Round Function in Python
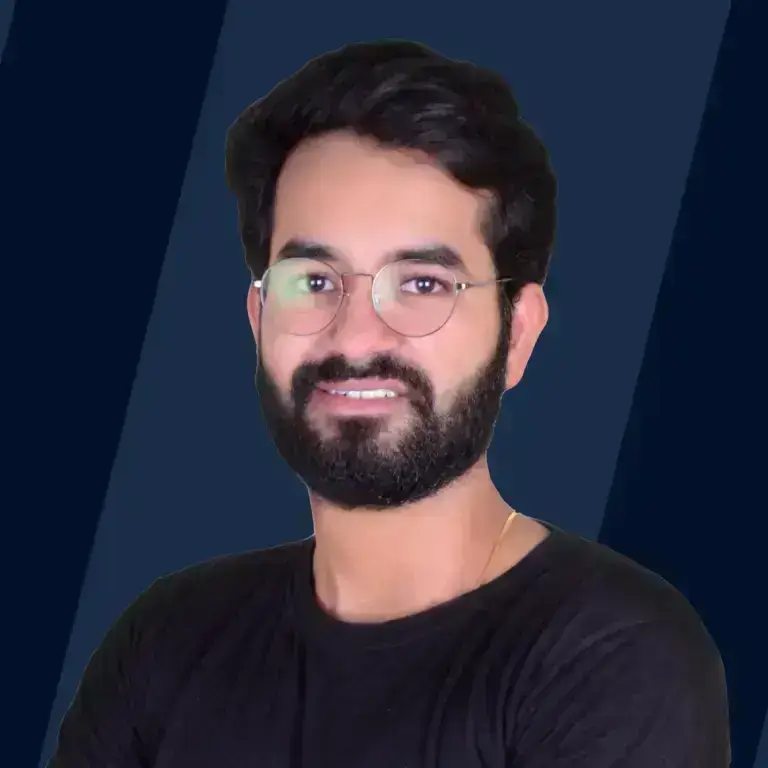
Overview
Round function in Python is an inbuilt function. It is used to round off numbers up to the specified number of decimal points. It is an important inbuilt function.
Introduction to Round Function in Python
Suppose you go to a store and buy multiple items and the bill comes out to be of an amount with 2 decimal places. Ever wondered how rounding off is done for such a bill amount? The answer is the round function. Read along to know more.
The round function in Python is used for rounding off numbers up to a specified number of digits after the decimal point. For example, 65.234 can be rounded off to 65.2. It is an inbuilt function in Python and hence does not require any module to be imported for its usage.
Syntax of Round Function in Python
The round function has the following syntax:
round(number, n_digits)
- where number is the number to be rounded off
- n_digits is the number of digits after the decimal point to be rounded off. The n_digits parameter is optional and can also be omitted.
Python round() function Parameters
The round function accepts two parameters:
1. Number: This is the number that is to be rounded off. It can be a number of any type like floating point, integer, etc. This a required parameter for the round function and omitting this parameter gives an error. If any unsupported data type like string is passed to this parameter, it gives an error like str doesn't define round.
2. n_digits: This is the number of digits after the decimal point to be retained after rounding off the number. This is an optional parameter and can be omitted. Omitting it does not give any error and the code returns a value without any decimal points. The default value of this parameter is zero. This parameter can have any integer value(positive, negative, or zero).
Python Round() Function Return value
Round function's return value depends upon the value of n_digits. The round() function always returns a number that is either a float or an integer.
According to the official Python documentation, it returns a value rounded to the closest multiple of 10 to the power minus n_digits(10^-n_digits)
If the input number is an integer, the return value is also an integer.
If the input number is a floating-point number, the output depends on n_digits. If n_digits is not specified, an integer is returned, a floating-point number is returned.
Practical applications
- Rounding off bill amounts - A bill amount is generally paid in integer values, so the round() function can be used to round off the bill amount.
- Rounding off scientific data - Scientific data is high-precision data and thus needs to be in a required format with a fixed number of floating and integer digits. This precision can be achieved with the help of the round() function.
- Handling Engineering Data - Theoretical data in engineering may need adjustments to be used feasibly in real-world applications. The round() function can help in such cases to round off the values for applications.
Examples of Round off in Python
Example 1
Output
Example 2
Output
Python Round() Function Example With Float Numbers
Floating point numbers are rounded on the basis of the n_digits parameter.
Example
Output
Python Round() Function Example With Integer Values
Integer values are not much affected by the round function. Integers are affected only if the value of the n_digits parameter is less than zero. In such cases, the integer number gets less significant. For example, if 652 is rounded off to -1 decimal points, it would become 650.
Let's have a look at some more examples with the help of the code below:
- Round off in python with a positive value of n_digits has no effect on the integer number.
Output
- Round off in python with zero value of n_digits also has no effect on the integer number.
Output
- Round off in python with a negative value of n_digits makes the number less significant. If the value of n_digits is -a, it makes the last a digits of the number equal to zero while rounding off the other digits.
Output
Tie Breaking in Python Round() Function
In this section, we will discuss how the python round() function will break ties. A tie occurs when the last digit of a number is 5 and the last needs to be removed while rounding.
The above technique can be explained by the examples below:
- Rounding off integers in Python
Output
- The same technique is also applied when floating-point numbers are round off in python.
Output
Python Round() Function Example With Negative Numbers
Rounding off a number to a negative number of decimal points is valid in python and it rounds off the right most digits of the number. Using negative numbers as n_digits parameter reduces the significance of the input number.
This is explained further by the examples below:
- Rounding off to negative decimal points
Output
- If the absolute value of negative decimal points is greater than the number of digits in the original number.
Output
Python Round() Function Example With Numpy Arrays
The round() function can also be used on numpy arrays in a similar way as it is used on numbers. We need to import the numpy module and use the function as numpy.round(input_array) instead of round(). This feature will round off all the values of the numpy array to given precision with a single line of code. The below examples will draw a clearer picture:
Example 1
Output
Example 2
Output
Python Round() Function Example With Decimal Module
The decimal module can be used with the round function to handle decimal numbers more accurately. While using the decimal module we specify a rounding parameter to a flag to specify the type of rounding to be applied. The flags are a set of predefined rules as explained below.
DECIMAL MODULE has the following flags available:
- ROUND_CEILING - It can be specified using rounding=decimal.ROUND_CEILING. This flag rounds up a number towards infinity. It means that it will always round off the value to the next higher permissible value.
Output
- ROUND_DOWN - It can be specified using rounding=decimal.ROUND_DOWN. This flag rounds up a number towards zero. It means that it will always round off a positive value to the next lower permissible value but a negative value to the next higher permissible value.
Output
- ROUND_FLOOR- It can be specified using rounding=decimal.ROUND_FLOOR. This function rounds down a number towards negative infinity. This means that it will always round a number to the next lower permissible value. The difference between ROUND_FLOOR and ROUND_DOWN is in the case of negative numbers. ROUND_DOWN rounds up a negative value to the next higher permissible value whereas ROUND_FLOOR rounds down a negative number to the next lower permissible value.
Output
- ROUND_HALF_DOWN - It can be specified using rounding=decimal.ROUND_HALF_DOWN. This function rounds down a number towards the nearest permissible number and if a tie occurs, it will be rounded towards zero.
Output
- ROUND_HALF_EVEN - It can be specified using rounding=decimal.ROUND_HALF_EVEN. This function rounds down a number towards the nearest permissible number and if a tie occurs, it will be rounded towards the nearest even value.
Output
- ROUND_HALF_UP - It can be specified using rounding=decimal.ROUND_HALF_UP. This function rounds down a number towards the nearest permissible number and if a tie occurs, it will be rounded away from zero, which means positive values will be rounded to the next higher value whereas negative values will be rounded down the next lower value.
Output
- ROUND_UP - It can be specified using rounding=decimal.ROUND_UP. This function rounds a number away from zero irrespective of the tie condition. It will round off a positive number similar to the ceil() function. A positive number will be rounded off to the next higher value and a negative value will be rounded off to the next lower value.
Output
How to Round Away from Zero
If a value in the range [-0.5, 0.5] is rounded using the round() function of python, the answer leads to zero as it follows half to even rounding and rounds the number to the nearest integer value, which comes out to be zero for the range mentioned above.
To overcome this problem we can use the following solutions:
- For positive numbers, we can add 0.5 to our number. This ensures that our number is always greater than 0.5 and prevents it from being rounded to zero.
Output
- For negative numbers, we can subtract 0.5 from our number. This ensures that our number is always lesser than -0.5 and prevents it from being rounded to zero.
Output
Usages of Python Round() Function
Python round() function has a lot of usages -
- It can be used wherever a need to round off a number to a fixed number of decimal points arises.
- It can be used to format outputs of programs, for example, if we need only 2 decimal points in all our outputs but due to mathematical calculations we are getting a different number of decimal places for each value, we can use round() function to bring our output to a uniform format.
- It can be used to uniformly round off an array of values using numpy arrays.
- It can also be used to round off numbers to the required integer precision(252 can be rounded to 250)
- It cannot be used to round off a string value or a character array and in such cases, it gives an error.
Conclusion
- In this article, we have discussed the basic working of the round() function in python.
- We have also studied its required parameters and its return type.
- The usage of the round() function with different types of data like integer, float, etc. has also been discussed.
- The usage of the round() function with numpy arrays and with the decimal module is also explained.
- Finally, we have discussed how to round away from zero and also the various usages of the python round() function.