Reverse a String in C
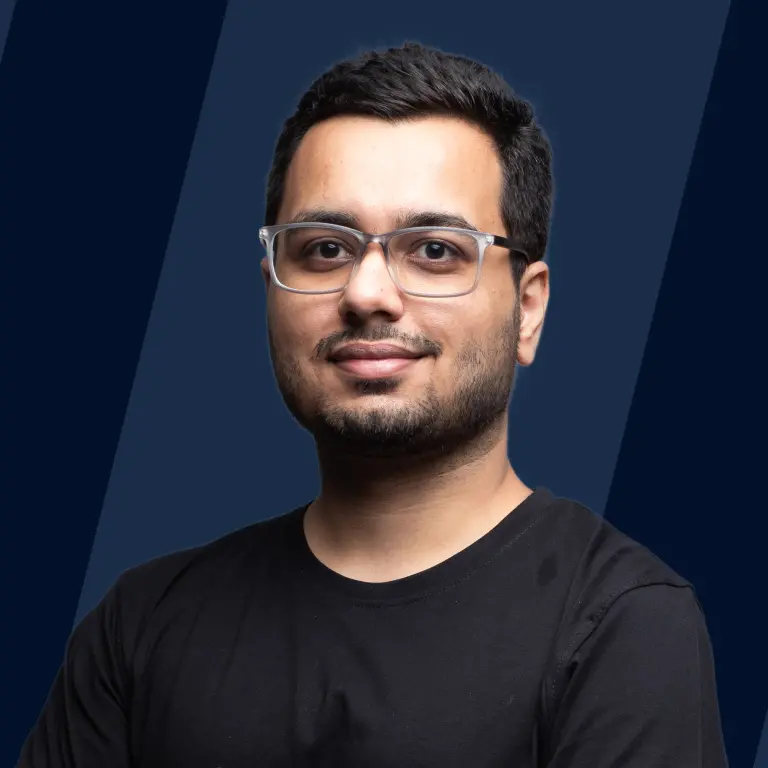
Reversing string in C is like solving a fun puzzle. Imagine you have a word written on a series of flip cards, and your task is to flip them around so the word reads backwards. In C programming, this isn't just a neat trick; it's a fundamental skill that helps programmers understand arrays and pointers better. By learning how to reverse a string, you're also getting a closer look at how data is stored and manipulated in C, laying the groundwork for more complex programming challenges.
How to Reverse a String in C?
There are various ways to reverse string in C. In this shall, we shall mainly be covering the following ways:
-
Reverse a string using the strrev() function
-
Reverse a string without using the library function
-
Reverse a string using the recursion function
-
Reverse a string using for loop
-
Reverse a string using a while loop
-
Reverse a string using pointers
Let us look at these ones by one.
Reverse a String Using the strrev() Function
strrev() is an in built function defined in the string.hheader file.
Syntax: Syntax of the strrev() function or in other words, it is written as:
Parameters: It takes a single parameter.
-
str: String that is to be reversed
Return Value: It returns the modified version of the reversed string.
Let us look at an example.
Output:
In the above program, strrev( ) is called on the given string, and reversed string is obtained. Notice that strrev() is not standard function so it may not work in modern compilers. One can write user defined strrev() function.
Reverse a String Without Using the Library Function
In the above section, we used an in-built function to reverse the string in C. Let us define our function to do the same. In this case, we shall first calculate the length of the string first, and run a for loop to assign characters of another string equal to the characters of the original string in reverse order.
Let us see how:
Output:
In the above program, we calculated the length of the original string and declared an empty string. Further, all the character values of the original string were assigned to that of the new string in reverse order. The iteration was done till the calculated length.
Reverse a String Using For Loop
In this section, we shall use a for loop to reverse a string in C. A basic swapping technique will be used, where we swap the string's first character by value with the last and so on. This swapping is implemented inside the for loop.
Let us see how:
Output:
In the above program, we first calculated the length of the string and iterated over the length to swap the characters. Finally, the reversed string is printed.
Reverse a String Using While Loop
This way is similar to the one where we used the for loop. The only difference is that it is replaced while the rest of the code remains the same.
Let us look at the implementation:
Output:
Reverse a String Using the Recursion Function
To make the code more efficient, we shall use the recursion to replace the basic iteration. The swapping technique remains the same. We shall call the function containing logic recursively, and character by character swapping shall occur.
Let us look at the implementation:
Output:
In the above program, the reverse function is called recursively. The characters are swapped to get the reverse string.
Reverse a String Using Pointers
Let us implement the string reverse technique using two pointers, start and end. Start points to the beginning of the string, whereas the end to the last character of the string. Character by character swapping occurs, and the pointers are incremented or decremented.
Let's see the code:
Output:
In the above program, we declared two pointers, start and end. The swapping is achieved with the help of these pointers, start keeps incrementing, whereas end keeps decrementing during the iteration over the length of the string.
Conclusion
- We explored various techniques for string reverse in c, including library functions, loops, recursion, and pointer manipulation, showcasing the flexibility of C programming.
- Through these methods, we delved deeper into C's core concepts like arrays, pointers, and recursion, enhancing our understanding of how data is managed and manipulated.
- String reversal might seem basic, but it lays the groundwork for tackling more complex problems in programming, from data processing to algorithm development.
- Each method of reversing a string in C offers a unique problem-solving approach, honing our analytical and logical thinking abilities.