Reverse Words in a String in Data Structures
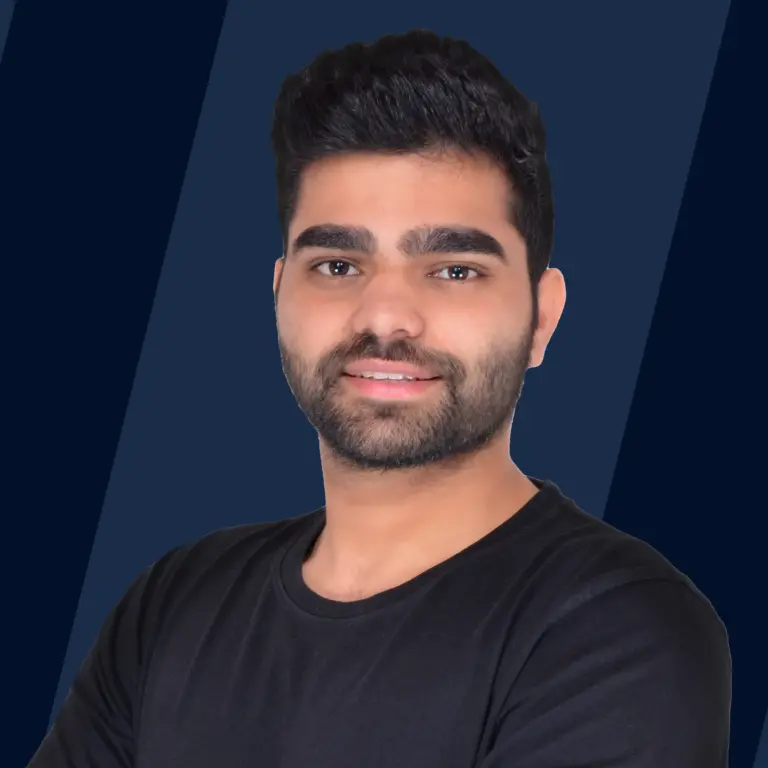
Overview
In this problem "Reverse words in a String", we are given an input string s. We need to reverse the order of the words and return a string of the words in reverse order.
Takeaways
In this article we will learn how to reverse words in a string using different approach.
Example
Input :
Output :
Example Explanation
The string given to us is "welcome to scaler topics". To reverse the words of this string, we need to think of a logic to reverse the words and also to remove any leading or trailing spaces. This gives us "topics scaler to welcome".
Constraints
- Length of the string s will be between 1 and 104.
- s could contain English letters (upper-case and lower-case), digits, and spaces ' '.
- There is at least one word in s.
Approach 1 : Splitting and Saving the String in a Reverse Manner
One of the easiest ways to reverse words in a string is by splitting the string and then saving it in reverse order. This is the naive approach. We will now look at the code for this method :
Java Implementation
In Java, we have the split method, which helps us split the string. We use it to split the string using the delimiter and reverse and store the line in another variable.
Output :
Python Implementation
In python too, we have the split method, which helps us split the string. We use it to split the string using the delimiter and reverse and store the line in another variable.
Output :
C++ Implementation
Output :
Complexity Analysis
Let's analyze the time and space complexity of this approach :
Time Complexity : as we use only one for a loop.
Space Complexity : as we are storing our output that is, the reversed string in a new variable.
Approach 2 : Using the Two-Pointers Approach
Now, here comes the optimal solution for this problem.
We initialize two pointers, one at the start of the string and another at the end. Then, we swap the words from the start and end, to achieve the reversed string.
Algorithm :
- Turn the string s into an array of strings in which the words of s are stored.
- Now, initialise the left and right pointers at 0 and – .
- Now, we need to swap the elements at the left and right pointers. For that, move the left pointer ahead and the right pointer backward by one position and swap the elements at each position while the left pointer does not exceed the right pointer.
- Return the final string.
Java Implementation
Output :
Python Implementation
Output :
C++ Implementation
Output :
Complexity Analysis
Let's analyze the time and space complexity of this approach :
Time Complexity :
Space Complexity :
Conclusion
- Reverse words in a string is a really important problem regarding interview preparation.
- One of the easiest ways to solve this problem is by splitting the string and then saving it in reverse order.
- Another way is to swap the words from the start and end to achieve the reversed string. This is also the optimal solution to the problem.