C Program for Round Robin Scheduling
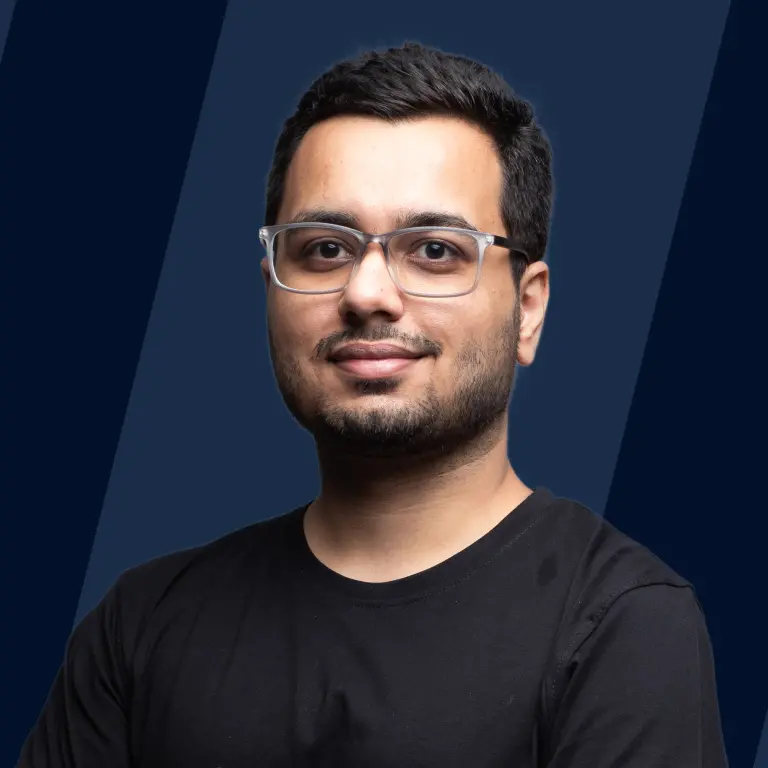
Overview
In our previous articles, we learned about First Come First Serve based CPU scheduling where the CPU scheduling algorithm scheduled the processes on the basis of the arrival time which is the process in which requests for the resources first are allocated likewise. Round Robin Scheduling is generally a primitive version of what we learned in First Come First Serve based CPU scheduling. In Round Robin Scheduling, the processes run for a specific time period(called quantum) and successive processes wait for their turn to execute.
Introduction to Round Robin Scheduling in C
Round Robin Scheduling Algorithm is a scheduling algorithm for time-sharing systems. It is preemptive in nature that it switches between processes according to the time allotted for each process. The time slice that is used to switch between the processes is known as Quantum. Round Robin scheduling is cyclic in nature and is also known as Time Slicing Scheduling.
Algorithm of Round Robin Scheduling in C
- All the processes are organized in the ready queue based on the arrival time. To execute the CPU processes, a queue based on FIFO is used.
- Push the first process from ready queue for the task to be executed for the allotted time.
- The process runs for the allocated time even if the process doesn't reach completion, still it is stopped by the process in queue until the arrival time for the next process is reached.
- In a similar manner, another process is selected by the ready queue to execute its task.
- The above steps are repeated until all processes are finished.
Characteristics of Round Robin Scheduling in C
- Round Robin Scheduling follows a preemptive approach.
- It is used for time-sharing systems.
- It shares equal time intervals for all processes.
- Round Robin Scheduling is a starvation-free algorithm.
- The implementation of Round Robin Scheduling is simple and easy to use.
Working of Round Robin Scheduling Algorithm
Let's consider a simple example following the above algorithm to understand the working of Round Robin Scheduling.
Round Robin CPU Scheduling Example
We will now work on a Round Robin scheduling program in C. Let us consider 4 processes P1, P2, P3, and P4 with CPU time as 3, 5, 2, and 4 with a time quantum of 1 unit.
Process | CPU time |
---|---|
P1 | 3 |
P2 | 5 |
P3 | 2 |
P4 | 4 |
The Gantt chart is prepared as follows:
P1 | P2 | P3 | P4 | P1 | P2 | P3 | P4 | P1 | P2 | P4 | P2 | P4 | P2 |
---|
Time | Process |
---|---|
0-1 | P1 |
1-2 | P2 |
2-3 | P3 |
3-4 | P4 |
4-5 | P1 |
5-6 | P2 |
6-7 | P3 |
7-8 | P4 |
8-9 | P1 |
9-10 | P2 |
10-11 | P4 |
11-12 | P2 |
12-13 | P4 |
13-14 | P2 |
The waiting time for processes are
P1= 0+(4-1)+(8-5)= (0+3+3)=6
P2=1+(5-2)+(9-6)+(11-10)+(12-11)+(13-12)=1+3+3+1+1+1=10
P3=2+(6-3)=2+3=5
P4=3+(7-4)+(10-8)+(12-11)=3+3+2+1=9
The average waiting time= ( 6+10+5+9 )/4= 7.5
Explanation:
The waiting time is calculated as the total waiting time till a process reaches a time of completion. Hence, we calculated the waiting time for all the processes P1, P2, P3 and P4 from its Gantt chart and then found the average waiting time by calculating the mean waiting time for all the processes.
Example of Round Robin Scheduling in C
Output:
Explanation:
Arrival time is the time at which the process arrives in the ready queue. Burst time is the time required by a process for its execution. Waiting time is the total time taken for the complete execution of the process. Turaround time is the total time for which the process exists in the system. Hence, here since the arrival time and burst time for each process are given, we calculate the turnaround time and waiting time. tat=ct- at and wt= tat-bt and accordingly calculate the average waiting time and average turnaround time.
Round Robin CPU Scheduling Example with Sequential Arrival Time
Output
Explanation
Here in the Round Robin Scheduling program in C, we organized all the processes according to their arrival time in the Ready queue. Then we pushed the first process from ready queue to be executed for the first time. The CPU saves the previous state of the process inorder to resume from the point where it was earlier interrupted. In a similar manner, another process is selected from the ready queue for the task to get executed and the same steps are continued till all the processes reach completion.
Program for Round Robin Scheduling with Arrival Time as 0 for All Processes
Algorithm:
- Consider an array rem_bt[] that keeps track of the remaining burst time of processes. rem_bt is initially a copy of bt[], where bt[] stores burst time in an array.
- Consider an array wt[] to store the waiting times of processes. Initialize wt[] = 0.
- Initialize time : t = 0
- Keep traversing all the processes till it doesn't reach completion.
- If rem_bt[i] > quantum t = t + quantum rem_bt[i] -= quantum;
- Else t = t + rem_bt[i]; wt[i] = t – bt[i] rem_bt[i] = 0;
- End
Example
Output
Program for Round Robin Scheduling with Different Arrival Times for All Processes
Output
Explanation:
In the above Round Robin Scheduling program in C, we first took the process that occurred first and start executing the process considering the time quantum. Then we check for any other process request. If there's a process request amidst the time quantum in which another process is being executed then add the new process to the ready queue. Once, the quantum time passes, we check for any other process in the ready queue. If the queue turns empty and the current process remains incomplete, then we add the current process to the ready queue. We then take the first process from the ready queue and execute it. Finally, when the process gets completed and the ready queue is empty, the task is said to be complete. Hence, we find the Average waiting time and Average turnaround time as we did previously.
Advantages and Disadvantages of Round Robin Scheduling in C
Advantages:
- Every process gets an equal share of CPU in Round Robin Scheduling.
- Since, the time slice is allocated in the form of quantum, it facilitates time sharing.
- The processes get a fair chance to reschedule after a particular time slice.
- It is a starvation-free algorithm and has a cyclic nature.
Disadvantages:
- Round Robin Scheduling is often time-consuming if the time slice or time quantum allocated is less.
- It has a higher response time and waiting time.
- There is context switching in Round Robin Scheduling.
- Round Robin Scheduling does not give priority to any specific process.
Applications of Round Robin Scheduling in C
- Round Robin Scheduling is used for processes having the same priorities or being in the same group in the Operating System.
- It is used by the process and network schedulers.
- It is used by time sharing and real-time systems.
Conclusion
- Round Robin Scheduling is a primitive CPU Scheduling Algorithm. In the above article, we did an implementation of the Round Robin scheduling program in C.
- It is used for Time sharing systems.
- The processes are divided into time slices known as quantum.
- Completion Time is the time when processes complete execution.
- Turn Around Time is the difference in time between the completion time (CT) and the arrival time (AT).
- Turn Around Time (TAT) = Completion Time (CT) - Arrival Time (AT)
- Waiting Time= total time between requesting action and acquiring the resource. (TAT-BT)
- Response Time is the time at which the system responds to a process.
- The main disadvantage of the Round Robin Scheduling Algorithm is Context Switching.
- The merit of using the Round Robin Scheduling Algorithm is that it is cyclic and starvation free.