scanf() Function in C
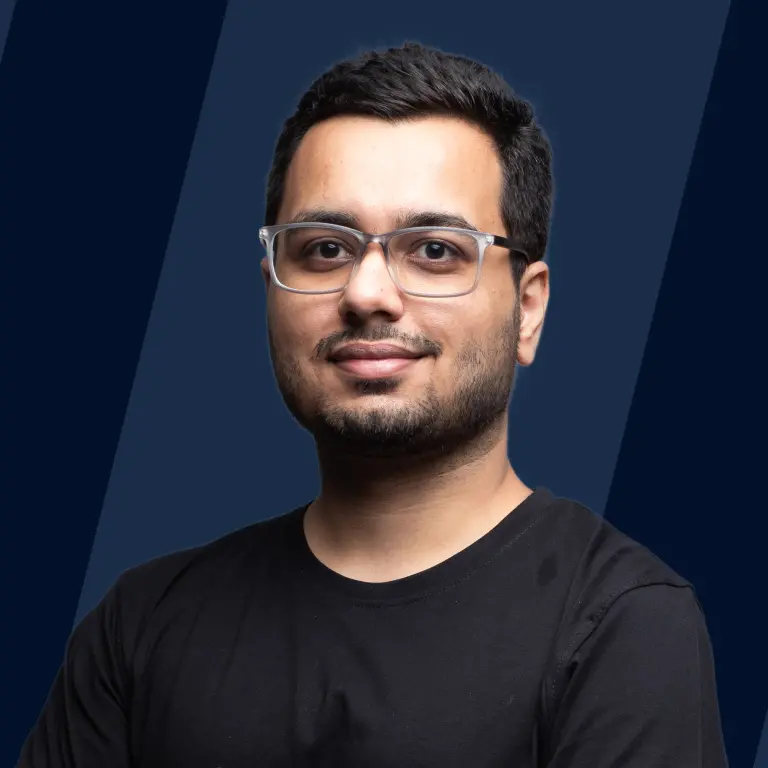
Overview
Scanf in C is defined in stdio.h header file reads formatted data from the standard input stream and writes the information into the memory location of variables passed as arguments in the function call. The scanf function provides the user with an option to provide the program with dynamic values at the time of program execution. The scanf function returns an integer value, indicating the number of items successfully assigned to variables.
Syntax of scanf() Function in C
The scanf in C accepts two values: formatted string and memory location of variables in which we wish to store input data obtained from the user. Let us look at the syntax of the scanf() function mentioned below:
Parameters of scanf() Function in C
The scanf in C reads the input stream and tries to match the input with the format string, and on success, the function writes the information to the memory location passed in the argument. The two parameters passed to the scanf() function are:
- format_string: A character string in C contains the type specifiers. Type specifiers help decide the type of value to be taken from the standard input stream. For example, %c is used for character value, %d is used for integer numbers, etc.
- &variable: The value taken from the input is stored in the location pointed by the variable. This is why we pass the variable address in which we want to store the value.
To understand the role of these two parameters, let's look at the code snippet mentioned below.
Here, we are using a %d format specifier that tells the function to take the integer value from the standard input stream, and &var is the memory address (as mentioned in the image) where we want to store the integer value obtained from the input stream.
The scanf() function accepts the value from the input of the specific type with the help of format specifier and stores this value at the location pointed by the variable passed in the function as an argument.
A simple type specifier has a percentage symbol (%) followed by an alpha character that indicates the data type. The list of valid format specifiers for scanf in C is as follows:
Format specifier | Type of argument | Description |
---|---|---|
d | int * | Accepts an integer value with (optional) + or - sign |
c | char * | Reads a single character value from the input stream |
s | char * | This reads a C string until a white space is read (white space is either a blank space or a newline \n or a tab \t |
o | int * | Reads an octal value |
x,X | int * | Reads an hexadecimal value |
f, g, e, G, E | float * | Accepts a floating point number optionally with a + or - sign and optionally followed with an e or E character. For example, both -3.14 and 3.14e2 are valid entries |
Return Value of scanf() Function in C
The scanf in C returns an integer value. The function returns the following values:
- Greater than zero (>0): This value returns the number of values successfully converted and assigned to the variable location.
- Equal to zero (=0): No items were assigned to variables.
- Less than zero (<0): A error occurred while reading the input or EOF (end-of-file) reached before any assignment to a variable was made. The value of EOF is -1 and is used to indicate the end of the input. Different errors can occur while reading input from the stream, like the keyboard buffer, not being empty at the start, or the format string doesn't match the input stream.
Example
Let us see a simple example that accepts an integer value as an input and displays the value in the stdout console.
Output
Here, we are using "%d" as a format string that reads an integer value from the input stream and stores the read value at the location pointed by the variable number. To obtain the address of the variable number, we are using the & symbol.
What is scanf() Function in C?
In any programming language, we need to take inputs from the user for the computer to interact with the user dynamically. In C, the scanf function is defined in stdio.h header file reads formatted data from the standard input stream (stdin which is generally the keyboard) and writes the information into the memory location of variables passed as arguments in the function call. The scanf function provides the user with an option to provide the program with dynamic values.
More Examples
Let us now see how we can take strings, decimal points, and other data types as input from the user.
Output
In the above example, to read a string, we are using the %s format specifier. The problem with %s is it reads the string until a white space is encountered. If we pass a string with a length longer than the variable space, it will cause an overflow in the provided buffer. So if we pass a string of size longer than 19 characters for the name variable, then the printf function will not be able to find the trailing null character, and it will start reading other parts of the memory and throw garbage value (buffer overrun).
This is why we should use %19s and %29s instead of %s to prevent this vulnerability, as this will cut off the extra characters in the input if the string has a longer length than the buffer.
Conclusion
- The scanf in C reads the formatted data from stdin and writes the data in the memory location provided in the function argument.
- The scanf function in C accepts a format string followed by a list of the memory location of variables where scanf will assign read input data.
- Scanf function returns an integer value, indicating the number of items successfully assigned to variables.