest parameters hold all those leftover arguments that are not given a separate parameter name (i.e formally defined in function expression) By using the rest operator user can call a function with any number of arguments, no matter how it was defined.
The rest parameter always returns an array so we can use any array methods that the javascript provides us on it.
Syntax
The rest operator is denoted by three dots (...) as a prefix followed by the name of the parameter. Let us see the syntax of the rest operator in javaScript to understand it in a better way:
Here, a and b are normal parameters which will map to first and second argument respectively. Whereas myRest is the rest parameter, that will contain contains the third, fourth, and fifth ... nth arguments i.e all the remaining arguments other than the values of a and b.
The rest operator is always given as the last parameter and a function definition can have only one rest operator else the code throws a SyntaxError error in both cases.
Uncaught SyntaxError: Rest parameter must be the last formal parameter
Difference Between Rest Parameters and the Arguments Object
The arguments object is not a real array but, an array-like object (i.e. it does not have any array properties except length) that contains the arguments in the form of an array that are passed to a function.
Following are some differences between the rest parameters in JavaScipt and the arguments object:
- The arguments object is not an actual array, but an array-like object whereas the rest parameters are instances of the array.
- Array methods like push, pop, forEach, etc can be applied to rest parameters directly as arguments are stored in the rest parameter in an array but it throws an error in the case of the arguments object as the arguments object is an array-like object, not an actual array thus, we cannot directly use array properties on it (except length).
- The rest parameter includes only the parameters which are not given a separate name and bundles them into an array, while the arguments object includes all the arguments which are passed to a function and bundled in an array-like object.
- The argument object has an extra property precise to itself i.e. callee property.
Callee property: This property refers to the function which is currently executing. This is useful in functions with unknown names or no names (i.e. anonymous functions) and recursive functions.
Need of Rest Operator in Javascript
The code below, will not throw any error even though we are passing arguments more than the defined parameters, but it will only evaluate the first two arguments although five arguments have been passed.
Output:
However, what if we want to evaluate all the arguments? This is possible by using the rest operator in JavaScript. Rest parameter can bundles n number of arguments together in an array and allows us to do whatever we want with them.
Let us see the same example above with the rest parameter.
Output:
In the above example, we are getting the sum of all the arguments (irrespective of the number of parameters defined) using the rest parameter which bundles all the arguments into an array and then uses for..of the loop to iterate over each element of the array.
From Arguments to an Array
Rest parameters allow us to change the arguments into an array without writing any extra code for converting a set of arguments into an array as we did earlier (before the introduction of the rest operator in JavaScript i.e. before ES6).
In the above code, we first changed the arguments object to an array using some properties and stored it in a variable, changedToArray, and then used the array shift() property on it which worked properly. But when we used the shift() property directly on arguments, we got an error.
Whereas now, after the introduction of the rest operator in JavaScript we can easily access the array without writing such long code for first converting into an array as we did above.
Output:
Arrow Functions in JavaScript Rest Parameters and Arrow Function
Arrow functions in JavaScript do not have arguments object. So we must use the rest of parameters to pass arguments to an arrow function. Let us see the example below:
Output:
Examples
Let us see some examples to understand more about rest operators in Javascript.
Basic Example using Rest Parameters
In the example below, one is mapped to the first argument i.e HTML, two is mapped to the second argument i.e CSS and manyMoreArgs forms an array that contains all the remaining arguments i.e JavaScript, jQuery, Bootstrap, and ReactJS in this example.
Output:
Here, the first and second arguments i.e HTML and CSS are called named arguments.
Calling the above Function with Three Arguments
Output:
Even though there is just one value in manyMoreArgs, it still got stored in an array.
Calling the Above Function with Two Arguments
Output:
Although in the above example the third argument is not provided, still manyMoreArgs is an array, but it is an empty array in this case.
From the examples above we can conclude that the rest parameter in JavaScript always forms an array no matter how many elements (0,1,2 ..... n) are there in the array.
Using the Length Property of an Array
As the rest parameter always creates an array of arguments, so the length property can be used to count the elements of the array.
Output:
Using some more properties of an array:
Output:
In the example above, the rest parameter is passed as the third parameter and we called the function with five arguments. The first two arguments are treated normally and the rest parameter bundles all the remaining arguments in an array. Thus we can apply all methods/properties associated with an array. Since the rest parameter is the third parameter so array elements start from JavaScript till ReactJS, hence when we tried to access the index of JavaScript, we got 0.
Using Rest Parameters in Combination with Ordinary Parameters
In the example below, each of the array elements of the rest parameter (myArgs) is mapped one by one and added to the first parameter i.e numOne and the array is returned.
Output:
Evaluating Specific Arguments
Sometimes, it is possible that you might pass various arguments (of the same or different data types)in a function but want to evaluate some specific arguments based on various conditions. Below is an example of a multiplication() function with different data types as an argument, but we will only evaluate arguments having data type as number.
The function above will find all the arguments with data type number and then multiply them.
Output:
Whereas, the arguments object is not the instance of the array so we cannot use the filter() method directly. Instead, we have to first convert it into an array like this:
Output:
Dynamic Function with JavaScript Rest Parameter
JavaScript allows us to create dynamic functions in JavaScript using function constructor. To create new functions, function object can also be used as a constructor function.
For creating a function using a function object, the syntax is as follows:
Here, argOne, argTwo, .... argN are the function arguments and functionBody is the string which holds all the functions statements which will be executed when we call the function.
We can also use the rest parameter in a dynamic function of JavaScript. Let us see an example using the rest operator to understand better.
Output:
Note: We should avoid using dynamic function as it makes the open to performance and security issues.
Browser Compatibility
The rest parameter in JavaScript is supported by the following browsers:
Learn about JavaScript Arithmetic Operators
JavaScript Arithmetic Operators use two operands and an operator between them in order to calculate the result. JavaScript Arithmetic Operators use a specific symbol in order to be used within the program. You can read more about JavaScript Arithmetic Operators here.
Conclusion
- In JavaScript the three dots (...) are known as the rest operator.
- Rest Operator in JavaScript was introduced in ES6.
- The last parameter in a JavaScript function prefixed by ... is known as the rest parameter.
- A rest parameter allows us to bundle an indefinite number of arguments into an array.
- We can apply various array methods on the rest parameter since it is an array.