Right Shift Operator in Java
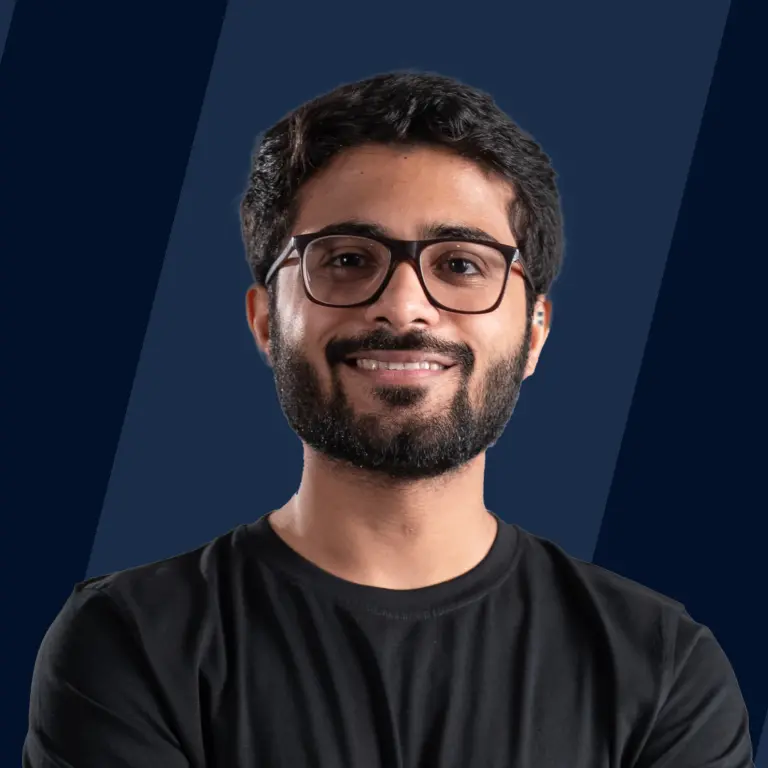
Overview
The right shift operator in Java moves the bits of a value towards the right by the specified number of bits. The right shift operator has two types: signed right shift (>>) and unsigned right shift (>>>) operator.
How Right Shift Operator Works in Java?
Consider having a sequence of bits representing a value in the binary format. The right shift (aka) signed right shift operator shifts the bits towards the right by the specified number of times. It is denoted by the symbol >>.
When a bit is shifted towards the right, the rightmost bit (least significant bit) is discarded and the leftmost bit (most significant) bit is filled with the sign bit (i.e.) when the number is positive, 0 is used to fill the leftmost position, and when the number is negative, the sign bit is used to fill the leftmost position.
In the above example, we shifted a bit towards the right in the binary value of 8. When we shift a bit towards the right, the rightmost bit (least significant bit) is discarded, and the leftmost bit (most significant bit) is filled with a sign bit (0 because 8 is a positive number). So the output became 0100, the binary value of 4.
Syntax
The syntax of the signed right shift operator in Java is:
- operand - The values whose bits will be shifted towards the right
- number - The number of bits to be shifted
What is an Unsigned Right Shift Operator in Java?
The unsigned right shift operator in Java moves the bits towards the right by the specified number of times. It is denoted by the symbol >>>. It behaves the same way as the signed right shift operator. The only difference is the left most bit (most significant bit) is filled with 0 irrespective of the sign of the value (+ve/-ve). The rightmost bit (the least significant bit) is discarded.
Syntax
The syntax of the unsigned right shift operator in Java is:
- operand - The values whose bits will be shifted towards the right
- number - The number of bits to be shifted
Examples of Right Shift Operators in Java
Example 1: Signed Right Shift Operator for a Positive Number
Let's take a positive number (8) and shift its bits twice (8 >> 2) using the signed right shift operator.
Solution
The binary value of 8 is 1000, and we need to shift its bits twice towards the right.
Output
Explanation
The below image illustrates the step-by-step shifting for 8 >> 2
- In the first shift, the rightmost bit (0) is discarded, the leftmost bit is filled with 0, and the value becomes 0100.
- In the second shift, the rightmost bit (0) is discarded, and the leftmost bit is filled with 0, and the value became 0010, and the result of 8 >> 2 is 2
Shifting a bit towards the right equals dividing the number by two. For example, 8 >> 1 is equal to 8 / 2. In this example, we shifted two bits towards the right, equivalent to dividing the number by 4. So (8 >> 2) = (8 / 4) = 2
Example 2: Signed Right Shift Operator for a Negative Number
Let's take a positive number (-8) and shift its bits twice (-8 >> 2) using the signed right shift operator.
Solution
-8 is a negative number. So, we need to use 2’s complement system to represent it in binary form. (Note that 1's complement is calculated by inverting all the bits of the number, and 2's complement is calculated by adding 1 to the 1's complement)
The binary representation of -8 is 11111000, where the first four bits (1111) represent the sign bits.
Output
Explanation
- In the first shift, the rightmost bit (0) is discarded, the leftmost bit is filled with 1, and the value becomes 11111100.
- In the second shift, the rightmost bit (0) is discarded, the leftmost bit is filled with 1, and the value becomes 11111110.
- The value 11111110 is the 2's complement form, and we need to convert it back into the actual binary form.
Output
Example 3: Unsigned Right Shift Operator
Shift the bits twice for the numbers 8 and -8 using the unsigned right shift operator.
Solution
The binary values of 8 and -8 are 00001000 and 11111000, and we need to shift their bits twice towards the right.
Output
Explanation
- The unsigned right shift operator gives the same result as the signed right shift operator for positive numbers. So, (8 >> 2) = (8 >>> 2) = 2.
- But the unsigned right shift operator doesn't give the same result as the signed right shift operator for negative numbers because for the signed right shift, the leftmost bit is filled with the sign bit, and for the unsigned right shift, it is filled with 0. So the result is a positive number (1073741822)
Learn More About Java Programming
Please visit Scaler Topic's Java hub to learn more about Java programming.
Conclusion
- The right shift operator in Java moves the bits of a value towards the right by the specified number of bits.
- In the signed right shift, the rightmost bit is discarded, and the leftmost bit is filled with the sign bit.
- In unsigned right shift, the rightmost bit is discarded, and the leftmost bit is filled with 0.
- The signed right bit operator and the unsigned right bit operator produce the same result for positive numbers but different results for negative numbers.