How to Reverse a Vector in C++?
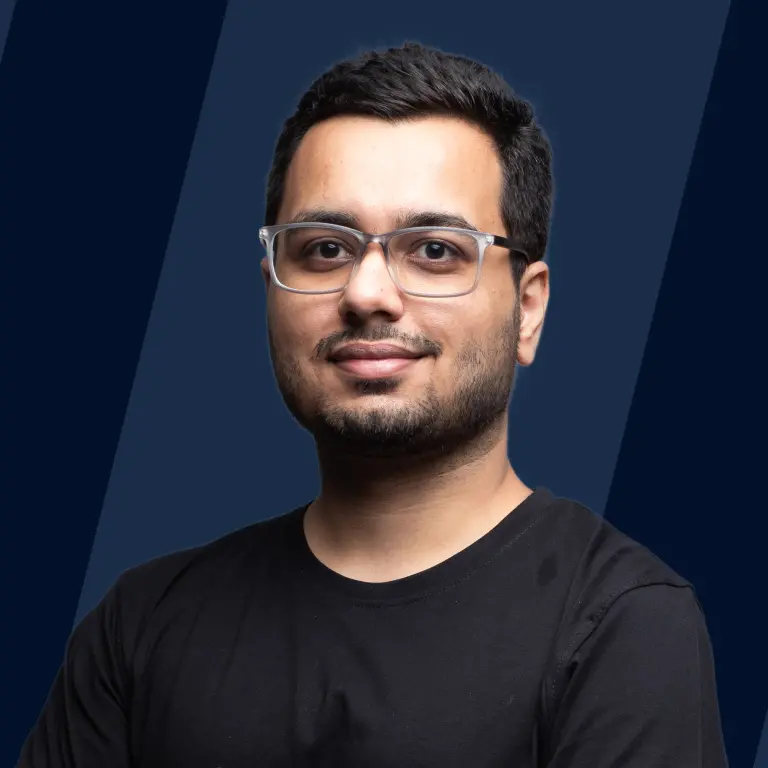
Overview
Vectors are essential in programming for dynamic storage. Reversing vector elements is a key operation with wide-ranging applications. Whether it's rearranging data or altering processing order, mastering vector manipulation is crucial. The uncomplicated std::reverse function from <algorithm> enables easy reversal, simplifying tasks like palindrome checks or output formatting. Understanding and implementing vector operations like reversal expands your C++ toolkit significantly. There are numerous ways to do it like: using std::reverse() function in STL, using swapping of elements, using reverse iterators, using std::transform() function, etc.
Reverse a Vector in C++ Using std::reverse() Function in STL
Reversing the order of elements in a vector is a common task in programming. C++ offers a convenient way to achieve this using the std::reverse() function from the Standard Template Library (STL). This function streamlines the process, saving both time and effort. In this section, we'll delve into how to use std::reverse() to reverse a vector, examine the associated code, observe sample outputs, and touch on the computational complexity involved.
Explanation
The std::reverse() function resides in the <algorithm> header of the C++ STL. It takes two iterators pointing to the beginning and end of the range to be reversed. When called, it swaps the elements so that the last element becomes the first, the second-to-last becomes the second, and so on, until the first element ends up as the last.
Code Example:
Output:
The above code will produce the output:
Complexity
std::reverse() has a linear time complexity of , where N is the number of entries in the range. This is because each element must be switched only once. The space complexity is constant because no additional memory is required, .
Important Points
-
std::reverse() Function:
The std::reverse() function, part of the <algorithm> library, offers a straightforward method to reverse a vector's elements. By providing the beginning and ending iterators of the vector, it efficiently swaps the elements in-place, resulting in a reversed vector.
-
Element Swapping Method:
A manual approach to reversing a vector involves swapping elements symmetrically from both ends. Starting with the first and last elements, iteratively swapping and progressing inwards results in a reversed vector.
-
Reverse Iterators Method:
Utilizing reverse iterators, which move in the opposite direction compared to regular iterators, offers a convenient way to traverse the vector in reverse order. This allows straightforward element access for reversal without the need for explicit index manipulation.
-
std::transform() Function:
The std::transform() function, another utility from the <algorithm> library, can be used to reverse a vector's elements by applying a transformation operation. By specifying the range of elements using iterators and providing a suitable transformation function, this function effectively reverses the elements in the vector.
Reverse a Vector in C++ Using by Swapping the Elements
Explanation
To reverse a vector, iterate halfway through it and swap the matching front and rear components. This procedure is repeated until the halfway is achieved. Swapping items reverses the vector's order. This approach is memory-efficient and does not necessitate the use of extra RAM.
Code Example
Output:
Running the provided code will yield the following output:
Complexity
This reverse method has a temporal complexity of , which reduces to , where N is the number of items in the vector. The space complexity is , independent of vector size, and takes very little extra memory As a result, element swapping enables a simple approach to reverse a vector's element order in C++.
Reverse a Vector in C++ Using Reverse Iterators
Explanation
In C++, reverse iterators are utilized with the methods rbegin() and rend(). These iterators traverse the vector in reverse, enabling us to modify items easily. The elements are swapped starting from the front and rear and progressing towards the center. As a result, the vector is inverted.
Code Example
Complexity
The temporal complexity of reversing a vector with reverse iterators is , where N is the vector's element count. This is because each piece must be visited and switched only once. Because no additional space is required aside from the input vector, the space complexity remains .
Reverse a Vector in C++ Using std::transform() Function
When working with data, reversing the order of items in a C++ vector is a typical procedure. The standard library provides efficient tools, including the std::transform() function. This section will reverse a vector using std::transform(). We'll review the explanation, present a code sample, demonstrate the results, and analyze the approach's complexity.
Explanation
The std::transform() function is found in the <algorithm> header of the C++ Standard Library. It makes element-wise conversions between two input ranges easier. We may reverse the order of a vector by employing it wisely. This entails defining the source and destination vectors and a transformation function that assigns values in reverse order.
Code Example
Output:
Complexity
This method has a linear time complexity, , where N is the size of the vector. Each element is processed precisely once by the std::transform() method. The space complexity is also since the reversed items require storing an extra vector.
In the context of the provided code and the method used, the best and worst-case complexities for reversing a vector using std::transform() are as follows:
-
Best Case Complexity:
The best-case scenario occurs when the vector has only a few elements or even when it's empty. In this case, the time complexity is still , where N is the size of the vector. This is because even though the number of operations might be relatively small, the linear time complexity is inherent to the algorithm.
-
Worst Case Complexity:
The worst-case scenario happens when the vector contains a large number of elements. Again, the time complexity remains . The std::transform() function and the lambda function it uses both have constant time complexity for each element and since there are N elements in the vector, the total time complexity remains linear.
The C++ method std::transform() provides an elegant and efficient mechanism to reverse the order of elements within a vector. This method eliminates the need for manual looping and improves code readability. Understanding how to use the function and its code implementation, output, and complexity provides developers with a strong vector manipulation tool.
Conclusion
- Vectors are resizable dynamic arrays that provide flexibility in handling data collections.
- Begin by adding the necessary header, vector, which provides access to the vector container class in C++.
- Reversing the order of items in a vector is a helpful operation in various situations. In C++, you may reverse a vector using one of many approaches.
- The std::reverse() function in C++ simplifies vector reversal by handling all the sophisticated swapping logic behind the scenes.
- The C++ function std::transform() provides an elegant and efficient way to reverse the order of elements within a vector. This method eliminates the need for manual looping and improves code readability.
- Using reverse to reverse a vector in C++ iterators is a simple and efficient approach, accomplished with the help of rbegin() and rend() functions.